AIOCV
aiocv Is A Python Library Used To Track Hands, Track Pose, Detect Face, Detect Contours (Shapes), Detect Cars, Detect Number Plate, Detect Smile, Detect Eyes, Control Volume Using Gesture, Read QR Codes And Create Face Mesh On Image/Video.
Installation
Use the package manager pip to install aiocv.
pip install aiocv
Usage
Hand Tracking
import aiocv
import cv2
img = cv2.imread("hands.png")
hands = aiocv.HandTrack()
hands.findHands(img,draw=True)
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findHands() Method :
findHands(self,img=None,draw=True)
If You Are Not Getting Desired Results, Consider Changing detectionConfidence = 1 and trackConfidence = 1
Output :
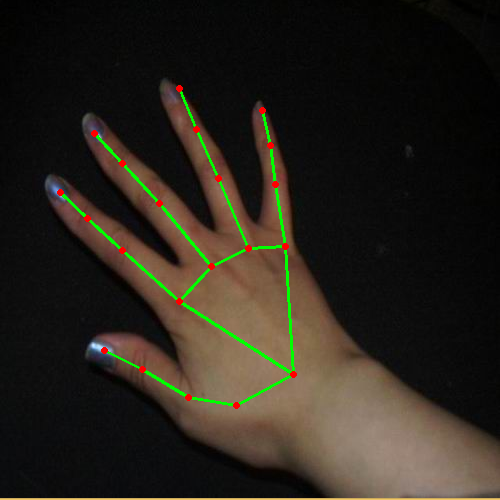
Pose Detector
import aiocv
import cv2
img = cv2.imread("man.png")
pose = aiocv.PoseDetector()
pose.findPose(img,draw=True)
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findPose() Method :
findPose(self,img=None,draw=True)
If You Are Not Getting Desired Results, Consider Changing detectionConfidence = 1 and trackConfidence = 1
Output :
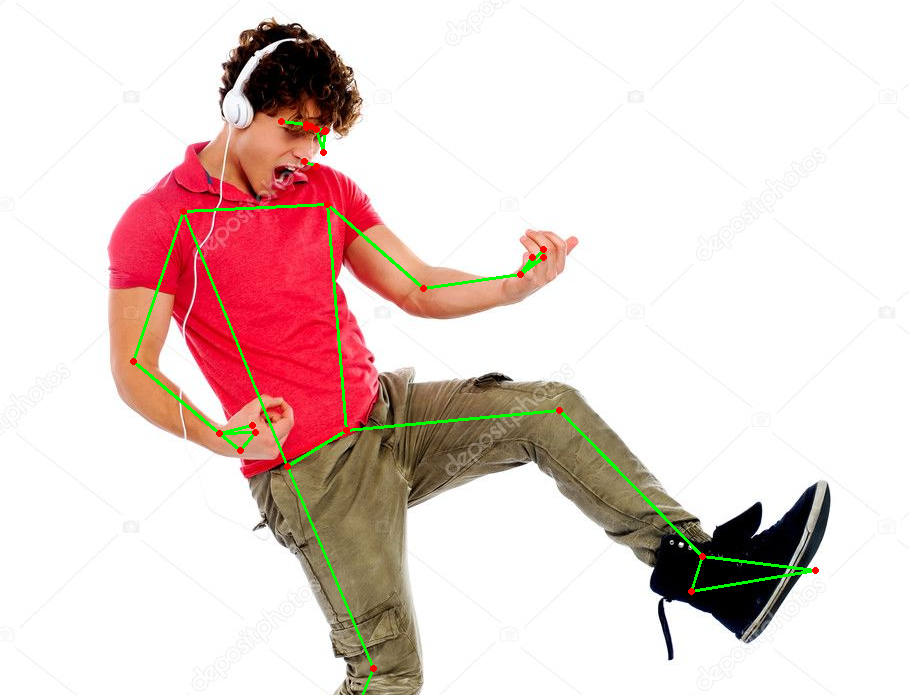
Face Detection
import aiocv
import cv2
img = cv2.imread("elon_musk.png")
face = aiocv.FaceDetector()
face.findFace(img,draw=True)
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findFace() Method :
findFace(self,img=None,draw=True)
