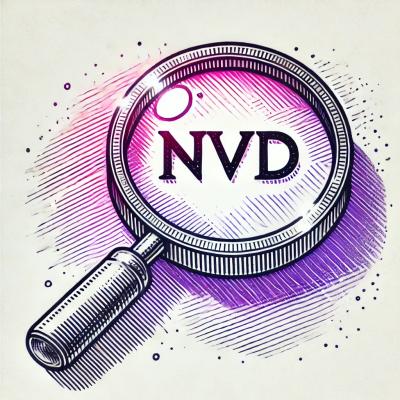
Security News
NIST Under Federal Audit for NVD Processing Backlog and Delays
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
A fluent design widgets library based on Flet
[!IMPORTANT] fluentflet is still in alpha version. this means that there could be bugs and unexpected behaviours
pip install fluentflet
git clone https://github.com/Bbalduzz/fluentflet.git
cd fluentflet
py setup.py install
fluentflet/components/button.py
class ButtonVariant(Enum):
DEFAULT = "default" # Standard button styling
ACCENT = "accent" # Primary action/emphasized styling
HYPERLINK = "hyperlink" # Text-like button styling
TOGGLE = "toggle" # Toggleable state button
Purpose: Implements Fluent Design System button control with multiple variants and styles.
Inherits from: ft.TextButton
is_toggled: bool = False
. Current toggle state for toggle variant buttons_variant: ButtonVariant
. Current button style variantdesign_system: FluentDesignSystem
. Reference to design system for stylingtheme: Theme
. Current theme settingscontent: Union[str, ft.Control] = None
. Button content (text or control like icon)
variant: ButtonVariant = ButtonVariant.DEFAULT
. Button style variantheight: int = 35
. Button height in pixelscustom_color: Optional[str] = None
. Optional color overridedesign_system: FluentDesignSystem = None
. Design system instanceis_dark_mode: bool = True
. Theme mode selection**kwargs
. Additional Flet button propertiesfrom fluentflet.components import Button, ButtonVariant
from fluentflet.utils import FluentIcon, FluentIcons
# Text button
basic_button = Button(
"Click Me",
variant=ButtonVariant.DEFAULT,
on_click=lambda e: print("Clicked!")
)
# Icon button with accent
icon_button = Button(
content=FluentIcon(FluentIcons.ADD),
variant=ButtonVariant.ACCENT
)
# Toggle button
toggle = Button(
"Toggle Me",
variant=ButtonVariant.TOGGLE,
on_click=lambda e: print(f"Toggled: {toggle.is_toggled}")
)
Path: fluentflet/components/checkbox.py
class CheckState(Enum):
UNCHECKED = "unchecked" # Box is empty
CHECKED = "checked" # Box has checkmark
INDETERMINATE = "indeterminate" # Box has dash (mixed state)
Purpose: Implements tri-state checkbox control with Fluent Design styling.
Inherits from: ft.Container
state: CheckState
. Current checkbox state_disabled: bool
. Disabled state trackingsize: int
. Size of the checkbox in pixelsthree_state: bool
. Whether indeterminate state is allowedstate: CheckState = CheckState.UNCHECKED
. Initial statelabel: str = ""
. Optional label textsize: int = 20
. Size in pixelson_change: Callable[[CheckState], None] = None
. State change callbackdisabled: bool = False
. Initial disabled statethree_state: bool = False
. Enable tri-state behaviordesign_system: FluentDesignSystem = None
. Design system instanceis_dark_mode: bool = True
. Theme mode**kwargs
. Additional container propertiesfrom fluentflet.components import Checkbox, CheckState
# Basic checkbox
checkbox = Checkbox(
label="Enable feature",
on_change=lambda state: print(f"State: {state}")
)
# Tri-state checkbox
tristate = Checkbox(
label="Select files",
three_state=True,
state=CheckState.INDETERMINATE
)
# Disabled checkbox
disabled = Checkbox(
label="Unavailable option",
disabled=True,
state=CheckState.CHECKED
)
fluentflet/components/dialog.py
Purpose: Implements a modal dialog overlay with Fluent Design styling.
