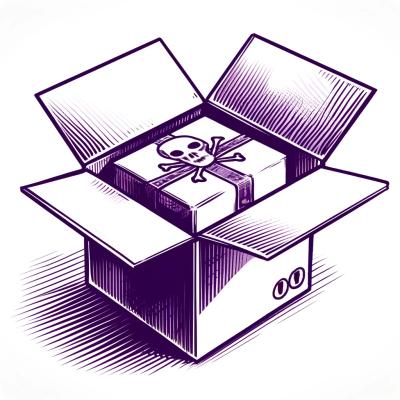
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
High-Performance Linear/Non-Linear Filters and Window Functions Library in C++ with Python Bindings
fastfilter is a powerful library written in C++ with Python bindings. It provides you with the capability to use commonly used window functions and non-linear filters that are utilized in digital signal processing. You can use it in both Python and C++.
Here are some key features of fastfilter
Python Bindings
Python
and use the filters and window functions seamlessly.Python
and C++
environments.Common Non-Linear Filters
Window Functions
Header-Only Implementation
Comprehensive Documentation
Using fastfilter
is intuitive and straightforward. Below are some examples to help you get started.
All classes come with a help section to guide you through their usage:
import medianFilter
help(medianFilter)
print(medianFilter.__version__)
You can easily apply various filters to your signal data. Here are some examples:
import numpy as np
import medianFilter as filt
signal = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
kernelSize = 5
output = filt.movingfilter(signal, kernelSize // 2, 'average')
print(output)
import numpy as np
import medianFilter as filt
signal = np.array([1, 12, 7, 8, 1, 16, 2, 18, 9, 21])
kernelSize = 5
output = filt.movingfilter(signal, kernelSize // 2, 'median')
print(output)
import numpy as np
import medianFilter as filt
signal = np.array([1, 12, 7, 8, 1, 16, 2, 18, 9, 21])
kernelSize = 5
# Apply maximum filter
output_max = filt.movingfilter(signal, kernelSize // 2, 'maximum')
print(output_max)
# Apply minimum filter
output_min = filt.movingfilter(signal, kernelSize // 2, 'minimum')
print(output_min)
You can also apply various window functions to your signal data. Here’s an example of using the Hamming window:
import numpy as np
import windowFunctions as wf
# It should be completed here
# windowFunctions is not binded yet.
Integrating fastfilter
into your C++ projects is simple.
You can effortlessly apply various filters to your signal data.
#include <iostream>
#include "medianFilter.h"
int main() {
std::vector<float> data {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Create output vector and variables
std::vector<float> output(data.size());
const uint32_t halfWindowSize = 2;
// Apply median filter
filt::movingFilter(output, data, halfWindowSize, filt::kernel::average);
// Display the output
for (const auto& val : output) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
#include <iostream>
#include "medianFilter.h"
int main() {
std::vector<float> data {1, 12, 7, 8, 1, 16, 2, 18, 9, 21};
// Create output vector and variables
std::vector<float> output(data.size());
const uint32_t halfWindowSize = 2;
// Apply median filter
filt::movingFilter(output, data, halfWindowSize, filt::kernel::median);
// Display the output
for (const auto& val : output) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
#include <iostream>
#include "medianFilter.h"
int main() {
std::vector<float> data {1, 12, 7, 8, 1, 16, 2, 18, 9, 21};
// Create output vector and variables
std::vector<float> output(data.size());
const uint32_t halfWindowSize = 2;
// Apply minimum filter
filt::movingFilter(output, data, halfWindowSize, filt::kernel::minimum);
// Display the output
std::cout << "Minimum Filter Output: ";
for (const auto& val : output) {
std::cout << val << " ";
}
std::cout << std::endl;
// Apply maximum filter
filt::movingFilter(output, data, halfWindowSize, filt::kernel::maximum);
// Display the output
std::cout << "Maximum Filter Output: ";
for (const auto& val : output) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
#include <iostream>
#include "windowing.h"
#include <vector>
int main(int argc, char** argv) {
std::vector<float> input = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
std::vector<float> output(input.size());
// Apply various window functions
window::windowFunction(output, input, input.size(), window::kernel::triangularWindow);
window::windowFunction(output, input, input.size(), window::kernel::hammingWindow);
window::windowFunction(output, input, input.size(), window::kernel::parzenWindow);
window::windowFunction(output, input, input.size(), window::kernel::hannWindow);
window::windowFunction(output, input, input.size(), window::kernel::blackmanWindow);
window::windowFunction(output, input, input.size(), window::kernel::triangularWindow);
return 0;
}
#include <iostream>
#include "windowing.h"
#include <vector>
int main(int argc, char** argv) {
std::vector<float> input = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
std::vector<float> output(input.size());
const float parameter = 0.3;
// Apply window functions with parameters
window::windowFunction(output, input, input.size(), parameter, window::kernel::gaussianWindow);
window::windowFunction(output, input, input.size(), parameter, window::kernel::tukeyWindow);
return 0;
}
These examples demonstrate how to use various window functions provided by the library. The first example shows basic window functions, while the second example includes window functions that require additional parameters.
FAQs
High-Performance Linear/Non-Linear Filters and Window Functions Library in C++ with Python Bindings
We found that fastfilter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.