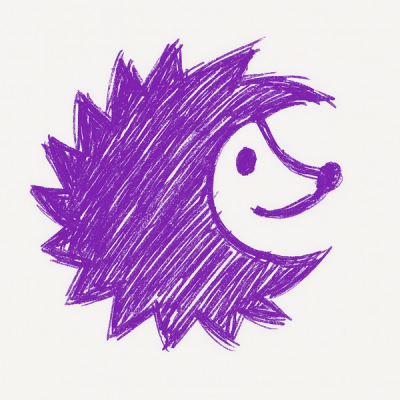
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
The Exsited Python SDK provides an easy-to-use library for integrating Exsited services into your project. This includes Custom Integration, Onsite Integration and all APIs.
Python 3.12 and Later
#Navigate to the project directory
cd exsited-python
# Install virtualenv
pip install virtualenv
# Create Virtual Environment
python -m venv venv
# Active virtual Environment from windows
venv\Scripts\activate
# Upgrade the pip
python -m pip install --upgrade pip
# Install setup tools
pip install setuptools
# Install app Dependency
pip install -e .
# Usage association Dependencies
pip install peewee
pip install mysql-connector-python
To set up the Exsited SDK, you'll require your Client ID
, Client Secret
, and Redirect URL
. If you have not received these details already, please reach out to your designated client contact to obtain them
Locate common_data.py
: Open the SKD project directory on an IDE and navigate to the common_data.py
file which is located in the following path: tests/common/common_data.py
.
Update get_request_token_dto
function: Within the common_data.py
class, locate the method named get_request_token_dto
and update it with the credentials you were provided.
Provide Credential Values: Populate the mandatory fields (clientId
, clientSecret
, redirectUri
, and ExsitedUrl
) within the RequestTokenDTO
object. However, replace the placeholder values in the following code block with your actual credentials:
def get_request_token_dto():
return RequestTokenDTO(
grantType="client_credentials",
clientId="[YOUR_CLIENT_ID]", # Replace with your actual Client ID
clientSecret="[YOUR_CLIENT_SECRET]", # Replace with your actual Client Secret
redirectUri="[YOUR_REDIRECT_URI]", # Replace with your actual Redirect URL
ExsitedUrl="[YOUR_EXSITED_SERVER_URL]" # Replace with your Exsited server URL,
)
Key | value |
---|---|
clientId | "[YOUR_CLIENT_ID]" |
clientSecret | "[YOUR_CLIENT_SECRET]" |
redirectUri | "[YOUR_REDIRECT_URI]" |
ExsitedUrl | "[YOUR_EXSITED_SERVER_URL]" |
Follow the common pattern to test the functions on the SDK. All the tests can be done on the test files located in the Tests directory.
test_account_create_basic
Parameter | Description | Type | Required |
---|---|---|---|
account | Contains the account details to be created. | AccountDataDTO | Yes |
account.name | The name of the account. | str | Yes |
account.emailAddress | The email address associated with the account. | str | Yes |
{
"account": {
"name": "Example Name",
"emailAddress": "example@example.com"
}
}
def test_account_create_basic():
SDKConfig.PRINT_REQUEST_DATA = False
SDKConfig.PRINT_RAW_RESPONSE = False
exsited_sdk: ExsitedSDK = ExsitedSDK().init_sdk(request_token_dto=CommonData.get_request_token_dto())
try:
# You will edit the following request_data
request_data = AccountCreateDTO(account=AccountDataDTO(name="Example Name", emailAddress="example@example.com"))
response = exsited_sdk.account.create(request_data=request_data)
print(response)
except ABException as ab:
print(ab)
print(ab.get_errors())
print(ab.raw_response)
test_order_create_basic
Parameter | Description | Type | Required |
---|---|---|---|
accountId | The ID of the account associated with the order. | str | Yes |
item_id | The ID of the item being ordered. | str | Yes |
quantity | The quantity of the item being ordered. | str | Yes |
{
"order": {
"accountId": "30PS79",
"lines": [
{
"item_id": "ITEM-0055",
"quantity": "1"
}
]
}
}
def test_order_create_basic():
SDKConfig.PRINT_REQUEST_DATA = True
SDKConfig.PRINT_RAW_RESPONSE = False
exsited_sdk: ExsitedSDK = ExsitedSDK().init_sdk(request_token_dto=CommonData.get_request_token_dto())
try:
# You will edit the following request_data
request_data = OrderCreateDTO(order=OrderDataDTO(accountId="30PS79").add_line(item_id="ITEM-0055", quantity="1"))
response = exsited_sdk.order.create(request_data=request_data)
print(response)
except ABException as ab:
print(ab)
print(ab.get_errors())
print(ab.raw_response)
Field | Description |
---|---|
response | The response from the the method being called. |
errors | Any errors encountered during the account creation process. |
Field | Description |
---|---|
ab | The exception object. |
ab.get_errors() | A list of errors that occurred during the account creation process. |
ab.raw_response | The raw response data from the API call, useful for debugging. |
To test the SDK functions, adhere to the common pattern outlined below. All tests should be conducted using the provided test files located in the "Tests" directory.