Inherits from: ft.Container
dialog_width: int = 400
. Width of dialog in pixelstitle_text: str
. Dialog title_content: ft.Control
. Main dialog contentactions: List[Button]
. Action buttons for dialogtitle: str = "Dialog Title"
. Dialog header textcontent: Optional[ft.Control] = None
. Main content area
actions: Optional[List[Button]] = None
. Bottom action buttons
show()
: Display the dialog
Returns:
- None: Updates page overlay
close_dialog(e: Optional[ft.ControlEvent] = None)
: Hide and remove dialog
Returns:
- None: Removes from page overlay
from fluentflet.components import Dialog, Button, ButtonVariant
# Simple dialog
dialog = Dialog(
title="Confirm Action",
content=ft.Text("Are you sure you want to proceed?")
)
# Custom dialog with multiple actions
custom_dialog = Dialog(
title="Save Changes",
content=ft.Column([
ft.Text("Save current changes?"),
ft.TextField(label="Comment")
]),
actions=[
Button("Save", variant=ButtonVariant.ACCENT),
Button("Don't Save"),
Button("Cancel")
]
)
# Show dialog
page.overlay.append(dialog)
dialog.show()
fluentflet/components/dropdown.py
Purpose: Implements a dropdown select control with Fluent Design styling.
Inherits from: ft.Container
options: List[Union[str, ft.Control]]
. Available optionsmax_width: int
. Maximum width of dropdownselected_value: str
. Currently selected optionis_open: bool
. Dropdown expanded stateoptions: List[Union[str, ft.Control]]
. List of dropdown options
max_width: int = 150
. Maximum width in pixelstheme_mode: ft.ThemeMode = ft.ThemeMode.DARK
. Theme selectionon_select: Optional[Callable] = None
. Selection change callbackanimated: bool = True
. Enable animationsinitial_value: Optional[str] = None
. Initially selected item**kwargs
. Additional container propertiesfrom fluentflet.components import Dropdown
# Simple string options
dropdown = Dropdown(
options=["Option 1", "Option 2", "Option 3"],
on_select=lambda value: print(f"Selected: {value}")
)
# Custom control options
dropdown_with_icons = Dropdown(
options=[
ft.Row([
ft.Icon(ft.icons.SETTINGS),
ft.Text("Settings")
]),
ft.Row([
ft.Icon(ft.icons.PERSON),
ft.Text("Profile")
])
],
max_width=200
)
fluentflet/components/expander.py
Purpose: Implements collapsible/expandable section with Fluent Design styling.
Inherits from: ft.Container
_expanded: bool
. Current expansion state_width: int
. Width of expander_header: ft.Control
. Header content_content: ft.Control
. Main content_content_height: Optional[int]
. Cached content height for animationheader: Union[str, ft.Control]
. Content shown in always-visible header
content: ft.Control
. Content shown when expandedexpand: bool = False
. Initial expanded statewidth: int = 600
. Expander width in pixelsis_dark_mode: bool = True
. Theme mode**kwargs
. Additional container propertiesexpanded: bool
. Get/set expanded statefrom fluentflet.components import Expander
# Simple text expander
expander = Expander(
header="Click to Expand",
content=ft.Text("Expanded content here"),
expand=False
)
# Complex expander with custom header
expander = Expander(
header=ft.Row([
ft.Icon(ft.icons.SETTINGS),
ft.Text("Advanced Settings")
]),
content=ft.Column([
ft.TextField(label="Setting 1"),
ft.TextField(label="Setting 2")
])
)
fluentflet/components/listitem.py
Purpose: Implements selectable list item with Fluent Design styling.