If You Are Not Getting Desired Results, Consider Changing detectionConfidence = 1
Output :
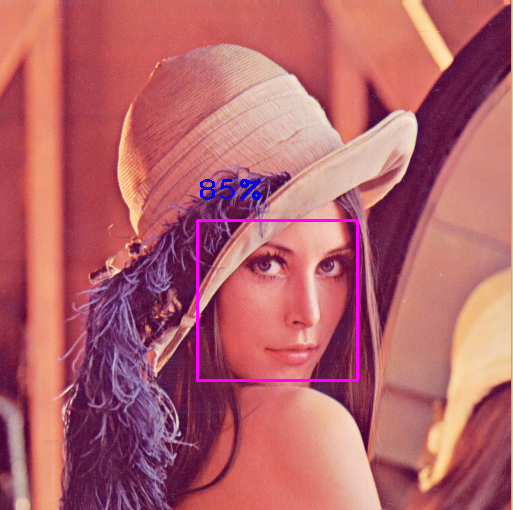
Face Mesh
import aiocv
import cv2
img = cv2.imread("elon_musk.png")
mesh = aiocv.FaceMesh()
mesh.findFaceMesh(img,draw=True)
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findFaceMesh() Method :
findFaceMesh(self,img=None,draw=True)
If You Are Not Getting Desired Results, Consider Changing detectionConfidence = 1 and trackConfidence = 1
Output :
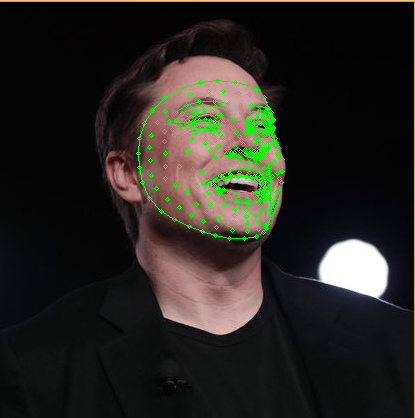
Contour (Shape) Detection
import aiocv
import cv2
img = cv2.imread("shapes.png")
shape = aiocv.ContourDetector(img)
shape.findContours(img,draw=True)
cv2.imshow("Image",img)
cv2.waitKey(0)
Output :
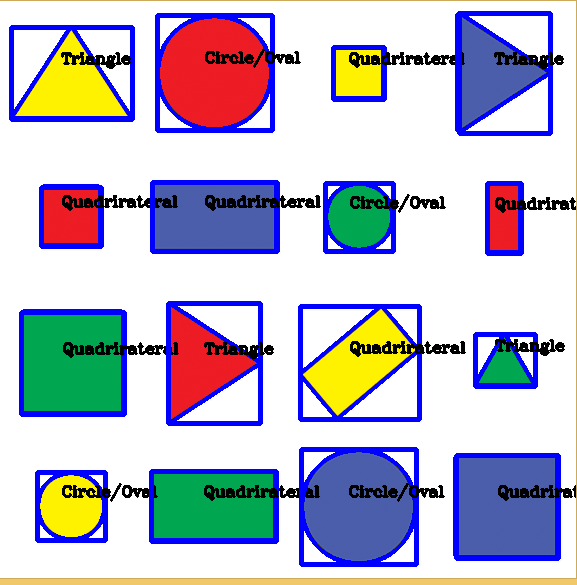
Car Detection
import aiocv
import cv2
img = cv2.imread("car.png")
car = aiocv.CarDetector(img)
car.findCars()
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findCars() Method :
findCars(self,color=(255,0,0),thickness=2)
Output :
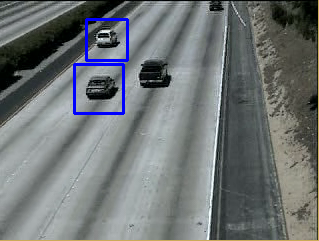
Number Plate Detection
import aiocv
import cv2
img = cv2.imread("car.png")
car = aiocv.NumberPlateDetector(img)
car.findNumberPlate()
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findNumberPlate() Method :
findNumberPlate(self,color=(255,0,0),thickness=2)
Output :
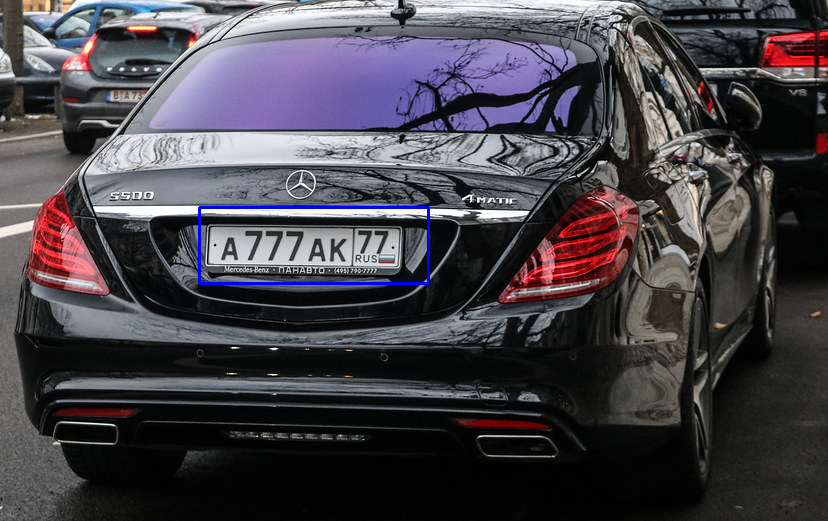
Smile Detection
