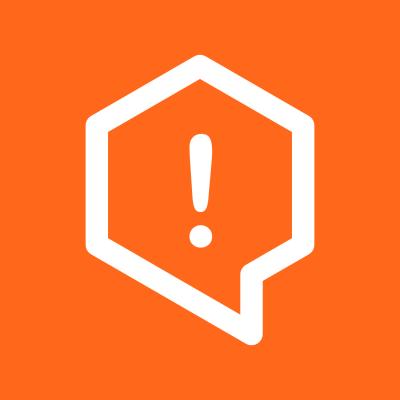
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
This package is designed to replace the
algoliasearch
package in asynchronous environments.
This package is only compatible with python 3.4 and onward.
Is compatible with python asyncio
.
Provide asynchronous alternatives to most of the client methods.
All those methods are just suffixed by _async
(search_async
,
add_object_async
, etc.)
Still provide synchronous versions of the methods.
Uses aiohttp
as the HTTP underlying library.
Uses __aexit__
to avoid manually closing aiohttp
sessions with
python >= 3.5.1.
Implement the search_disjunctive_faceting
method.
Support task canceling (yet).
Most of the logic of the synchronous client is being used here, so this
client depends on the synchronous one. It also depends on aiohttp
.
To install this package: pip install algoliasearchasync
.
All the asynchronous functions have the same names as the synchronous ones
with _async
appended. Synchronous methods keep the same name.
Arguments taken by the asynchronous functions are the same as the synchronous one, for documentation on the behavior of each function please see:
With python >= 3.4
import asyncio
from algoliasearchasync import ClientAsync
@asyncio.coroutine
def main(terms):
client = ClientAsync('<APP_ID>', '<API_KEY>')
index = client.init_index('<INDEX_NAME>')
# Create as many searches as there is terms.
searches = [index.search_async(term) for term in terms]
# Store the aggregated results.
s = yield from asyncio.gather(*searches)
# Client must be closed manually before exiting the program.
yield from client.close()
# Return the aggregated results.
return s
terms = ['<TERM2>', '<TERM2>']
loop = asyncio.get_event_loop()
# Start and wait for the tasks to complete.
complete = loop.run_until_complete(asyncio.gather(*searches))
for term, search in zip(terms, complete):
print('Results for: {}'.format(term))
# Display the field '<FIELD>' of each result.
print('\n'.join([h['<FIELD>'] for h in search['hits']]))
With python >= 3.5.1
import asyncio
from algoliasearchasync import ClientAsync
# Define a coroutine to be able to use `async with`.
async def main(terms):
# Scope the client for it to be closed automatically.
async with ClientAsync('<APP_ID>', '<API_KEY>') as client:
index = client.init_index('<INDEX_NAME>')
# Create as many searches as there is terms.
searches = [index.search_async(term) for term in terms]
# Return the aggregated results.
return await asyncio.gather(*searches)
terms = ['<TERM1>', '<TERM2>']
loop = asyncio.get_event_loop()
# Start and wait for the tasks to complete.
complete = loop.run_until_complete(main(terms))
for term, search in zip(terms, complete):
print('Results for {}'.format(term))
# Display the field '<FIELD>' of each result.
print('\n'.join([h['<FIELD>'] for h in search['hits']]))
FAQs
Algolia Search Asyncronous API Client for Python
We found that algoliasearchasync demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.