-
window()
It create a window for where your output is displayed. Every program must have a window
Parameter of Window
window(width: int, height: int)
Example
from GraphicswithPython import window
window(700,700)
-
color()
Graphicswithpython supports rgb color. So to make this easy just pass a string of color example red
and it will return rgb tuple value
Parameter
color("color") supported color for now are black, grey, red, white, blue, green, yellow, orange, purple
Examples
from GraphicswithPython import color
color("red")
color("blue")
color("black")
color("green")
-
putpixel()
It put specific pixel at given x , y coordinate
Parameter
putpixel(xcordinates: int, ycordinates: int, color: str, intensity: int) # intensity is optional parameter
Examples
from GraphicswithPython import putpixel,window
window(700,700)
putpixel(300,300,"black")
-
getpixel()
It returns RBG color data of specific pixel at provided x ,y co-ordinate
Parament
getpixel(xcordinates: int, ycordinates: int) -> return tuple color example (0,0,255)
Examples
from GraphicswithPython import putpixel,window
window(700,700)
putpixel(300,300,"black")
print(getpixel(300,300))
-
delay()
It delay the programe so you can see the animation
Parament
delay(Milliseconds)
Examples
from GraphicswithPython import delay
delay(1000)
-
circle()
It create a circle of given radius
parameter
circle(xcordinates: int, ycordinates: int, radius: int, color: tuple)
Examples
from graphicswithpython import circle , display , delay , color
display(700, 700 )
circle(300,300,40,color("green"))
delay(5000)
-
rectangle()
It create a Rectangle
parameter
rectangle(left: int, top: int, width: int, height: int, color: tuple)
Examples
from graphicswithpython import rectangle , display , delay , color
display(700, 700 )
rectangle(300,300,100,100,color("green"))
delay(5000)
-
elipse()
It create a Elipse
parameter
elipse(left: int, top: int, width: int, height: int, color: tuple)
Examples
from graphicswithpython import elipse , display , delay , color
display(700, 700 )
ellipse(200,200,100,100,color("white"))
delay(5000)
-
polygon()
It create a Polygon
parameter
polygon(points: tuple, color: tuple)
Examples
from graphicswithpython import polygon , window , delay ,color
window(700,700)
polygon(((300,300),(200,400),(300,500),(500,500),(300,400)),color("white"))
delay(5000)
-
point_in_circle()
It check wheater the point is in circle or not.
parameter
point_in_circle(centerx: int, centery: int, radius: int, x: int, y: int)
Examples
from graphicswithpython import window , delay ,color, pointInCircle ,circle
window(700,700)
circle(300,300,40,color("red"))
print(pointInCircle(300,300,40,500,500))
print(pointInCircle(300,300,40,320,320))
delay(5000)
-
DDA
DDA stands for Digital Differential Analyzer. It is an incremental method of scan conversion of line
Parameter | Explaination |
---|
x1 | Integer varible of x-coordinate |
y1 | Interger variable for y-coordinate of |
x2 | Integer variable for x-coordinate for 2nd point of line |
y2 | Integer variable for y-coordinate for 2nd point of line |
DDA type | Any of dda types required => Line , dash ,solid , dotted . |
color | color() funtion is required to mention the color of dda needed |
Parameter for DDA is
dda(x1: int, y1: int, x2: int, y2: int, DDatype: str, color: tuple
Examples
from graphicswithpython import dda, window, delay, color
window(700, 700)
dda(100, 100, 200, 200, "line", color("blue"))
dda(200, 200, 300, 300, "dash", color("white"))
dda(300, 300,400,400, "solid", color("blue"))
dda(400,400,500,500, "dotted", color("blue"))
delay(5000)
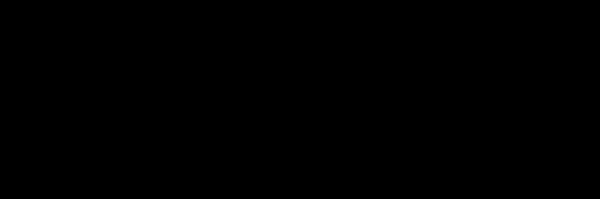
-
Breshham
Bresenham's line algorithm is a line drawing algorithm that determines the points of an n-dimensional raster that should be selected in order to form a close approximation to a straight line between two points.
Required | Explanation |
---|
x1 | x-coordinate for 1st point of line |
y1 | y-coordinate for 1st point of line |
x2 | x-coordinate for 2nd point of line |
y2 | y-coordinate for 2nd point of line |
bresenhamstype | Type of Bresenham needed => line ,dash , solid , dotted |
color | color() funtion is required (rgb tuple ) |
Parameter for Bresenham
bresenham(x1: int, y1: int, x2: int, y2: int, bresenhamstype: str, color: tuple)
Examples
from graphicswithpython import bresenhams, window,delay,color
window(700,700)
bresenhams(200,200,300,300,"line",color("green"))
bresenhams(300,300,400,400,"dash",color("yellow"))
bresenhams(400,400,500,500,"solid",color("red"))
bresenhams(500,500,600,600,"dotted",color("white"))
delay(5000)

-
Midpointcircle
The midpoint circle algorithm is an algorithm used to determine the points needed for rasterizing a circle.
