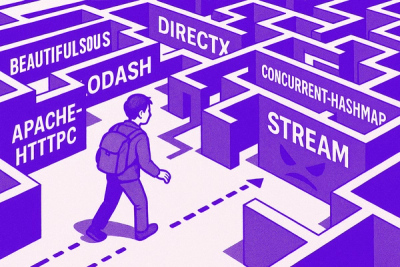
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
This package contains the Gurobi Optimizer v12.0.1. It is compatible with .NET Standard 2.0 or higher.
For more information about Gurobi, please visit our website.
You need a license to solve models with Gurobi. To acquire a license for Gurobi, please visit our License Center.
For an easier start with Gurobi's .NET API, we provide Getting Started with the Gurobi .NET API and multiple .NET code examples showing most of Gurobi's features. You can find all information on methods available in the Gurobi's .NET API in our documentation.
For more information on installing the Gurobi NuGet package, please refer to the Getting Started with Gurobi Optimizer page.
If you encounter any issues during the installation process and could not find an appropriate answer in Gurobi's Knowledge Base, please open a Support Request through our Support Portal.
The following example formulates and solves a simple MIP model via Gurobi's .NET interface.
/*
maximize x + y + 2 z
subject to x + 2 y + 3 z <= 4
x + y >= 1
x, y, z binary
*/
using System;
using Gurobi;
class mip1_cs
{
static void Main()
{
// Create an empty environment, set options and start
GRBEnv env = new GRBEnv(true);
env.Set("LogFile", "mip1.log");
env.Start();
// Create empty model
GRBModel model = new GRBModel(env);
// Create variables
GRBVar x = model.AddVar(0.0, 1.0, 0.0, GRB.BINARY, "x");
GRBVar y = model.AddVar(0.0, 1.0, 0.0, GRB.BINARY, "y");
GRBVar z = model.AddVar(0.0, 1.0, 0.0, GRB.BINARY, "z");
// Set objective: maximize x + y + 2 z
model.SetObjective(x + y + 2 * z, GRB.MAXIMIZE);
// Add constraint: x + 2 y + 3 z <= 4
model.AddConstr(x + 2 * y + 3 * z <= 4.0, "c0");
// Add constraint: x + y >= 1
model.AddConstr(x + y >= 1.0, "c1");
// Optimize model
model.Optimize();
Console.WriteLine(x.VarName + " " + x.X);
Console.WriteLine(y.VarName + " " + y.X);
Console.WriteLine(z.VarName + " " + z.X);
Console.WriteLine("Obj: " + model.ObjVal);
// Dispose of model and env
model.Dispose();
env.Dispose();
}
}
FAQs
The Gurobi Optimizer, including the solver and the .NET API.
We found that gurobi.optimizer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.