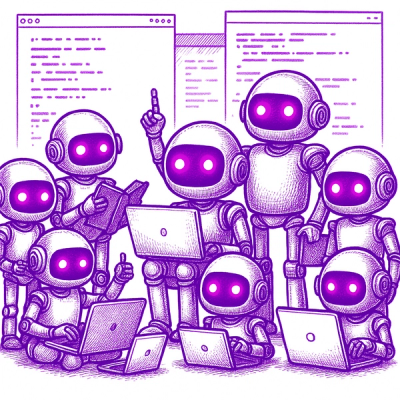
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
django-form-generator
Advanced tools
django form generator will help you create forms very easily. you can use it any where in your html files.
there is an API-manager tool for you to call some APIs on loading form or after submit.
install it via pip:
pip install django-form-generator
add ('django_form_generator'
, 'django_htmx'
, 'crispy_forms'
, 'crispy_bootstrap5'
, 'captcha'
, 'tempus_dominus', 'rest_framework', 'drf_recaptcha'
) to your INSTALLED_APPS
:
INSTALLED_APPS = [
...
'django_htmx',
'crispy_forms',
'crispy_bootstrap5',
'captcha',
'tempus_dominus',
'rest_framework',
'drf_recaptcha',
'django_form_generator',
]
do a migrate
:
python manage.py migrate
do a collectstatic
:
python manage.py collectstatic
finally include django_form_generator urls to your urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
...
path('form-generator/', include(('django_form_generator.urls', 'django_form_generator'), namespace='django_form_generator')),
...
]
<!doctype html>
<html lang="en">
<head>
...
</head>
<body>
{% load django_form_generator %}
{% render_form 1 %} {# it will render the form that has id of 1 #}
</body>
</html>
To use this tags on a Richtext field like CKeditor
or tinymce
.
Note: In some richtext packages some elements (specialy jinja elements) will be removed from the field there are some tweaks to solve this problems on the packages documentations.
CK-Editor example
./app_name/templates/index.html
<!doctype html>
<html lang="en">
<head>
...
</head>
<body>
{% load django_form_generator %}
{{ object.rich_text_field|eval_data }}
</body>
</html>
There is also an API manager for the forms
example:
Note:
first_name
,last_name
andjob
are the name of the fields that are defined in a form which this api is assigned to.
Note: assigend apis will be called automatically. the ones with execution_time of
PRE
will be called when form renders. and the ones with execution_time ofPOST
will be called after form submition.
Note: in the response field you can write HTML tags it will be rendrend as
safe
.
Note: You have access to
request
globaly in api manager
Also you have access to the response of the api. so you can use Jinja
syntax to access data.
You can use this feature on the (url
, body
, response
) fields of APIManager
model
example:
Note: To show the response use the template tag of
{% render_pre_api form_id api_id %}
inside your html file that theform
is there.
example:
output of the api:
{
"result": [
{
"first_name": "john",
"last_name": "doe",
"avatar": "https://www.example.com/img/john-doe.png",
},
{
"first_name": "mary",
"last_name": "doe",
"avatar": "https://www.example.com/img/mary-doe.png",
}
]
}
in the response field you can do:
<h1> Response of the API: </h1>
{% for item in result %}
<p>{{item.first_name}}</p>
<p>{{item.last_name}}</p>
<img src="{{item.avatar}}">
{% endfor %}
<!doctype html>
<html lang="en">
<head>
...
</head>
<body>
{% load django_form_generator %}
{% render_pre_api 1 %} {# it will render all pre apis that are assigned to a form with id of 1 #}
{% render_pre_api 1 6 %} {# it will render the pre api with id of 6 which is assigned to a form with id of 1 #}
{% render_post_api 1 %} {# it will render all pre apis that are assigned to a form with id of 1 #}
{% render_post_api 1 7 %} {# it will render the post api with id of 7 which is assigned to a form with id of 1 #}
</body>
</html>
you can access the whole submited data by adding {{ form_data }}
to your api body
.
to have multiple styles the html of template_name_p
(attribute of django.forms.Form) will be replace by our html file. ({{form.as_p}}
)
there are 3 styles(or actualy styel of rendering fields) for the forms:
Note: If you want to render two fields
inline
and one fieldinorder
you should usebreak
for the second field
field1 inline
field2 break
field3 inorder
Note: You can add your custom styles by create new TextChoices object and add it to
settings.py
myapp/const.py
from django.db.models import TextChoices
class CustomFormGeneratorStyle(TextChoices):
MY_STYLE = "myapp/templates/my_style.html", 'My Custom Style'
...
myproject/settings.py
DJANGO_FORM_GENERATOR = {
"FORM_STYLE_CHOICES": "myapp.const.CustomFormGeneratorStyle"
}
# to access the form you can call this url:
"""GET: http://127.0.0.1:8000/form-generator/form/1/"""
#or
reverse('django_form_generator:form_detail', kwargs={'pk': 1})
#api:
reverse('django_form_generator:api_form_detail', kwargs={'pk': 1})
# to access the response of the form you can call this url the kwarg_lookup is UUID field
"""GET: http://127.0.0.1:8000/form-generator/form-response/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/"""
#or
reverse('django_form_generator:form_response', kwargs={'unique_id': 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'})
#api:
reverse('django_form_generator:api_form_response', kwargs={'unique_id': 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'})
below is the default settings for django_form_generator. you can change them by adding it to your settings.py
DJANGO_FORM_GENERATOR = {
'FORM_RESPONSE_SAVE': 'django_form_generator.models.save_form_response',
'FORM_EVALUATIONS': {'form_data': '{{form_data}}'},
'FORM_GENERATOR_FORM': 'django_form_generator.forms.FormGeneratorForm',
'FORM_RESPONSE_FORM': 'django_form_generator.forms.FormGeneratorResponseForm',
'FORM_STYLE_CHOICES': 'django_form_generator.const.FormStyle',
'FORM_MANAGER': 'django_form_generator.managers.FormManager',
'FORM_GENERATOR_SERIALIZER': 'django_form_generator.api.serializers.FormGeneratorSerializer',
'FORM_RESPONSE_SERIALIZER': 'django_form_generator.api.serializers.FormGeneratorResponseSerializer',
}
To have nice styled fields you can add crispy-form to your settings.py
CRISPY_ALLOWED_TEMPLATE_PACKS = 'bootstrap5'
CRISPY_TEMPLATE_PACK = 'bootstrap5'
To access google recaptcha you should add these to your settings.py
Note: you can have public & private keys by registring your domain on Google recaptcha admin console
RECAPTCHA_PUBLIC_KEY = 'public_key'
RECAPTCHA_PRIVATE_KEY = 'private_key'
# ===== FOR DRF =========
DRF_RECAPTCHA_SECRET_KEY = "YOUR SECRET KEY"
Note: If you don't want to use google-recaptcha(
django-recaptcah
) you should add below code to yoursettings.py
SILENCED_SYSTEM_CHECKS = ['captcha.recaptcha_test_key_error']
FAQs
An app to create any form in django admin panel.
We found that django-form-generator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.