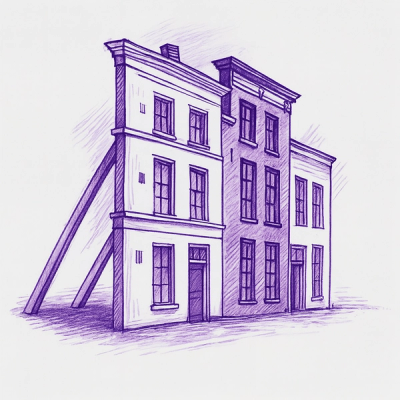
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
high-precision-timer
Advanced tools
A sub-microsecond-precise timer for timing and delaying code execution in python with/out blocking.
A library that offers sub-microsecond precise interval timing and blocking / non-blocking delay functionality for Python projects.
This library uses the 'chrono' C++ library to access the fastest available system clock and use it to provide interval timing and delay functionality via a Python binding API. While the performance of the timer heavily depends on the particular system configuration and utilization, most modern CPU should be capable of sub-microsecond precision using this timer. Additionally, the library offers a set of standalone utility functions that provide further date and time related services, such as time conversion and timestamp generation.
For users, all library dependencies are installed automatically when using the appropriate installation specification for all supported installation methods (see Installation section). For developers, see the Developers section for information on installing additional development dependencies.
Note. Building from source may require additional build-components to be available to compile the C++ portion of the library. It is highly advised to use the option to install from PIP or CONDA instead.
cd
to the root directory of the project using your CLI of choice.python -m pip install .
to install the project.benchmark_timer
command from your CLI (no need to use 'python'
directive). You can use the benchmark_timer --help
command to see the list of additional configuration
parameters that can be used to customize the benchmark.Use the following command to install the library using PIP:
pip install high-precision-timer
Use the following command to install the library using Conda or Mamba:
conda install high-precision-timer
This is a minimal example of how to use this library:
# First, import the timer class.
from high_precision_timer.precision_timer import PrecisionTimer
import time as tm
# Then, instantiate the timer class using the desired precision. Supported precisions are: 'ns' (nanoseconds),
# 'us' (microseconds), 'ms' (milliseconds), and 's' seconds.
timer = PrecisionTimer('us')
# Interval timing example
timer.reset() # Resets (re-bases) the timer
tm.sleep(1) # Simulates work (for 1 second)
print(f'Work time: {timer.elapsed} us') # This returns the 'work' duration using the precision units of the timer.
print() # Separates interval example from delay examples
# Delay example:
for i in range(10):
print(f'us delay iteration: {i}')
timer.delay_block(500) # Delays for 500 microseconds, does not release the GIL
print() # Separates the us loop from ms loop
timer.set_precision('ms') # Switches timer precision to milliseconds
for i in range(10):
print(f'ms delay iteration: {i}')
timer.delay_noblock(500) # Delays for 500 milliseconds, releases the GIL
See the API documentation for the detailed description of the methods and their arguments, exposed through the PrecisionTimer python class or the direct CPrecisionTimer binding class. The documentation also describes the benchmark method and the compatible CLI arguments used to control benchmark behavior and the standalone utility functions.
This section provides additional installation, dependency, and build-system instructions for the developers that want to modify the source code of this library. Additionally, it contains instructions for recreating the conda environments that were used during development from the included .yml files.
cd
to the root directory of the project using your CLI of choice.python -m pip install .'[dev]'
command to install development dependencies and the library. For some
systems, you may need to use a slightly modified version of this command: python -m pip install .[dev]
.
Alternatively, see the environments section for details on how to create a development environment
with all necessary dependencies, using a .yml or requirements.txt file.Note: When using tox automation, having a local version of the library may interfere with tox methods that attempt to build a library using an isolated environment. It is advised to remove the library from your test environment, or disconnect from the environment, prior to running any tox tasks.
In addition to installing the python packages, separately install the following dependencies:
To help developers, this project comes with a set of fully configured 'tox'-based pipelines for verifying and building the project. Each of the tox commands builds the project in an isolated environment before carrying out its task.
Below is a list of all available commands and their purpose:
tox -e lint
Checks and, where safe, fixes code formatting, style, and type-hinting.tox -e test
Builds the projects and executes the tests stored in the /tests directory using pytest-coverage
module.tox -e doxygen
Uses the externally installed Doxygen distribution to generate documentation from docstrings of
the C++ extension file.tox -e docs
Uses Sphinx to generate API documentation from Python Google-style docstrings. If Doxygen-generated
.xml files for the C++ extension are available, uses Breathe plugin to convert them to Sphinx-compatible format and
add
them to the final API .html file.tox --parallel
Carries out all commands listed above in-parallel (where possible). Remove the '--parallel'
argument to run the commands sequentially. Note, this command will build and test the library for all supported python
versions.tox -e build
Builds the binary wheels for the library for all architectures supported by the host machine.In addition to tox-based automation, all environments used during development are exported as .yml files and as spec.txt files to the envs folder. The environment snapshots were taken on each of the three supported OS families: Windows 11, OSx 14.5 and Ubuntu Cinnamon 24.04 LTS.
To install the development environment for your OS:
cd
into the envs folder.conda env create -f ENVNAME.yml
or mamba env create -f ENVNAME.yml
. Replace 'ENVNAME.yml' with the
name of the environment you want to install (hpt_dev_osx for OSx, hpt_dev_win64 for Windows and hpt_dev_lin64 for
Linux). Note, the OSx environment was built against M1 (Apple Silicon) platform and may not work on Intel-based Apple
devices.This project is licensed under the GPL3 License: see the LICENSE file for details.
FAQs
A sub-microsecond-precise timer for timing and delaying code execution in python with/out blocking.
We found that high-precision-timer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.