Inherits from: ft.GestureDetector
instances: List[ListItem]
. All created instances for selection managementitem_content: ft.Control
. Item's contentis_hovered: bool
. Hover stateis_pressed: bool
. Press state_is_selected: bool
. Selection statecontent: ft.Control
. Item contenton_click: Optional[Callable] = None
. Click handleris_dark_mode: bool = True
. Theme modeselected: bool = False
. Initial selection state**kwargs
. Additional gesture detector propertiesselected: bool
. Get/set selection statefrom fluentflet.components import ListItem
# Simple list item
item = ListItem(
content=ft.Text("List Item 1"),
on_click=lambda _: print("Clicked")
)
# Complex list item
item = ListItem(
content=ft.Row([
ft.Icon(ft.icons.PERSON),
ft.Column([
ft.Text("John Doe"),
ft.Text("john@example.com", size=12)
])
]),
selected=True
)
# List of items
items_list = ft.ListView(
controls=[
ListItem(content=ft.Text(f"Item {i}"))
for i in range(5)
]
)
fluentflet/components/progressring.py
Purpose: Implements circular progress indicator with Fluent Design styling.
Inherits from: ft.ProgressRing
stroke_width: int = 3
. Width of the ring strokevalue: Optional[float] = None
. Progress value (0.0-1.0 for determinate, None for indeterminate)**kwargs
. Additional progress ring propertiesfrom fluentflet.components import ProgressRing
# Indeterminate progress
loading = ProgressRing()
# Determinate progress
progress = ProgressRing(value=0.75)
# Custom styled progress
custom = ProgressRing(
value=0.5,
stroke_width=5,
width=100,
height=100
)
fluentflet/components/radio.py
Purpose: Implements individual radio button control.
Inherits from: ft.Container
value: Any
. Value associated with radio button_selected: bool
. Current selection state_disabled: bool
. Disabled stateis_hovered: bool
. Hover stateis_pressed: bool
. Press state_radio_group: Optional[RadioGroup]
. Parent radio groupvalue: Any = None
. Value for this radio optionlabel: str = ""
. Label textselected: bool = False
. Initial selectiondisabled: bool = False
. Disabled statedesign_system: FluentDesignSystem = None
. Design system instanceis_dark_mode: bool = True
. Theme mode**kwargs
. Additional container propertiesPurpose: Container for managing a group of related radio buttons.
Inherits from: ft.Container
radios: List[Radio]
. Child radio buttons_value: Any
. Currently selected value_on_change: Optional[Callable]
. Value change callbackcontent: ft.Control = None
. Container contentvalue: Any = None
. Initial selected valueon_change: Optional[Callable] = None
. Value change handlerdesign_system: FluentDesignSystem = None
. Design system instanceis_dark_mode: bool = True
. Theme mode**kwargs
. Additional container propertiesfrom fluentflet.components import Radio, RadioGroup
# Single radio button
radio = Radio(
value="option1",
label="Option 1",
disabled=False
)
# Radio group
radio_group = RadioGroup(
content=ft.Column([
Radio(value="small", label="Small"),
Radio(value="medium", label="Medium"),
Radio(value="large", label="Large")
]),
value="medium",
on_change=lambda value: print(f"Selected: {value}")
)
fluentflet/components/slider.py
class SliderOrientation(Enum):
HORIZONTAL = "horizontal"
VERTICAL = "vertical"
Purpose: Implements a draggable slider control with Fluent Design styling.
Inherits from: ft.Container
current_value: float
. Current slider valuemin: float
. Minimum valuemax: float
. Maximum valuedragging: bool
. Current drag statethumb_size: int
. Size of slider thumbis_hovered: bool
. Hover stateis_pressed: bool
. Press statevalue: float = 0
. Initial value
min: float = 0
. Minimum valuemax: float = 100
. Maximum valueon_change: Optional[Callable[[float], None]] = None
. Value change callbacksize: int = 200
. Slider length in pixelsdisabled: bool = False
. Disabled stateorientation: SliderOrientation = SliderOrientation.HORIZONTAL
. Slider orientationis_dark_mode: bool = True
. Theme mode**kwargs
. Additional container propertiesfrom fluentflet.components import Slider, SliderOrientation
# Horizontal slider
slider = Slider(
value=50,
min=0,
max=100,
on_change=lambda e: print(f"Value: {e.current_value}")
)
# Vertical slider
vertical_slider = Slider(
value=0.5,
min=0,
max=1,
orientation=SliderOrientation.VERTICAL,
size=150
)
# Custom range slider
custom = Slider(
value=-50,
min=-100,
max=100,
disabled=False
)
fluentflet/components/textbox.py
Purpose: Implements text input control with Fluent Design styling.
