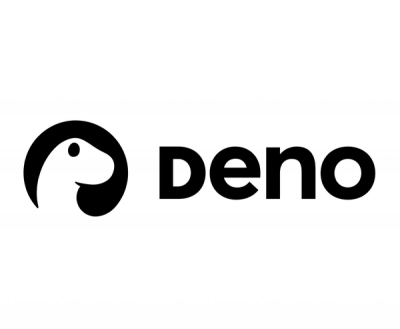
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Highly useful utilities for mocking execution flow during unit test execution.
Highly useful utilities for mocking execution flow during unit test execution.
pip install mocking-utils
from mocking_utils import MockFunction
class A(object):
def my_method(self):
print('I am in my_method')
a = A()
a.my_method() # Out: 'I am in my_method'
mock = MockFunction(A, 'my_method', lambda x: print('lambda function'), call=True)
a.my_method() # Out: 'lambda function'
mock.reset()
a.my_method() # Out: 'I am in my_method'
pytest
from mocking_utils import MockFunction
@pytest.fixture(scope='module', autouse=True)
def setup__teardown():
"""
Standard setup & teardown within a module of unit tests.
"""
mocks = [
MockFunction(A, 'my_method', lambda x: print('lambda function'), call=True)
]
yield 'Setup complete'
[mock.reset() for mock in mocks]
Code coverage reports for master, branches, and PRs are posted here in CodeCov.
FAQs
Highly useful utilities for mocking execution flow during unit test execution.
We found that mocking-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.