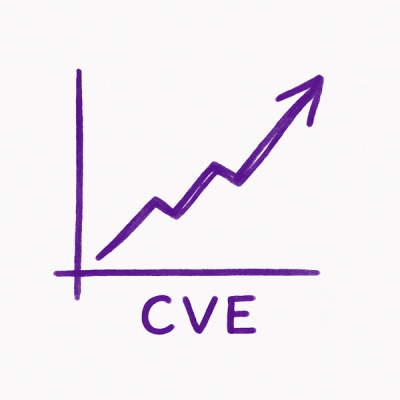
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
concurrent-progressbar
Advanced tools
This package is used to track the processes executed in the program. It will show individual progress bar for each task alloted using this package. It runs each task in separate process.
This package is used to track the processes executed in the program. It will show individual progress bar for each task alloted using this package. It runs each task in separate process.
These instructions will get you a copy of the project up and running on your local machine for development and testing purposes.
pip install concurrent-progressbar
import os
from concurrent_progressbar.concurrent import Multithreading, MultiProcessing
def target_1(i):
pass
def target_2(j):
pass
pool = Multithreading(
num_workers=os.cpu_count(),
target=[target_1, target_2],
args=[[(i,) for i in range(1000)], [(j,) for j in range(100)]]
)
pool.run()
# OR
pool = Multiprocessing(
num_workers=os.cpu_count(),
target=[target_1, target_2],
args=[[(i,) for i in range(1000)], [(j,) for j in range(100)]]
)
pool.run()
pool = Multiprocessing(
num_workers=os.cpu_count(),
target=[target_1, target_2],
args=[[(i,) for i in range(1000)], [(j,) for j in range(100)]],
task_desc=["my-task-1", "my-task-2"],
tasks_at_a_time=1
)
# tasks_at_a_time=1 will run one task, when this task completed
# then next task will be executed. It is independent from num_workers argument.
pool.run()
FAQs
This package is used to track the processes executed in the program. It will show individual progress bar for each task alloted using this package. It runs each task in separate process.
We found that concurrent-progressbar demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.