import aiocv
import cv2
img = cv2.imread("person.png")
smile = aiocv.SmileDetector(img)
smile.findSmile()
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findSmile() Method :
findSmile(self,color=(255,0,0),thickness=2)
Output :
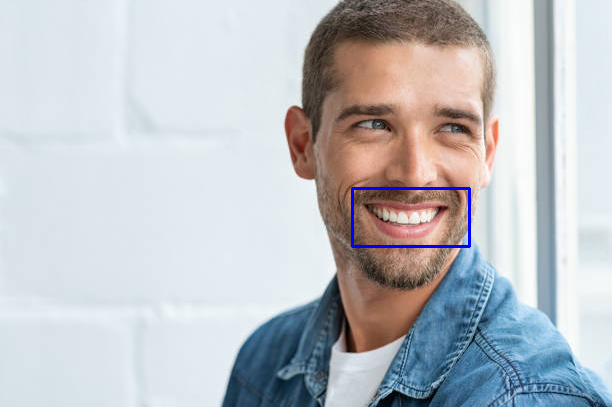
Eyes Detection
import aiocv
import cv2
img = cv2.imread("person.png")
eyes = aiocv.EyesDetector(img)
eyes.findEyes()
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findEyes() Method :
findEyes(self,color=(255,0,0),thickness=2)
Output :
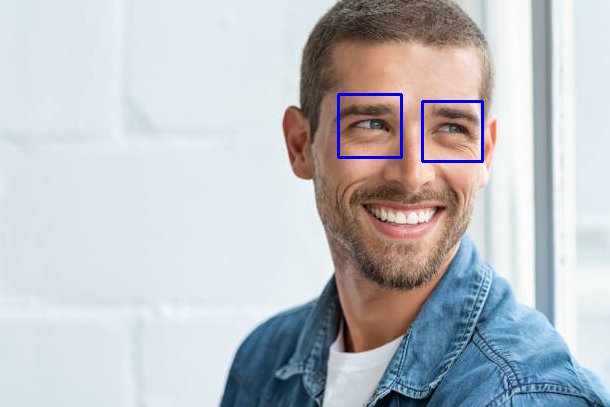
Control Volume Using Gesture
import aiocv
gvc = aiocv.GestureVolumeControl()
gvc.controlVolume()
Params For controlVolume() Method :
controlVolume(self,color=(255,0,0),thickness=2)
Params For GestureVolumeControl Class :
gvc = aiocv.GestureVolumeControl(webcamIndex = 0)
Output :
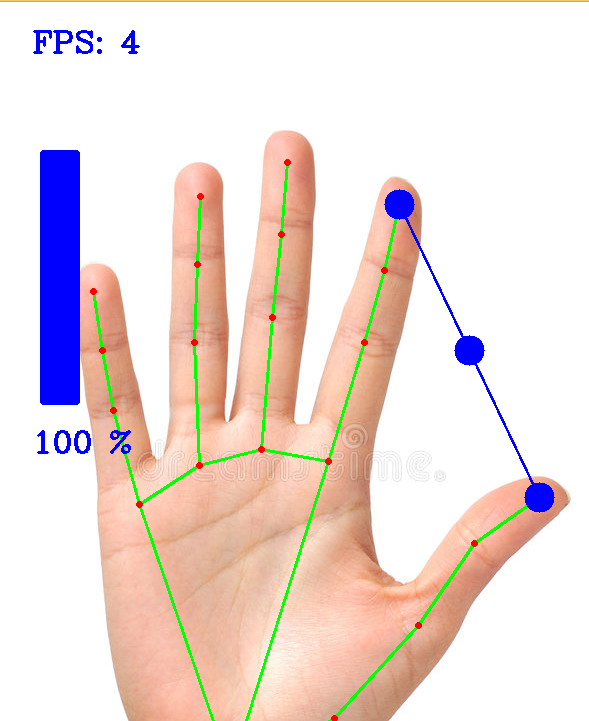
Read QR Code
import aiocv
import cv2
img = cv2.imread("qr.png")
qr = aiocv.QRCodeReader(img)
text=qr.findQRCode()
cv2.imshow("Image",img)
cv2.waitKey(0)
Params For findQRCode() Method :
findQRCode(self,color=(255,0,0),thickness=3)
print(text)
Output :
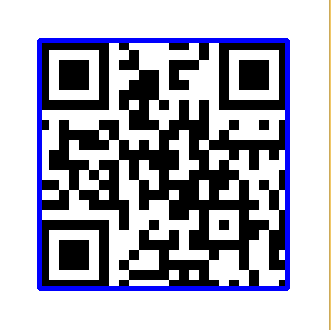
Contributing
Pull Requests Are Welcome. For Major Changes, Please Open An Issue First To Discuss What You Would Like To Change.
Please Make Sure To Update Tests As Appropriate.
License
MIT