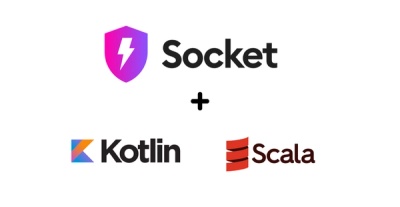
Product
Introducing Scala and Kotlin Support in Socket
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
feature-selection-toolkit
Advanced tools
A comprehensive toolkit for performing various feature selection techniques in machine learning.
The Feature Selection Toolkit is designed to simplify the process of selecting the most significant features from a dataset. By utilizing various feature selection methods, this toolkit aims to enhance model performance and reduce computational complexity. This comprehensive toolkit supports both classification and regression tasks, providing a range of methods to fit different scenarios.
To install the Feature Selection Toolkit, you can use pip:
pip install feature-selection-toolkit
First, initialize the FeatureSelection
class with your dataset:
from sklearn.datasets import load_iris
import pandas as pd
from feature_selection_toolkit import FeatureSelection
data = load_iris()
X = pd.DataFrame(data.data, columns=data.feature_names)
y = pd.Series(data.target)
fs = FeatureSelection(X, y)
Filter methods assess each feature independently to determine its relevance to the target variable.
The Chi-Squared test evaluates the independence between categorical features and the target variable. It's particularly useful for classification tasks where both the features and target are categorical.
scores, p_values = fs.filter_method(method='chi2')
print("Chi-Squared Scores:", scores)
print("p-values:", p_values)
Benefits:
Use Case: Ideal for datasets with categorical variables where the goal is to select features that are significantly associated with the target variable.
The ANOVA (Analysis of Variance) test assesses the difference between group means for continuous features relative to the target variable. It's suitable for classification tasks with continuous features.
scores, p_values = fs.filter_method(method='anova')
print("ANOVA Scores:", scores)
print("p-values:", p_values)
Benefits:
Use Case: Ideal for datasets with continuous features where the goal is to determine features that significantly differentiate between target classes.
Wrapper methods evaluate feature subsets using a specific model to iteratively select or remove features based on model performance.
Forward Selection starts with an empty set of features and adds one feature at a time based on the model performance until the addition of new features does not improve the model.
selected_features = fs.forward_selection(significance_level=0.05)
print("Selected Features using Forward Selection:", selected_features)
Benefits:
Use Case: Useful when you have a relatively small number of features and want to build a model by iteratively adding the most significant features.
Backward Elimination starts with all features and iteratively removes the least significant feature based on model performance until only significant features remain.
selected_features = fs.backward_elimination(significance_level=0.05)
print("Selected Features using Backward Elimination:", selected_features)
Benefits:
Use Case: Ideal for datasets with a large number of features, where the goal is to iteratively remove the least significant ones to improve model performance.
RFE removes the least important features iteratively based on a specified estimator until the desired number of features is reached.
support = fs.recursive_feature_elimination(estimator=RandomForestClassifier(), n_features_to_select=2)
print("RFE Support:", support)
Benefits:
Use Case: Suitable for situations where you want to rank features based on their importance and iteratively refine the feature set.
Embedded methods perform feature selection during the model training process.
Lasso (Least Absolute Shrinkage and Selection Operator) adds a penalty equal to the absolute value of the magnitude of coefficients, shrinking some coefficients to zero.
coefficients = fs.embedded_method(method='lasso', alpha=0.01)
print("Lasso Coefficients:", coefficients)
Benefits:
Use Case: Ideal for regression tasks with a large number of features where regularization and feature selection are required simultaneously.
Ridge Regression adds a penalty equal to the square of the magnitude of coefficients, shrinking coefficients but keeping all features.
coefficients = fs.embedded_method(method='ridge', alpha=0.01)
print("Ridge Coefficients:", coefficients)
Benefits:
Use Case: Suitable for regression tasks where overfitting is a concern, and you want to regularize without eliminating features.
Decision Trees provide feature importances inherently, which can be used for feature selection.
importances = fs.embedded_method(method='decision_tree')
print("Decision Tree Importances:", importances)
Benefits:
Use Case: Ideal for datasets where you want a quick and interpretable way to assess feature importance.
Random Forests aggregate the importance scores from multiple decision trees, providing a more robust feature importance measure.
importances = fs.embedded_method(method='random_forest')
print("Random Forest Importances:", importances)
Benefits:
Use Case: Useful when you want a robust and stable measure of feature importance from an ensemble of trees.
Evaluate all possible feature combinations to find the best performing subset. This method ensures the selection of the optimal feature set by trying every possible combination.
best_scores = fs.scored_columns(test_size=0.2, random_state=1, r_start_on=2)
print("Best Scores:", best_scores)
Benefits:
Use Case: Ideal for small to medium-sized datasets where computational resources allow for evaluating all feature combinations to find the best subset.
Combines the strengths of Recursive Feature Elimination and Brute Force Search to ensure the best feature set is selected by evaluating all possible subsets generated by RFE.
best_features = fs.rfe_brute_force(estimator=RandomForestClassifier(), n_features_to_select=5, force=True)
print("Best Features from RFE Brute Force:", best_features)
Benefits:
Use Case: Ideal for complex datasets where both feature importance and interactions need to be evaluated comprehensively to select the best feature subset.
The methods included in this toolkit are based on well-established statistical techniques and have been extensively validated in academic research. For instance:
Using the Iris dataset, the toolkit can help in selecting the most important features for classifying different species of flowers. Forward Selection, for instance, can iteratively add features to find the optimal subset that maximizes classification accuracy.
In a regression task like predicting housing prices, embedded methods like Lasso and Ridge can be used to handle high-dimensional data and identify key features that influence prices, leading to more accurate and interpretable models.
The Feature Selection Toolkit is an essential tool for data scientists and machine learning practitioners looking to improve their models' performance by selecting the most relevant features. With its comprehensive range of methods and user-friendly interface, it provides a robust solution for feature selection in various machine learning tasks.
We welcome contributions to the Feature Selection Toolkit. If you have ideas for new features, bug fixes, or improvements, please follow these steps:
The Feature Selection Toolkit is licensed under the MIT License. See the LICENSE file for more information.
FAQs
A comprehensive toolkit for performing various feature selection techniques in machine learning.
We found that feature-selection-toolkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.