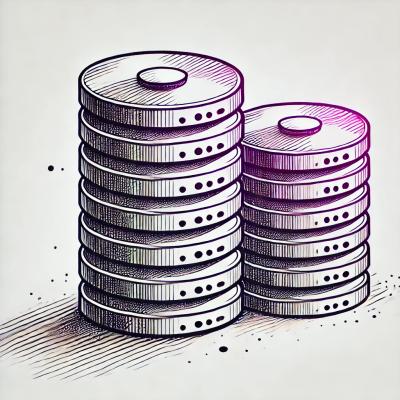
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
zip-stream
Advanced tools
The zip-stream npm package is a streaming zip archive generator. It allows you to compress files and directories on the fly and stream them to the user or save them to disk. It is particularly useful for creating zip files without having to store the entire contents in memory or on disk before sending to the recipient.
Creating a zip archive
This code sample demonstrates how to create a simple zip archive containing a single file with the text 'Hello World!' and the file name 'hello.txt'. The archive is then written to 'path/to/archive.zip'.
const ZipStream = require('zip-stream');
const fs = require('fs');
const archive = new ZipStream();
const output = fs.createWriteStream('path/to/archive.zip');
archive.pipe(output);
archive.entry('Hello World!', { name: 'hello.txt' }, function(err) {
if (err) throw err;
archive.finish();
});
Adding files to a zip archive from a stream
This code sample shows how to add a file to a zip archive from a readable stream. The file 'path/to/file.txt' is streamed and added to the archive under the name 'file.txt'.
const ZipStream = require('zip-stream');
const fs = require('fs');
const archive = new ZipStream();
const output = fs.createWriteStream('path/to/archive.zip');
const fileStream = fs.createReadStream('path/to/file.txt');
archive.pipe(output);
archive.entry(fileStream, { name: 'file.txt' }, function(err) {
if (err) throw err;
archive.finish();
});
Adding multiple files and directories
This code sample illustrates how to add multiple files and directories to a zip archive. It adds 'file1.txt' with direct content, streams 'file2.txt' from the file system, and includes an entire directory 'path/to/directory' under the name 'directory' in the archive.
const ZipStream = require('zip-stream');
const fs = require('fs');
const archive = new ZipStream();
const output = fs.createWriteStream('path/to/archive.zip');
archive.pipe(output);
archive.entry('File content', { name: 'file1.txt' }, function(err) {
if (err) throw err;
archive.entry(fs.createReadStream('path/to/file2.txt'), { name: 'file2.txt' }, function(err) {
if (err) throw err;
archive.directory('path/to/directory', 'directory', function(err) {
if (err) throw err;
archive.finish();
});
});
});
Archiver is a high-level streaming interface for creating archives. It supports multiple archive formats, including ZIP and TAR. It offers more customization options and archive formats compared to zip-stream, which is focused solely on ZIP files.
JSZip is a library for creating, reading, and editing .zip files with JavaScript, with a focus on client-side use. It differs from zip-stream as it is not stream-based and is designed to work with the entire content in memory, which can be less efficient for large files.
Yazl is a minimalistic zip library for Node.js. It is similar to zip-stream in that it focuses on ZIP file creation, but it has a simpler API and fewer features. It may be more suitable for straightforward ZIP file creation without the need for streaming capabilities.
zip-stream is a streaming zip archive generator based on the ZipArchiveOutputStream
prototype found in the compress-commons project.
It was originally created to be a successor to zipstream.
Visit the API documentation for a list of all methods available.
npm install zip-stream --save
You can also use npm install https://github.com/archiverjs/node-zip-stream/archive/master.tar.gz
to test upcoming versions.
This module is meant to be wrapped internally by other modules and therefore lacks any queue management. This means you have to wait until the previous entry has been fully consumed to add another. Nested callbacks should be used to add multiple entries. There are modules like async that ease the so called "callback hell".
If you want a module that handles entry queueing and much more, you should check out archiver which uses this module internally.
import { ZipStream } from "zip-stream":
const archive = new ZipStream(); // OR new ZipStream(options)
archive.on("error", function (err) {
throw err;
});
// pipe archive where you want it (ie fs, http, etc)
// listen to the destination's end, close, or finish event
archive.entry("string contents", { name: "string.txt" }, function (err, entry) {
if (err) throw err;
archive.entry(null, { name: "directory/" }, function (err, entry) {
if (err) throw err;
archive.finish();
});
});
Concept inspired by Antoine van Wel's zipstream module, which is no longer being updated.
FAQs
a streaming zip archive generator.
We found that zip-stream demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.