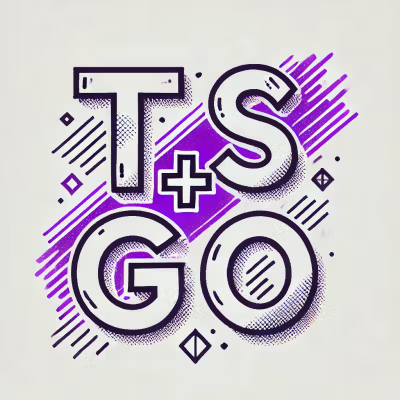
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
A Python client for the Box API, implementing multiple third-party APIs like Instagram.
A Python client for interacting with the Box API for Instagram. This library wraps multiple Instagram endpoints including user information, media retrieval, and direct messages, allowing you to easily integrate Instagram functionality into your Python applications.
You can install the package via pip or Poetry.
pip install boxapi
poetry add boxapi
boxapi-python/
├── boxapi/
│ ├── __init__.py # Package initializer, re-exports public API
│ ├── constants.py # Global constants and endpoint paths
│ ├── client.py # BoxApiClient: top-level client for Box API
│ ├── instagram/
│ │ ├── __init__.py # Makes the instagram module importable
│ │ ├── api_client.py # InstagramAPIClient: general Instagram endpoints
│ │ └── dm_client.py # InstagramDMClient: direct message endpoints
│ └── utils/
│ └── base_url_session.py # Custom session with base URL support
├── examples/ # Example scripts demonstrating usage
├── tests/ # (Optional) Unit tests
├── pyproject.toml # Build configuration for Poetry
├── LICENSE # License file
└── README.md # This file
from boxapi import BoxApiClient
# Initialize BoxApiClient with Box API credentials
# IMPORTANT: MAKE SURE YOU ARE READING YOUR USERNAME AND PASSWORD FROM ENVIRONMENT VARIABLES
box_client = BoxApiClient("your_boxapi_username", "your_boxapi_password")
# Use the Instagram sub-client for general endpoints
user_info = box_client.instagram.get_user_info("leomessi")
print(user_info)
Direct Message functionality is available through a separate sub-client. Instantiate it when needed:
from boxapi import BoxApiClient
# Initialize BoxApiClient with Box API credentials
box_client = BoxApiClient("your_boxapi_username", "your_boxapi_password")
# Use DM endpoints by providing the Instagram account credentials per call
login_response = box_client.instagram_dm.direct_login("insta_username", "insta_password")
print(login_response)
Check out the examples folder for complete scripts demonstrating how to use the different features of this library (It's a work in progress).
Contributions are welcome! Feel free to fork the repository and submit pull requests. Please review our contributing guidelines for more information.
This project is licensed under the MIT License. See the LICENSE file for details.
FAQs
A Python client for the Box API, implementing multiple third-party APIs like Instagram.
We found that boxapi demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.