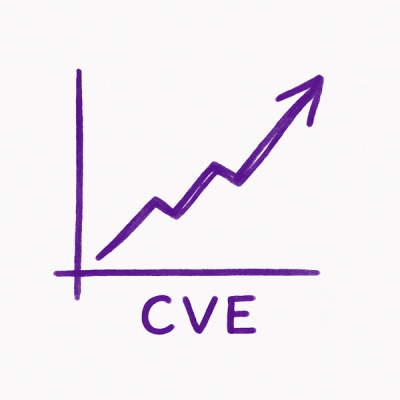
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
A package that allows developers to create popup messages to aid in debugging and add some spice into their coding process.
PopMessage is a Python package that enhances your development process with customizable pop-up messages. It allows you to display reminders, debug feedback, and fun surprises through pop-ups with random colors and sounds. With timer functionality, you can set delays for reminders, while error and debug pop-ups provide real-time feedback on your code. Whether you need a moment of wholesome encouragement or a playful surprise, PopMessage adds a unique touch to your coding experience.
PopMessage on PyPI broken link for now
Please make sure you have installed
In your active virtual environment, you can download and install the latest version of our package from PyPI by running the command:
pipenv install popmessage
You also need to install the Kivy library to use our package. Run the command:
pipenv install "kivy[base]"
from popmessage.popmsg import PopupMessage
To clone the repository, please open terminal or command prompt on your system and run:
git clone https://github.com/software-students-spring2025/3-python-package-push-then-pull.git
cd 3-python-package-push-then-pull
We recommend you use a virtual environment when running PopMessage. To set up your virtual environment and install dependencies, run these commands in your terminal or command prompt when you are in the cloned repository:
pipenv lock
pipenv install -e .
Once you make sure all dependencies have been installed, please execute the following command to run PopMessage:
pipenv run python -m popmessage.popmsg
Please note executing this command won't do anything as no functions from the package will be called.
To see examples of pop-up messages using PopMessage's functions, please execute the following command:
pipenv run python demo.py
You can easily import PopMessage into your Python project and start using the functions right away. Here's how you can use the package:
PopupMessage()
Constructs an instance of the PopupMessage
class which represents a pop-up message window. It initializes instance variables with default properties for the pop-up message window. To display a popup message window, you must first create an instance of PopupMessage
class by calling this constructor.
Upon instantiation, each PopupMessage object has the following properties with default values:
message
: The message to be displayed in the pop-up window. Defaults to "Default Message"bgColor
: The background color in the pop-up window. Defaults to "white".fontColor
: The text color of the displayed message. Defaults to "black".fontSize
: The font size of the displayed message. Defaults to 75.timerDuration
: The number of seconds before the pop-up window gets displayed. Defaults to 0.Parameters: None
Return: None
displayPopup(self, msg=None, bgColor=None, fontColor=None, fontSize=None)
This method displays the pop-up message window with customized properties defined through the parameters. If any of the parameters are not specified, it will default the property to the last configured value.
Parameters:
msg
(str, optional): The message to be displayed in the pop-up window. Defaults to the last configured value.bgColor
(str or tuple, optional): The background color in the pop-up window. Defaults to the last configured value.fontColor
(str or tuple, optional): The text color of the displayed message. Defaults to the last configured value.fontSize
(int, optional): The font size of the displayed message. Defaults to the last configured value.timerSeconds
(int, optional): The amount of time, in seconds, to wait before the popup will be shown. Defaults to a random integer between 1 and 60.Return: None
displayTimerPopup(self, msg=None, bgColor=None, fontColor=None, fontSize=None, timerSeconds=None)
This method will display a popup message similar to the previous function, with customizable properties, but will schedule the popup to be executed with a delay. The timer can be used to schedule a popup reminder, or to keep you on your toes when developing. If the timer duration is not specified, the function will randomize a timer duration of up to 60 seconds. If any of the other parameters are not specified, they will default to the last configured value.
