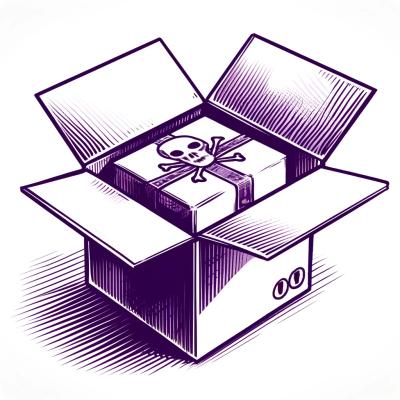
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
yargs-unparser
Advanced tools
The yargs-unparser npm package is used to reverse the process of parsing arguments. It takes an argv object, like the one yargs or minimist would generate, and turns it back into a string of command-line arguments. This can be useful for debugging, logging, or recreating command lines for subprocesses.
Unparsing arguments
This feature allows you to take an argument object and convert it back into an array of command-line arguments.
const yargsUnparser = require('yargs-unparser');
const argv = { _: ['run'], 'file': 'test.js', 'verbose': true };
const unparsedArgs = yargsUnparser(argv);
console.log(unparsedArgs); // Output: ['run', '--file=test.js', '--verbose']
Minimist is a library for parsing command-line arguments. Unlike yargs-unparser, it does not unparse arguments but is commonly used for the initial parsing step that yargs-unparser would reverse.
Commander is a complete solution for node.js command-line interfaces. It is similar to yargs in that it helps with parsing arguments, but it does not have a built-in feature to unparse them like yargs-unparser.
Arg is a simple argument parsing library with a focus on performance. It does not have the unparse functionality but serves a similar initial purpose of parsing command-line arguments.
Converts back a yargs
argv object to its original array form.
Probably the unparser word doesn't even exist, but it sounds nice and goes well with yargs-parser.
The code originally lived in MOXY's GitHub but was later moved here for discoverability.
$ npm install yargs-unparser
const parse = require('yargs-parser');
const unparse = require('yargs-unparser');
const argv = parse(['--no-boolean', '--number', '4', '--string', 'foo'], {
boolean: ['boolean'],
number: ['number'],
string: ['string'],
});
// { boolean: false, number: 4, string: 'foo', _: [] }
const unparsedArgv = unparse(argv);
// ['--no-boolean', '--number', '4', '--string', 'foo'];
The second argument of unparse
accepts an options object:
alias
: The aliases so that duplicate options aren't generateddefault
: The default values so that the options with default values are omittedcommand
: The command first argument so that command names and positional arguments are handled correctlycommand
optionsconst yargs = require('yargs');
const unparse = require('yargs-unparser');
const argv = yargs
.command('my-command <positional>', 'My awesome command', (yargs) =>
yargs
.option('boolean', { type: 'boolean' })
.option('number', { type: 'number' })
.option('string', { type: 'string' })
)
.parse(['my-command', 'hello', '--no-boolean', '--number', '4', '--string', 'foo']);
// { positional: 'hello', boolean: false, number: 4, string: 'foo', _: ['my-command'] }
const unparsedArgv = unparse(argv, {
command: 'my-command <positional>',
});
// ['my-command', 'hello', '--no-boolean', '--number', '4', '--string', 'foo'];
The returned array can be parsed again by yargs-parser
using the default configuration. If you used custom configuration that you want yargs-unparser
to be aware, please fill an issue.
If you coerce
in weird ways, things might not work correctly.
$ npm test
$ npm test -- --watch
during development
Libraries in this ecosystem make a best effort to track Node.js' release schedule. Here's a post on why we think this is important.
FAQs
Converts back a yargs argv object to its original array form
The npm package yargs-unparser receives a total of 5,574,393 weekly downloads. As such, yargs-unparser popularity was classified as popular.
We found that yargs-unparser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.