Set up the environment: Ensure that the SDK configuration is appropriately initialized for testing purposes.
Customize request data: Adjust the request_data
as needed for the specific function being tested.
Execute the function: Call the desired function from the SDK, updating the request_data
inside the function body.
The following tables contain for the required fields for the test cases
Function Name | Required Fields |
---|---|
test_account_create_basic | name, emailAddress |
test_account_update_info | id (Account ID) |
test_account_list_basic | n/a |
test_account_details | id (Account ID) |
test_account_delete | id (Account ID) |
test_account_payment_methods_add | processorType, default, paymentProcessor, reference |
test_account_payment_card_methods_add | processorType, default, paymentProcessor, reference, cardType, token, cardNumber, expiryMonth, expiryYear |
test_list_payment_methods | account_id (Account ID) |
test_delete_payment_methods | account_id (Account ID), reference |
test_payment_method_details | account_id (Account ID), reference |
Function | Required Parameters |
---|---|
test_order_create_basic | accountId, item_id, quantity (Item Quantity) |
test_order_list_basic | n/a |
test_order_details | id (Order ID) |
test_order_cancel | id (Order ID), effective_date |
test_order_usage_add | chargeItemUuid, chargingPeriod, quantity, startTime, endTime, type |
Function | Required Parameters |
---|---|
test_invoice_list | n/a |
test_invoice_details | id |
charge_item_uuid
AssociationThe order_usage_db
folder contains the functionality for handling database operations related to orders within the Exsited SDK. This module is responsible for connecting to the database, managing orders, processing order data, and saving the relevant information to the database.
order_usage_db
connect_with_db.py
order_manager.py
charge_item_uuid
order_model.py
order_service.py
save_to_db.py
connect_with_db.py
module to perform save operations.After configuring your database connection in connect_with_db.py
, you can use the other scripts to manage orders and persist data to your database. For example, you can create and process an order using order_manager.py
and then save it using save_to_db.py
.
Adding Database Details: In the SaveToDB class within the order_usage_db module, ensure that you update the OrderManager initialization with your specific database connection details, such as the database name, username, password, and host address.
class SaveToDB:
def process_order_data(_order_id: str, _account_id: str, _item_id: str, _item_name: str, _charge_item_uuid: str):
# Add your database details below
order_manager = OrderManager('your_database_name', 'your_username', 'your_password', 'your_host_address')
order_manager.connect_to_db()
order_manager.process_order(
account_id=_account_id,
order_id=_order_id,
item_id=_item_id,
item_name=_item_name,
charge_item_uuid=_charge_item_uuid
)
order_manager.disconnect_from_db()
Once the database details and other necessary configurations have been added, you can use the following example to add an order. This will automatically update the association tables, allowing you to retrieve the relevant details needed to update usage data.
def test_order_create_basic():
SDKConfig.PRINT_REQUEST_DATA = True
SDKConfig.PRINT_RAW_RESPONSE = False
exsited_sdk: ExsitedSDK = ExsitedSDK().init_sdk(request_token_dto=CommonData.get_request_token_dto())
try:
request_data = OrderCreateDTO(
order=OrderDataDTO(accountId="AC01").add_line(item_id="ITEM-001", quantity="1"))
response = exsited_sdk.order.create(request_data=request_data)
if response.order:
account_id = response.order.accountId
order_id = response.order.id
for line in response.order.lines:
if line.itemChargeType == 'METERED':
#Note: The item must be of type "Metered" for the association data to be stored.
SaveToDB.process_order_data(_account_id=account_id, _order_id=order_id, _item_id=line.itemId,
_item_name=line.itemName, _charge_item_uuid=line.chargeItemUuid)
except ABException as ab:
print(ab)
print(ab.get_errors())
print(ab.raw_response)
FAQs
This is the sdk support for account
We found that ab-py demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.