Parameters:
msg
(str, optional): The message to be displayed in the pop-up window. Defaults to the last configured value.bgColor
(str or tuple, optional): The background color in the pop-up window. Defaults to the last configured value.fontColor
(str or tuple, optional): The text color of the displayed message. Defaults to the last configured value.fontSize
(int, optional): The font size of the displayed message. Defaults to the last configured value.Return: None
displaySFPopup(self, code_to_execute)
This method displays a success or error message depending on the status of your code. If the code runs successfully, a nice comment will be displayed. If the code runs unsuccessfully (throws an exception), a derogatory comment will be displayed.
Parameters:
code_to_execute
: A lambda functionReturn: None
displayRandomPopup(self)
This method displays a pop-up message window with randomly chosen properties. A random message will be displayed and a random sound will be played when this method is called.
Parameters: None
Return: None
You can create a basic pop-up message window by creating an instance of PopupMessage class first and then call displayPopup() with no parameters specified.
# Example1: Create and display a pop-up message window with default properties
myPopup1 = PopupMessage()
myPopup1.displayPopup()
To customize a basic pop-up message window, you can invoke displayPopup() method on an existing instance of PopupMessage and specify the properties you want to customize through its parameters.
# Example2: Create and display a pop-up message window with customized properties
myPopup2 = PopupMessage()
myPopup2.displayPopup(msg="Hello World", bgColor="blue", fontSize=75)
You can create a delayed popup by invoking the displayTimerPopup() function. Parameters may be specified if desired. If no delay is specified, the timer duration will be randomized.
Note: Be sure to save the instance of PopupMessage() in a variable before invoking this method. If not, unpredictable behavior will occur and the timed function may not be called.
#Example3 timer window
myPopup3 = PopupMessage()
myPopup3.displayTimerPopup(msg="Surprise!", bgColor="pink", timerSeconds=5)
myPopup3 = PopupMessage()
myPopup3.displayTimerPopup(msg="Randomly generated wait time ...", bgColor=(1, .769, 0, 1))
#Example4 Create and display a debug success/error message test
myPopup4 = PopupMessage()
myPopup4.displaySFPopup(lambda: print("Code ran successfully!"))
myPopup4.displaySFPopup(lambda: 5 + 5)
myPopup4.displaySFPopup(lambda: print(hello))
myPopup4.displaySFPopup(lambda: 1 / 0)
You can create a random pop-up message window by creating an instance of PopupMessage class first and then call displayRandomPopup() with no parameters specified.
# Example5: Create and display a random pop-up message window with randomized properties
myPopup5 = PopupMessage()
myPopup5.displayRandomPopup()
Link to a sample program: demo.py
Note: Due Kivy library's limitation, although multiple windows are created in this program, only one pop-up window is displayed at a time. The user must close the existing pop-up window displayed to view the next pop-up window.
We welcome contributions! If you'd like to contribute to our package, here's how to set up your development environment:
git clone https://github.com/your-username/3-python-package-push-then-pull.git
pipenv
. Regenerate the Pipfile.lock file for your machine specifications.pipenv lock
pipenv install -e .
src/popmessage
directory. The main code of our module is located in src/popmessage/popmsg.py
file.popmessage
packagetest_popmsg.py
, located in the tests
directory. You can add more unit test cases in this file.python -m pytest
pipenv
virtual environment, run: pipenv run python -m pytest
popmessage
packageBuild the project from the root directory where the pyproject.toml
file is located.
python -m build
pipenv
virtual environment, run: pipenv run python -m build
Verify that the dist
directory now contains a wheel and a zipfile with the correct version number in the filename.
For your development testing purposes, you can follow this tutorial to use twine to upload the package with your latest changes to TestPyPI.
Note: The popmessage
package has been manually tested with Python 3.10, 3.12, and 3.13 on MacOS, Windows, and Ubuntu. Because this package relies on the Kivy library which requires graphical libraries available on the local OS, if you experience any compatibility issues on your local machine, please refer to Kivy official website.
Click Here to view the license.
FAQs
A package that allows developers to create popup messages to aid in debugging and add some spice into their coding process.
We found that popmessage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.