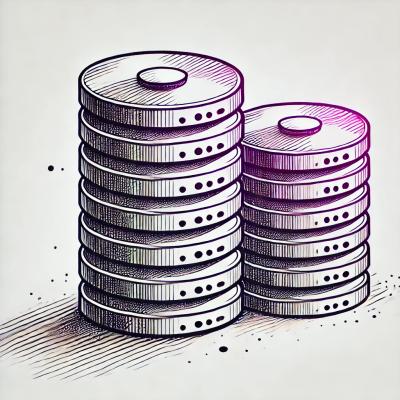
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
django-pagination-utils
Advanced tools
django-pagination-utils
is a library that extends the default paginator and provides utilities for pagination in Django. This library includes mixins and views that facilitate the implementation of these functionalities in your Django projects.
To install the library, run the following command:
pip install django-pagination-utils
This class provides pagination and ordering for a queryset. To use it, you must create a subclass of PaginatorView and define the necessary attributes.
Ejemplo:
from django_pagination_utils.paginator_view import PaginatorView
from .models import MyModel
from .filters import MyFilterSet
class MyView(PaginatorView):
model = MyModel
template_name = 'my_template.html'
filterset_class = MyFilterSet
allowed_fields_order = ['field1', 'field2', 'field3']
model: The Django model that will be used for the queryset. template_name: The name of the template that will be used to render the view. filterset_class: The filters class that will be applied to the queryset. allowed_fields_order: List of fields that can be used to order the queryset. If not provided, all fields will be allowed.
QueryOrderMixin
from django_pagination_utils.mixins.query_order_mixin import QueryOrderMixin
class MyOrderView(QueryOrderMixin):
model = MyModel
allowed_fields_order = ['field1', 'field2', 'field3']
The QueryFilterMixin provides functionalities to filter a queryset.
from django_pagination_utils.mixins.query_filter_mixin import QueryFilterMixin
class MyFilterView(QueryFilterMixin):
model = MyModel
filterset_class = MyFilterSet
The QueryFilterOrderMixin provides functionalities to filter and order a queryset.
from django_pagination_utils.mixins.query_filter_order_mixin import QueryFilterOrderMixin
class MyFilterOrderView(QueryFilterOrderMixin):
model = MyModel
filterset_class = MyFilterSet
allowed_fields_order = ['field1', 'field2', 'field3']
The library defines several exceptions that you can handle in your code:
from django_pagination_utils.paginator_view import PaginatorView
from .models import MyModel
from .filters import MyFilterSet
class MyView(PaginatorView):
model = MyModel
template_name = 'my_template.html'
filterset_class = MyFilterSet
allowed_fields_order = ['field1', 'field2', 'field3']
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['extra_data'] = 'some extra data'
return context
In your my_template.html template, you can access the paginated and ordered objects:
{% for obj in page_obj %}
{{ obj }}
{% endfor %}
<div class="pagination">
<span class="step-links">
{% if page_obj.has_previous %}
<a href="?page=1">« first</a>
<a href="?page={{ page_obj.previous_page_number }}">previous</a>
{% endif %}
<span class="current">
Page {{ page_obj.number }} of {{ page_obj.paginator.num_pages }}.
</span>
{% if page_obj.has_next %}
<a href="?page={{ page_obj.next_page_number }}">next</a>
<a href="?page={{ page_obj.paginator.num_pages }}">last »</a>
{% endif %}
</span>
</div>
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Django pagination utils
We found that django-pagination-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.