Inherits from: ft.Container
textfield: ft.TextField
. Core text input controlbottom_border: ft.Container
. Bottom border with animationactions_row: ft.Row
. Container for action buttonsdefault_bgcolor: str
. Default background colordesign_system: FluentDesignSystem = FluentDesignSystem()
. Design system instanceplaceholder: str = "TextBox"
. Placeholder textprefix: str = None
: Optional text to display before the input areasuffix: str = None
: Optional text to display after the input areawidth: int = 200
. Control widthtext_size: int = 14
. Font sizeheight: int = 32
. Control heightpassword: bool = False
. Password input modeactions_visible: bool = True
. Show/hide action buttons**kwargs
. Additional container propertiesadd_action(icon: FluentIcons, on_click=None, tooltip: str = None) -> Button
from fluentflet.components import TextBox
from fluentflet.utils import FluentIcons
# Basic textbox
textbox = TextBox(
placeholder="Enter text",
width=300
)
# Password textbox
password = TextBox(
placeholder="Password",
password=True
)
# TextBox with prefix and suffix
url_input = TextBox(
placeholder="Enter domain name",
prefix="https://",
suffix=".com",
width=300
)
# Textbox with actions
textbox_with_actions = TextBox(
placeholder="Search",
width=400
)
textbox_with_actions.add_action(
icon=FluentIcons.SEARCH,
on_click=lambda _: print("Search clicked"),
tooltip="Search"
)
textbox_with_actions.add_action(
icon=FluentIcons.DISMISS,
on_click=lambda _: setattr(textbox_with_actions, 'value', ''),
tooltip="Clear"
)
fluentflet/components/toggle.py
Purpose: Implements a toggle switch with Fluent Design styling.
Inherits from: ft.Container
value: bool
. Current toggle stateon_content: str
. Label text for ON stateoff_content: str
. Label text for OFF state_label: str
. Current label text_handle_size: int
. Size of toggle handle_handle_expanded_width: int
. Width when handle is pressedvalue: bool = False
. Initial statelabel: Union[str, dict] = None
. Label text or dict with on/off states
label_position: ft.LabelPosition = ft.LabelPosition.RIGHT
. Label placementon_change: Optional[Callable[[bool], None]] = None
. State change callbacklabel_style: Optional[Dict] = None
. Label text stylingdisabled: bool = False
. Disabled statewidth: int = 40
. Toggle widthheight: int = 20
. Toggle height**kwargs
. Additional container propertiesfrom fluentflet.components import Toggle
# Simple toggle
toggle = Toggle(
value=False,
label="Enable feature",
on_change=lambda state: print(f"Toggled: {state}")
)
# Toggle with different labels
toggle = Toggle(
value=True,
label={
"on_content": "Enabled",
"off_content": "Disabled"
}
)
# Custom styled toggle
toggle = Toggle(
label="Custom toggle",
label_style={
"size": 16,
"weight": "bold"
},
width=50,
height=25
)
fluentflet/components/tooltip.py
Purpose: Implements hover tooltip with Fluent Design styling.
