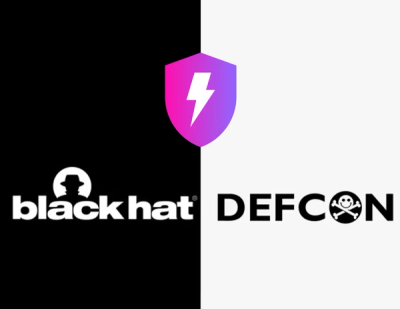
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
CONTENTS
pipeline.auto_load_lora_weights()
and pipeline.auto_load_textual_inversion()
may not work properly at the moment due to a bug in the Civitai API.
Enhance the functionality of diffusers.
pip install --quiet auto_diffusers
from auto_diffusers import EasyPipelineForText2Image
# Search for Huggingface
pipe = EasyPipelineForText2Image.from_huggingface("search_word").to("cuda")
img = pipe("cat").images[0]
img.save("cat.png")
# Search for Civitai
pipe = EasyPipelineForText2Image.from_civitai("search_word").to("cuda")
image = pipe("cat").images[0]
image.save("cat.png")
# Load Lora into the pipeline.
pipe.auto_load_lora_weights("Detail Tweaker")
# Load TextualInversion into the pipeline.
pipe.auto_load_textual_inversion("EasyNegative", token="EasyNegative")
[!TIP] If an error occurs, insert the
token
and run again.
EasyPipeline.from_civitai
parametersName | Type | Default | Description |
---|---|---|---|
search_word | string, Path | ー | The search query string. Can be a keyword, Civitai URL, local directory or file path. |
model_type | string | Checkpoint | The type of model to search for. (for example Checkpoint , TextualInversion , Controlnet , LORA , Hypernetwork , AestheticGradient , Poses ) |
base_model | string | None | Trained model tag (for example SD 1.5 , SD 3.5 , SDXL 1.0 ) |
torch_dtype | string, torch.dtype | None | Override the default torch.dtype and load the model with another dtype. |
force_download | bool | False | Whether or not to force the (re-)download of the model weights and configuration files, overriding the cached versions if they exist. |
cache_dir | string, Path | None | Path to the folder where cached files are stored. |
resume | bool | False | Whether to resume an incomplete download. |
token | string | None | API token for Civitai authentication. |
search_civitai
parametersName | Type | Default | Description |
---|---|---|---|
search_word | string, Path | ー | The search query string. Can be a keyword, Civitai URL, local directory or file path. |
model_type | string | Checkpoint | The type of model to search for. (for example Checkpoint , TextualInversion , Controlnet , LORA , Hypernetwork , AestheticGradient , Poses ) |
base_model | string | None | Trained model tag (for example SD 1.5 , SD 3.5 , SDXL 1.0 ) |
download | bool | False | Whether to download the model. |
force_download | bool | False | Whether to force the download if the model already exists. |
cache_dir | string, Path | None | Path to the folder where cached files are stored. |
resume | bool | False | Whether to resume an incomplete download. |
token | string | None | API token for Civitai authentication. |
include_params | bool | False | Whether to include parameters in the returned data. |
skip_error | bool | False | Whether to skip errors and return None. |
[!TIP] If an error occurs, insert the
token
and run again.
EasyPipeline.from_huggingface
parametersName | Type | Default | Description |
---|---|---|---|
search_word | string, Path | ー | The search query string. Can be a keyword, Hugging Face URL, local directory or file path, or a Hugging Face path (<creator>/<repo> ). |
checkpoint_format | string | single_file | The format of the model checkpoint. ● single_file to search for single file checkpoint ● diffusers to search for multifolder diffusers format checkpoint |
torch_dtype | string, torch.dtype | None | Override the default torch.dtype and load the model with another dtype. |
force_download | bool | False | Whether or not to force the (re-)download of the model weights and configuration files, overriding the cached versions if they exist. |
cache_dir | string, Path | None | Path to a directory where a downloaded pretrained model configuration is cached if the standard cache is not used. |
token | string, bool | None | The token to use as HTTP bearer authorization for remote files. |
search_huggingface
parametersName | Type | Default | Description |
---|---|---|---|
search_word | string, Path | ー | The search query string. Can be a keyword, Hugging Face URL, local directory or file path, or a Hugging Face path (<creator>/<repo> ). |
checkpoint_format | string | single_file | The format of the model checkpoint. ● single_file to search for single file checkpoint ● diffusers to search for multifolder diffusers format checkpoint |
pipeline_tag | string | None | Tag to filter models by pipeline. |
download | bool | False | Whether to download the model. |
force_download | bool | False | Whether or not to force the (re-)download of the model weights and configuration files, overriding the cached versions if they exist. |
cache_dir | string, Path | None | Path to a directory where a downloaded pretrained model configuration is cached if the standard cache is not used. |
token | string, bool | None | The token to use as HTTP bearer authorization for remote files. |
include_params | bool | False | Whether to include parameters in the returned data. |
skip_error | bool | False | Whether to skip errors and return None. |
In accordance with Apache-2.0 license
I have used open source resources and free tools in the creation of this project.
I would like to take this opportunity to thank the open source community and those who provided free tools.
FAQs
diffusers with search engine
We found that auto-diffusers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.