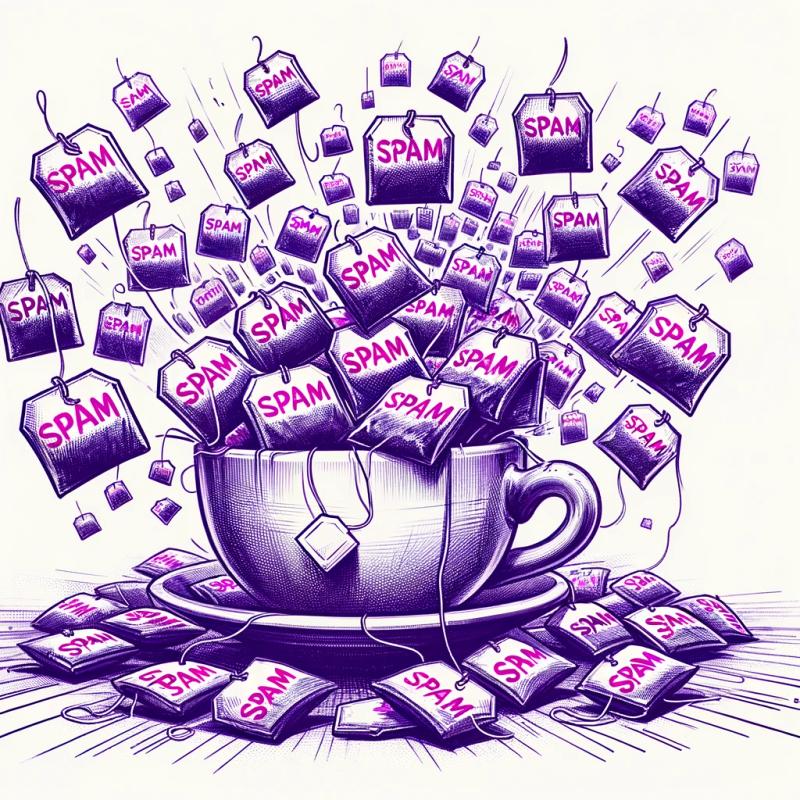
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
A library that allows the editing of content control XML components in .docx files.
Readme
This package allows you to easily modify the values of content controls & form fields in .docx
files with Python.
pip install docx-form
The file ./docx_form_tests/test.docx
wil be used for the following guide.
example.py
from docx_form import DocxForm
DocxForm
instance:...
full_path = 'path/to/docx/file/test.docx'
document = DocxForm(full_path)
...
document.print_all_content_controls_and_form_fields()
0: RichTextContentControl | id: -1012910137 | text: Rich Text Content Control
1: RichTextContentControl | id: -1135860421 | text: Another Rich Text Content Control
2: PlainTextContentControl | id: -132710284 | text: Plain Text Content Control
3: PlainTextContentControl | id: 28152470 | text: Another Plain Text Content Control
4: CheckBoxContentControl | id: -1942055255 | text: ☐
5: CheckBoxContentControl | id: -635946620 | text: ☒
6: ComboBoxContentControl | id: 199831773 | text: Combo t Option 1
7: ComboBoxContentControl | id: -1984237200 | text: Choose an item.
8: DropDownListContentControl | id: -827207619 | text: Drop-Down Content Control
9: DropDownListContentControl | id: 2026666311 | text: Another Drop-Down Content Control
10: DatePickerContentControl | id: 645172330 | text: 9/6/2022
11: DatePickerContentControl | id: 539787165 | text: 9/7/2022
Note: The Content Controls are listed starting from the top-left of the document going from left to right on each line of the document all the way to the bottom.
...
# Import type for proper intellisense in your editor/IDE
from docx_form.content_controls import RichTextContentControl
...
rich_text_control: RichTextContentControl = document.content_control_forms_and_form_fields[1]
rich_text_control.set_text("The example worked!")
...
# Note: This will overwrite the original file
document.save()
document.print_all_content_controls_and_form_fields()
is run again:...
1: RichTextContentControl | id: -1135860421 | text: The example worked!