Inherits from: ft.Tooltip
padding: int = 6
. Tooltip paddingborder_radius: int = 4
. Corner radiustext_style: ft.TextStyle
. Tooltip text stylingbgcolor: str = "#2d2d2d"
. Background colorborder: ft.Border
. Border styleprefer_below: bool = False
. Show below target when possiblewait_duration: int = 300
. Delay before showing (ms)**kwargs
. Additional tooltip propertiesfrom fluentflet.components import ToolTip, Button
# Basic tooltip
button = Button("Hover me")
tooltip = ToolTip(
message="This is a tooltip",
content=button
)
# Custom styled tooltip
tooltip = ToolTip(
message="Custom tooltip",
content=ft.Text("Hover for info"),
text_style=ft.TextStyle(
size=14,
weight=ft.FontWeight.BOLD
),
bgcolor="#333333",
border_radius=8
)
fluentflet/components/treeview.py
Purpose: Base data structure for tree items.
id: str
. Unique identifier for the itemlabel: str
. Display textvalue: Optional[Any] = None
. Associated valueparent_id: Optional[str] = None
. ID of parent itemchildren: List[TreeItemData] = None
. Child itemsmetadata: Dict[str, Any] = None
. Additional item dataitem = TreeItemData(
id="root",
label="Root Item",
children=[
TreeItemData(id="child1", label="Child 1"),
TreeItemData(id="child2", label="Child 2")
]
)
Purpose: Abstract base class for tree data models.
Generic Type: T
- Type of raw data
items: List[TreeItemData]
. Processed tree items_raw_data: Optional[T]
. Source dataprocess_data()
: Convert raw data to TreeItemData objectsget_item_by_id(item_id: str) -> Optional[TreeItemData]
get_root_items() -> List[TreeItemData]
get_children(parent_id: str) -> List[TreeItemData]
Purpose: Tree model for dictionary data.
Inherits from: TreeViewAbstractModel[Dict]
data = {
"Root": {
"Child1": {
"GrandChild1": 1.1,
"GrandChild2": 1.2
},
"Child2": 2.0
}
}
model = DictTreeViewModel()
model.raw_data = data
Purpose: Tree model for JSON array/object data.
Inherits from: TreeViewAbstractModel[List[Dict]]
field_mapping: Optional[Dict[str, str]] = None
. Custom field name mapping
data = [
{
"id": "1",
"label": "Item 1",
"children": [
{
"id": "1.1",
"label": "Subitem 1.1"
}
]
}
]
model = JSONTreeViewModel()
model.raw_data = data
Purpose: Main tree view component with drag-drop support.
Inherits from: ft.Column
data: dict
. Source datamodel: Optional[TreeViewAbstractModel] = DictTreeViewModel()
. Data modelon_right_click: Optional[Callable] = None
. Right-click handleris_dark_mode: bool = True
. Theme modehandle_item_drop(src_id: str, target_id: str)
: Handle item reorderingfrom fluentflet.components import TreeView, DictTreeViewModel
# Basic tree
data = {
"Root": {
"Item1": 1.0,
"Item2": {
"SubItem1": 2.1,
"SubItem2": 2.2
}
}
}
tree = TreeView(
data=data,
model=DictTreeViewModel(),
on_right_click=lambda item: print(f"Right clicked: {item.label}")
)
# Custom model tree
class MyModel(TreeViewAbstractModel[List[Dict]]):
def process_data(self):
for item in self.raw_data:
self.items.append(TreeItemData(
id=str(item["id"]),
label=item["name"],
value=item["data"]
))
tree = TreeView(
data=my_data,
model=MyModel()
)
fluentflet/components/toast.py
class ToastPosition(Enum):
TOP_LEFT = "top-left"
TOP_RIGHT = "top-right"
TOP_CENTER = "top-center"
BOTTOM_LEFT = "bottom-left"
BOTTOM_RIGHT = "bottom-right"
BOTTOM_CENTER = "bottom-center"
class ToastVariant(Enum):
SINGLE_LINE = "single-line" # Compact single line toast
MULTI_LINE = "multi-line" # Expanded multi-line toast
class ToastSeverity(Enum):
INFORMATIONAL = "informational" # Blue info style
SUCCESS = "success" # Green success style
WARNING = "warning" # Yellow warning style
CRITICAL = "critical" # Red error style
class ToastActionType(Enum):
NONE = "none" # No action button
HYPERLINK = "hyperlink" # Link style action
DEFAULT = "default" # Button style action
Purpose: Individual toast notification with Fluent Design styling.
