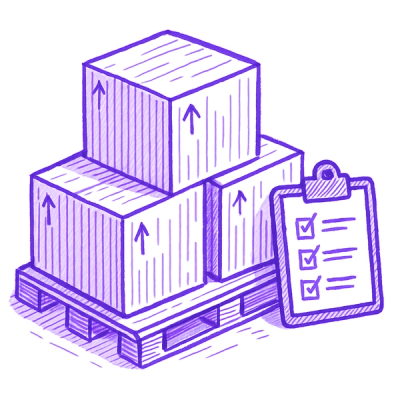
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
FunStrings is a comprehensive Python package that provides a wide range of functions for string manipulation, analysis, and transformation. It's designed to make working with strings easier and more efficient for developers, students, and educators.
FunStrings includes 44 utility functions organized into eight categories:
You can install FunStrings directly from PyPI:
pip install funstrings
Or install from source:
pip install git+https://github.com/nilkanth02/funstrings.git
import funstrings
# Basic operations
text = "Hello, World!"
print(funstrings.reverse_string(text)) # !dlroW ,olleH
print(funstrings.count_vowels(text)) # 3
# Text analysis
sentence = "The quick brown fox jumps over the lazy dog"
print(funstrings.is_pangram(sentence)) # True
print(funstrings.longest_word(sentence)) # quick
# Transformations
snake = "hello_world_example"
print(funstrings.snake_to_camel(snake)) # helloWorldExample
# Pattern-based
text_with_emails = "Contact us at info@example.com or support@example.org"
print(funstrings.extract_emails(text_with_emails)) # ['info@example.com', 'support@example.org']
# Data cleaning
html_text = "<p>Hello <b>World</b></p>"
print(funstrings.remove_html_tags(html_text)) # Hello World
print(funstrings.expand_contractions("I don't know")) # I do not know
# Text analysis helpers
print(funstrings.sentence_count("Hello! How are you? I'm fine.")) # 3
print(funstrings.most_common_word("hello world hello python")) # hello
# ML/NLP preprocessing
print(funstrings.generate_ngrams("hello", 2)) # ['he', 'el', 'll', 'lo']
print(funstrings.strip_accents("café")) # cafe
# Validation
print(funstrings.is_valid_email("user@example.com")) # True
print(funstrings.is_valid_url("https://example.com")) # True
For detailed documentation and examples, visit the GitHub repository.
FunStrings is designed to be educational and beginner-friendly. It includes:
tutorials/
directoryexamples/
directoryContributions are welcome! Here's how you can help:
git checkout -b feature-name
git commit -m 'Add some feature'
git push origin feature-name
Please make sure to update tests as appropriate.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
A Python package for string manipulation and analysis, and to play with strings
We found that funstrings demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.