...
from docx_form import DocxForm
from docx_form.content_controls import RichTextContentControl
# Create a DocxForm instance
full_path = "path/to/docx/file/test.docx"
document = DocxForm(full_path)
# Kept for reference
# document.print_all_content_controls_and_form_fields()
# Edit the second Rich Text content control
rich_text_control: RichTextContentControl = (
document.content_control_forms_and_form_fields[1]
)
rich_text_control.set_text("The example worked!")
# Note: This will overwrite the original file
document.save()
The file ./docx_form_tests/test.docx
wil be used for the following examples.
from docx_form import DocxForm
from docx_form.content_controls import PlainTextContentControl
# Create a DocxForm instance
full_path = "path/to/docx/file/test.docx"
document = DocxForm(full_path)
# Kept for reference
# document.print_all_content_controls_and_form_fields()
# Edit the content control (remember the console output)
content_control: PlainTextContentControl = (
document.content_control_forms_and_form_fields[2]
)
content_control.set_text("Plain text edit")
# Note: This will overwrite the original file
document.save()
from docx_form import DocxForm
from docx_form.content_controls import CheckBoxContentControl
# Create a DocxForm instance
full_path = "path/to/docx/file/test.docx"
document = DocxForm(full_path)
# Kept for reference
# document.print_all_content_controls_and_form_fields()
# Edit the content control (remember the console output)
content_control: CheckBoxContentControl = (
document.content_control_forms_and_form_fields[4]
)
content_control.set_check_box(True)
# Note: This will overwrite the original file
document.save()
from docx_form import DocxForm
from docx_form.content_controls import ComboBoxContentControl
# Create a DocxForm instance
full_path = "path/to/docx/file/test.docx"
document = DocxForm(full_path)
# Kept for reference
# document.print_all_content_controls_and_form_fields()
# Edit the content control (remember the console output)
content_control: ComboBoxContentControl = (
document.content_control_forms_and_form_fields[6]
)
content_control.print_options()
"""
Output:
0: Display Value = "None" || Value = "Choose an item."
1: Display Value = "Combo Box Option 1" || Value = "Combo Box Option 1"
2: Display Value = "Combo Box Option 2" || Value = "Combo Box Option 2"
"""
content_control.set_text(2)
# Note: This will overwrite the original file
document.save()
from docx_form import DocxForm
from docx_form.content_controls import DropDownListContentControl
# Create a DocxForm instance
full_path = "path/to/docx/file/test.docx"
document = DocxForm(full_path)
# Kept for reference
# document.print_all_content_controls_and_form_fields()
# Edit the content control (remember the console output)
content_control: DropDownListContentControl = (
document.content_control_forms_and_form_fields[8]
)
content_control.print_options()
"""
Output:
0: Display Value = "None" || Value = "Choose an item."
1: Display Value = "Drop-Down Content Control" || Value = "Drop-Down Content Control"
"""
content_control.set_option(0)
# Note: This will overwrite the original file
document.save()
from datetime import datetime
from docx_form import DocxForm
from docx_form.content_controls import DatePickerContentControl
# Create a DocxForm instance
full_path = "path/to/docx/file/test.docx"
document = DocxForm(full_path)
# Kept for reference
# document.print_all_content_controls_and_form_fields()
# Edit the content control (remember the console output)
content_control: DatePickerContentControl = (
document.content_control_forms_and_form_fields[10]
)
print(content_control.date_format) # Output: "M/d/yyyy"
new_date = datetime(1999, 12, 31)
content_control.set_date(new_date)
# Note: This will overwrite the original file
document.save()
PATH
(step 3)The following will be done in Visual Studio Code on a Windows machine:
poetry install
poetry env info
Virtualenv > Executable:
CTRL + SHIFT + P
to open the command menuinterpreter
and hit ENTER
+ Enter interpreter path...
ENTER
./docx_form/docx_form.py
(This is the root file, so do any local testing within the if __name__ == "__main__":
scope)Run
button in the top-right of the editor (assuming the Python Extension is installed)
Git Bash
terminal in the repository's root
curl -o- https://raw.githubusercontent.com/tapsellorg/conventional-commits-git-hook/master/scripts/install.sh | sh
git init
Any
, it WILL NOT be acceptedFAQs
A library that allows the editing of content control XML components in .docx files.
We found that docx-form demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.