Inherits from: ft.Container
title: Optional[str] = None
. Toast title textmessage: Optional[str] = None
. Toast message textseverity: ToastSeverity | str = ToastSeverity.INFORMATIONAL
. Toast stylevariant: ToastVariant | str = ToastVariant.SINGLE_LINE
. Layout styleaction_type: ToastActionType | str = ToastActionType.NONE
. Action button typeaction_text: Optional[str] = None
. Action button textaction_url: Optional[str] = None
. URL for hyperlink actionon_action: Optional[Callable] = None
. Action click handlerposition: ToastPosition | str = ToastPosition.TOP_RIGHT
. Toast position**kwargs
. Additional container propertiesPurpose: Toast notification manager for showing/hiding toasts.
page: ft.Page
. Flet page instanceexpand: bool = False
. Auto-expand toasts on hoverposition: ToastPosition | str = ToastPosition.TOP_RIGHT
. Default positiontheme: str = "dark"
. Theme modedefault_toast_duration: int = 3
. Default show duration in secondsdefault_offset: int = 20
. Spacing from window edgedef show_toast(
self,
title: Optional[str] = None,
message: Optional[str] = None,
severity: ToastSeverity | str = ToastSeverity.INFORMATIONAL,
variant: ToastVariant | str = ToastVariant.SINGLE_LINE,
action_type: ToastActionType | str = ToastActionType.NONE,
action_text: Optional[str] = None,
action_url: Optional[str] = None,
on_action: Optional[Callable] = None,
position: Optional[ToastPosition | str] = None,
duration: int = 3,
toast: Optional[Toast] = None,
**kwargs
) -> None
from fluentflet.components import Toast, Toaster, ToastSeverity
def main(page: ft.Page):
# Create toaster
toaster = Toaster(
page=page,
position="top-right",
default_toast_duration=5
)
# Show simple toast
toaster.show_toast(
title="Success!",
message="Operation completed",
severity=ToastSeverity.SUCCESS
)
# Show toast with action
toaster.show_toast(
title="Warning",
message="Connection lost",
severity=ToastSeverity.WARNING,
action_type="default",
action_text="Retry",
on_action=lambda: print("Retrying...")
)
# Show custom toast
custom_toast = Toast(
title="Custom Toast",
message="With custom styling",
severity=ToastSeverity.INFORMATIONAL,
variant=ToastVariant.MULTI_LINE,
action_type=ToastActionType.HYPERLINK,
action_text="Learn More",
action_url="https://example.com"
)
toaster.show_toast(toast=custom_toast)
ft.app(target=main)
The Toast system provides:
fluentflet/utils/__init__.py
Purpose: Extends Flet's Page class with additional functionality for blur effects and drag-drop support.
blur_effect
@property
def blur_effect(self) -> bool:
"""Controls window blur effect on Windows"""
return self._blur
@blur_effect.setter
def blur_effect(self, value: bool):
# Only available on Windows
# Enables/disables acrylic blur effect
accepts_drops
@property
def accepts_drops(self) -> bool:
"""Controls file drop acceptance"""
return self._accepts_drops
@accepts_drops.setter
def accepts_drops(self, value: bool):
# Enables/disables file drop handling
def enable_drag_and_drop(
files_callback: Optional[Callable[[List[str]], None]] = None,
drag_enter_callback: Optional[Callable[[Tuple[int, int]], None]] = None,
drag_over_callback: Optional[Callable[[Tuple[int, int]], None]] = None,
drag_leave_callback: Optional[Callable[[], None]] = None
):
"""Enable file drag-drop functionality
Parameters:
- files_callback: Called with list of dropped file paths
- drag_enter_callback: Called with (x,y) when drag enters window
- drag_over_callback: Called with (x,y) while dragging over window
- drag_leave_callback: Called when drag leaves window
"""
Example Usage:
def on_files_dropped(files):
print("Files dropped:", files)
def on_drag_enter(point):
print(f"Drag entered at {point}")
page.accepts_drops = True
page.enable_drag_and_drop(
files_callback=on_files_dropped,
drag_enter_callback=on_drag_enter
)
fluentflet/window/fluent_window.py
Purpose: Implements a window manager with navigation, routing and state management following Fluent Design.
