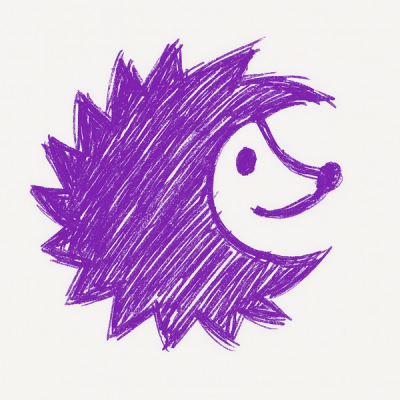
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
django-computedfields
Advanced tools
django-computedfields provides autoupdated database fields for model methods.
Tested with Django 3.2 and 4.2 (Python 3.8 to 3.11).
Just derive your model from ComputedFieldsModel
and place
the @computed
decorator at a method:
from django.db import models
from computedfields.models import ComputedFieldsModel, computed
class MyModel(ComputedFieldsModel):
name = models.CharField(max_length=32)
@computed(models.CharField(max_length=32), depends=[('self', ['name'])])
def computed_field(self):
return self.name.upper()
computed_field
will be turned into a real database field
and can be accessed and searched like any other database field.
During saving the associated method gets called and it’s result
written to the database.
If you need to recalculate the computed field but without saving it, use
from computedfields.models import compute
>>> from computedfields.models import compute
>>> person = MyModel.objects.get(id=1) # this is to retrieve existing record
>>> person.computed_field # outputs 'BERTY'
>>> person.name = 'nina' # changing the dependent field `name` to nina
>>> compute(person, 'computed_field') # outputs 'NINA'
>>> person.computed_field # outputs 'BERTY' because the `person` is not yet saved
>>> person.save() # alters the database record for `name` and `computed_field`
>>> person.computed_field # outputs 'NINA'
depends
keywordThe depends
keyword argument can be used with any relation to indicate dependencies to fields on other models as well:
from django.db import models
from computedfields.models import ComputedFieldsModel, computed
class MyModel(ComputedFieldsModel):
name = models.CharField(max_length=32)
fk = models.ForeignKey(SomeModel)
@computed(
models.CharField(max_length=32),
depends=[
('self', ['name']),
('fk', ['fieldname'])
]
)
def computed_field(self):
return self.name.upper() + self.fk.fieldname
Now changes to self.name
, fk
or fk.fieldname
will update computed_field
.
Instead of using the @computed
decorator with inline field definitions,
you can also use a more declarative syntax with ComputedField
, example from above rewritten:
from django.db import models
from computedfields.models import ComputedFieldsModel, ComputedField
def get_upper_string(inst):
return inst.name.upper()
class MyModel(ComputedFieldsModel):
name = models.CharField(max_length=32)
computed_field = ComputedField(
models.CharField(max_length=32),
depends=[('self', ['name'])],
compute=get_upper_string
)
The documentation can be found here.
0.2.10
0.2.9
0.2.8
0.2.7
0.2.6
0.2.5
0.2.4
ComputedField
for a more declarative code style added0.2.3
0.2.2
model._base_manager
instead of model.objects
to prevent using overridden models.objects
with a custom manager0.2.1
0.2.0 - next beta release
new features:
COMPUTEDFIELDS_QUERYSIZE
or as argument on @computed
COMPUTEDFIELDS_FASTUPDATE
checkdata
commandshowdependencies
commandenhancements:
updatedata
commandremoved features:
update_dependent_multi
and preupdate_dependent_multi
(due to showing low benefit and being a code nuisance)bug fixes:
0.1.7
update_fields
in signal handlers0.1.6
maintenace version with CI test dependencies changes:
Note that Django 2.2 will keep working until real incompatible code changes occur. This may happen by any later release, thus treat 0.1.6 as last compatible version.
0.1.5
0.1.4
0.1.3
0.1.2
0.1.1
0.1.0
m2m_changed
handler with filtering on m2m fields@precomputed
for custom save methods0.0.23:
0.0.22:
0.0.21:
0.0.20
bulk_update
for computed field updates.compute
.update_dependent
simplifying bulk actions on ComputedFieldsModel
.0.0.19
0.0.18
0.0.17
FAQs
autoupdated database fields for model methods
We found that django-computedfields demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.