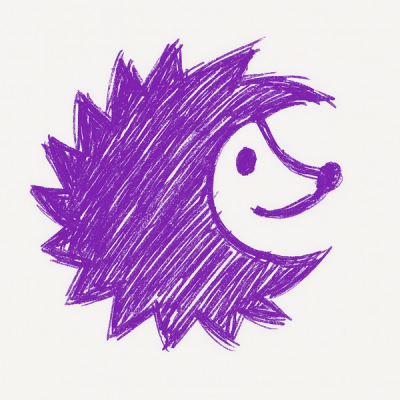
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Features
[5]. Chat with bing
You can click the table of contents to jump to the corresponding content.
cookie
: bing.com cookie, need to use browser extension cookie-editor
Export cookie to json in bing chat interface, then pass file path of str or Path, can also directly pass
list[dict] formatted cookieproxy
: LAN proxy, need to fill in when there is no global proxy or split tunneling on local machine, support
http, httpx, socks5import asyncio
from async_bing_client import Bing_Client
client = Bing_Client(cookie="cookie.json", proxy="socks5://127.0.0.1:7890")
if __name__ == '__main__':
async def main():
await client.init()
asyncio.run(main())
# The new conversation created here can be directly used for ask_stream
chat = await client.create_chat()
Contains chat history of all latest 200 conversations
chat_list = client.chat_list
# Just reinitialize
await client.init()
Parameters:
count: int = 20
: Number to deletedel_all: bool = False
: Whether to delete all (up to 200, because bing can only load latest 200 conversations)await client.delete_conversation_by_count()
Parameters:
conversation_id:str
: conversation_id of chat to delete (chat key is conversation_id)await client.delete_conversation('conversation_id')
Can pass pictures for image recognition, can also return Image type or md image format including new ai drawings generated by bing
Parameters:
question: str
: Your questionimage: str | Path | bytes = None
: Image you want to recognize (str here can be link of web image, can also be
str of path)chat: dict = None
: Chat window to send to, will create new one if not passed, and yield a NewChat class
containing the new chatconversation_style: ConversationStyle = ConversationStyle.Creative
: Conversation style to usepersonality=None
: Preset personality to load (just needs to be normal text, will auto obfuscate), content must
be within bing's moral and legal limits, otherwise will keep returning Apologylocale:str =guess_locale()
: Locale to use (usually don't need to specify yourself)Return types:
AsyncGenerator[NewChat | Apology | Notice | SearchResult | Text | SourceAttribution | SuggestRely | Limit | Response]
Text
: bing's text reply. Can access content attribute or use str method to get content. Addition of Text will still
return TextImage
: bing's image reply. url attribute is image link, base64 (Optional) attribute is image base64 content, name is
image nameNewChat
: Newly generated chat, can access chat attribute of NewChat to obtain.Apology
: bing's refusal or error message. Can access content attribute or use str method to obtain contentNotice
: bing's notification message. Can access content attribute or use str method to obtain contentSearchResult
: bing's search results. content attribute stores list[dict] or str. Can use str method to convert to
suitable str formatSourceAttribution
: bing's resource link reply, display_name attribute is displayed link name, see_more_url attribute
is link, image (optional) attribute is resource preview imageSuggestRely
: bing's suggested reply. Can access content attribute or use str method to obtain contentLimit
: bing's conversation limit content, will return Apology after exceeding limit. num_user_messages attribute is
number of messages user has sent, max_num_user_messages is max number of consecutive messages user can sendResponse
: Summary of bing's messages in this conversation, content stores dict of all data generated in this
conversation.
ask_stream function wraps ask_stream_raw examplechat = client.create_chat()
sources = []
suggest_reply = []
images = []
limit = None
async for data in client.ask_stream_raw('question', 'image', chat=chat):
if isinstance(data, Text):
yield data.content
elif isinstance(data, SuggestRely):
suggest_reply.append(data)
elif isinstance(data, SourceAttribution):
sources.append(data)
elif isinstance(data, Apology):
yield '\n' + data.content
elif isinstance(data, Image):
images.append(data)
elif isinstance(data, Limit):
limit = data
elif isinstance(data, SearchResult) and yield_search:
yield str(data)
for index, source in enumerate(sources):
if index == 0:
yield "\nSee more: \n"
yield f"({index + 1}):{source} \n"
for index, image in enumerate(images):
if index == 0:
yield "\nDrew images: \n"
yield f"{index + 1}:{image} \n"
for index, reply in enumerate(suggest_reply):
if index == 0:
yield "\nSuggest Replys: \n"
yield f"{index + 1}:{reply.content} \n"
if limit:
yield f"\n\nLimit:{limit.num_user_messages} of {limit.max_num_user_messages} "
async for text in client.ask_stream("hello", chat=chat,
yield_search=False,
conversation_style=ConversationStyle.Balanced):
print(text, end="")
(This function will be called automatically in ask_stream, can directly use human language to let bing generate images)
Return value: List[Image]
Image
: bing's image reply. url attribute is image link, name is image name
images = await client.draw("drawing prompt")
FAQs
Async Client for Newbing.
We found that async-bing-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.