Required | Explaination |
---|
radius | radius of circle |
xcenter | x-coordinate for center of circle |
ycenter | y-coordinate for center of circle |
midpointtype | type of midpoint circle needed => LINE , Dash, solid, Dotted, DashandLine |
color | color() function is required (rgb tuple ) |
Parament for Midpointcircle
midpoint(radius: int, xcenter: int, ycenter: int, midpointtype: str, color: tuple)
Examples
from graphicswithpython import window, delay, color, midpointcircle
window(700,700)
midpointcircle(70,200,200,"line",color("red"))
midpointcircle(70,400,200,"dash",color("green"))
midpointcircle(70,200,400,"dotted",color("white"))
midpointcircle(70,400,400,"solid",color("blue"))
midpointcircle(60,300,300,"dottedandline",color("yellow"))
delay(2000)
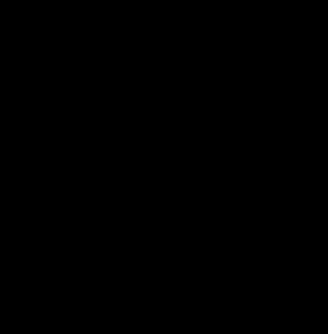
-
FloodFill
Flood fill, also called seed fill, is an algorithm that determines and alters the area connected to a given node in a multi-dimensional array with some matching attribute.
Required | Explaination |
---|
xcenter | x-coordinate of center point |
ycenter | y-coordinate of center point |
backgroundcolor | exisiting backgroundcolor of window |
newcolor | color() funtion required for new color to be filled |
seed | number of seed you need for FloodFill |
radius | radius of object in which doing floodfill. (optional) |
Note : Radius tuple is required for 8 seed floodfill
Parameter of FloodFill
xcenter: int, ycenter: int, backgroundcolor: tuple, newcolor: tuple, seeds: int, radius: int | None = None
Examples (for 4 seed )
from graphicswithpython import floodfill, window,delay,color , circle ,rectangle,polygon
window(700,700)
circle(100,100,40,color("red"))
floodfill(100,100,backgroundcolor=color("black"),newcolor=color("green"),seeds=4)
rectangle(200,300,100,100,color("blue"))
floodfill(210,310,backgroundcolor=color("black"),newcolor=color("green"),seeds=4)
polygon(points=((500,100),(500,200),(600,200),(630,150),(600,100)),color="red")
floodfill(530,150,backgroundcolor=color("black"),newcolor=color("green"),seeds=4)
rectangle(350,550,140,100,color("red"))
floodfill(400,600,backgroundcolor=color("black"),newcolor=color("green"),seeds=4)
delay(5000)
Examples (for 8 seed)
from graphicswithpython import floodfill, window, delay, color, circle, rectangle,triangle
window(700,700)
circle(390,348,50,color("purple"))
triangle(325,410,270,500,380,500,color("yellow"))
rectangle(200,300,100,100,color("red"))
rectangle(250,350,140,100,color("white"))
floodfill(210,310,backgroundcolor=color("black"),newcolor=color("green"),seeds=8)
floodfill(390,348,backgroundcolor=color("black"),newcolor=color("green"),seeds=8,radius=49)
floodfill(315,380,backgroundcolor=color("black"),newcolor=color("green"),seeds=4)
floodfill(325,470,backgroundcolor=color("black"),newcolor=color("green"),seeds=8)
delay(5000)
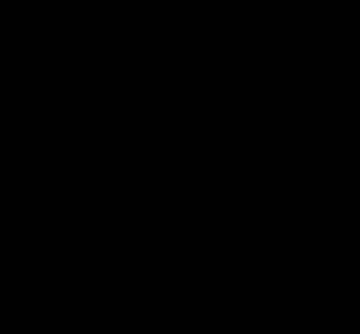
-
Boundary Fill
Boundary fill is the algorithm used frequently in computer graphics to fill a desired color inside a closed polygon having the same boundary color for all of its sides.
Required | Explaination |
---|
xcenter | x-coordinate of center point |
ycenter | y-coordinate of center point |
bordercolor | exisiting bordercolor of |
newcolor | color() funtion required for new color to be filled |
seed | number of seed you need for FloodFill |
radius | radius of object in which doing floodfill. (optional) |
Parameter for Boundary FIll
xcenter: int, ycenter: int, bordercolor: tuple, newcolor: tuple, seeds: int, radius: int | None = None
Examples ( for 4 seed )
from graphicswithpython import boundaryfill, window,delay,color , circle ,rectangle,polygon
window(700,700)
rectangle(350,550,140,100,color("red"))
boundaryfill(400,600,bordercolor=color("red"),newcolor=color("green"),seeds=4)
circle(100,100,40,color("red"))
boundaryfill(100,100,bordercolor=color("red"),newcolor=color("green"),seeds=4)
rectangle(200,300,100,100,color("blue"))
boundaryfill(210,310,bordercolor=color("blue"),newcolor=color("green"),seeds=4)
polygon(points=((500,100),(500,200),(600,200),(630,150),(600,100)),color="red")
boundaryfill(530,150,bordercolor=color("red"),newcolor=color("green"),seeds=4)
delay(5000)
Examples (for 8 seed)
from graphicswithpython import boundaryfill, window, delay, color, circle, rectangle,triangle
window(700,700)
rectangle(250,350,140,100,color("white"))
circle(390,348,50,color("purple"))
triangle(325,410,270,500,380,500,color("yellow"))
rectangle(200,300,100,100,color("red"))
boundaryfill(210,310,bordercolor=color("red"),newcolor=color("green"),seeds=8)
boundaryfill(390,348,bordercolor=color("purple"),newcolor=color("green"),seeds=8,radius=49)
boundaryfill(325,470,bordercolor=color("yellow"),newcolor=color("green"),seeds=8)
delay(5000)