enum for controlling navigation layout:
class NavigationType(Enum):
STANDARD = auto() # Original layout that pushes content
OVERLAY = auto() # Navigation overlays the content
from fluentflet import FluentWindow, NavigationType
window = FluentWindow(
page=page,
nav_type=NavigationType.OVERLAY,
navigation_items=[
{"icon": FluentIcons.HOME, "label": "Home", "route": "/home"},
{"icon": FluentIcons.SETTINGS, "label": "Settings", "route": "/settings"}
]
)
Purpose: State management for FluentWindow applications
class FluentState:
"""Manages persistent and ephemeral application state"""
def set(self, key: str, value: any, persist: bool = False):
"""Set state value
Parameters:
- key: State key
- value: State value
- persist: If True, saves to session storage
"""
def get(self, key: str, default=None) -> any:
"""Get state value"""
def subscribe(self, key: str, callback: callable):
"""Subscribe to state changes"""
Example Usage:
class AppState(FluentState):
def _load_initial_state(self):
self._state = {
"theme": "light",
"selected_user": None
}
def set_theme(self, theme: str):
self.set("theme", theme, persist=True)
window.state = AppState()
window.state.subscribe("theme", lambda t: print(f"Theme changed: {t}"))
Purpose: Main window manager implementing navigation and routing
page: ft.Page
. Flet page instancenavigation_items: Optional[List[Dict]]
. Navigation menu items
[
{
"icon": FluentIcons.HOME, # Icon enum
"label": "Home", # Display text
"route": "/", # Optional route
}
]
nav_type: NavigationType = NavigationType.STANDARD
. Side navigation rail typebottom_navigation_items: Optional[List[Dict]]
. Bottom nav itemsselected_index: int = 0
. Initial selected nav itemwindow_titlebar: Union[str, Titlebar]
. Window title or titlebar componentcolors: Optional[Dict]
. Color overrides
{
"nav_bg": "#1F1F1F",
"content_bg": "#282828",
"title_bar_bg": "#1F1F1F",
"icon_color": "white",
"text_color": "white"
}
nav_width_collapsed: int = 50
. Navigation width when collapsednav_width_expanded: int = 200
. Navigation width when expandedanimation_duration: int = 100
. Animation duration in msshow_back_button: bool = True
. Show navigation back buttonstate_manager: Type[FluentState] = FluentState
. State manager classdef navigate(self, route: str, **params):
"""Navigate to route with optional parameters
Parameters:
- route: Route path
- **params: Route parameters
"""
@route("/path")
def view_builder():
"""Route decorator for registering views"""
return ft.Column([...])
def add_route(self, route: str, view_builder: Callable[..., ft.Control],
is_template: bool = False):
"""Register route manually"""
Example Usage:
def main(page: ft.Page):
window = FluentWindow(
page,
navigation_items=[
{"icon": FluentIcons.HOME, "label": "Home", "route": "/"},
{"icon": FluentIcons.PEOPLE, "label": "Users", "route": "/users"}
]
)
@window.route("/")
def home_view():
return ft.Column([
ft.Text("Welcome!", size=32),
Button("View Users",
on_click=lambda _: window.navigate("/users"))
])
@window.route("/users/:user_id", is_template=True)
def user_profile(user_id: str):
return ft.Column([
ft.Text(f"User Profile: {user_id}")
])
window.navigate("/")
ft.app(target=main)
FAQs
Fluent Design System components for Flet
We found that fluentflet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.
Security News
TypeScript Native Previews offers a 10x faster Go-based compiler, now available on npm for public testing with early editor and language support.