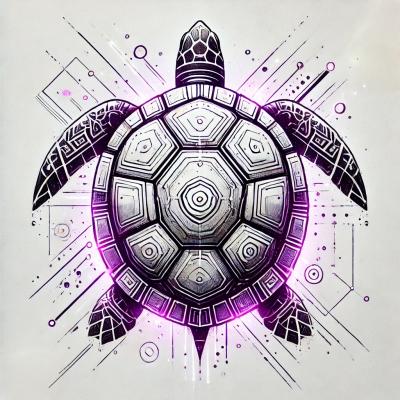
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Authenticate against OAuth2 Provider in Python CLIs
pip install oauth2-cli-auth
This should work for every provider supporting OIDC e.g. gitlab.com
:
from oauth2_cli_auth import get_access_token_with_browser_open, OAuth2ClientInfo
client_info = OAuth2ClientInfo.from_oidc_endpoint(
"https://gitlab.com/.well-known/openid-configuration",
client_id="my-client-id",
scopes=["openid"]
)
try:
token = get_access_token_with_browser_open(client_info)
print(f"Obtained token '{token}'")
except ValueError:
print("Failed to obtain token")
The following should work for almost all use cases, for rest please check the lib docs.
from oauth2_cli_auth import get_access_token_with_browser_open, OAuth2ClientInfo
client_info = OAuth2ClientInfo(
client_id="<clientId>",
authorization_url="<authorizeUrl>",
token_url="<TokenUrl>",
scopes=["scopeA", "scopeB"]
)
try:
token = get_access_token_with_browser_open(client_info)
print(f"Obtained token '{token}'")
except ValueError:
print("Failed to obtain token")
Building oauth2 integration for Python apps come quite handy, especially with Gitlab integration etc.
It is a bit cumbersome to do it manually everytime, existing solutions are way to overkill to put on this problem. So I created this small library without any dependencies besides the python standard library.
I love your input! I want to make contributing to this project as easy and transparent as possible, whether it's:
To get started please read the Contribution Guidelines.
poetry run pytest .
poetry install
FAQs
Authenticate against OAuth2 Provider in Python CLIs
We found that oauth2-cli-auth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.