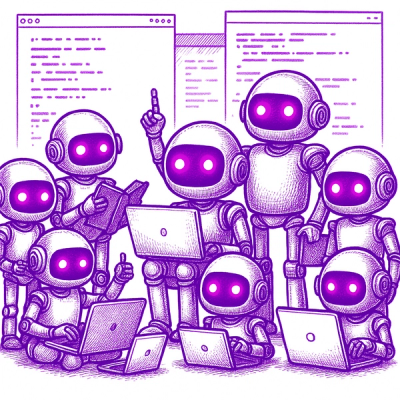
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
A library for enhanced JSON file handling with support for datetime and UUID objects, both synchronously and asynchronously.
Overview • Features • Installation • Usage • License • Contributing
JSONKit is a Python Library for enhanced JSON file handling with support for datetime
and UUID
objects. It provides both synchronous
and asynchronous
file operations.
Synchronous Operations: Read and write JSON files with custom encoding and decoding for datetime
and UUID
objects.
Asynchronous Operations: Handle JSON files asynchronously using aiofiles
.
Custom Serialization: Special handling for datetime
and UUID
types.
Pretty Printing: Pretty printing powered by the rich library!
You can install JSONKit via pip:
pip install py-jsonkit
You can load a JSON file into a Python object easily:
from jsonkit import JSONFile
# Initialize the JSONFile object
json_file = JSONFile('example.json')
# Load data from the file
data = json_file.load()
You can also write some data to a JSONFile easily
from jsonkit import JSONFile
from datetime import datetime
import uuid
# Initialize the JSONFile object
json_file = JSONFile('example.json')
# Example data
data_to_write = {
"name": "Alice",
"age": 30,
"created_at": datetime.now(),
"uid": uuid.uuid4()
}
# Insert to the JSON file
# JSONKit comes with custom encoding/decoding so you don't need to worry about parsing datetime or uuid objects!
# The `write` function returns the new data that is present in the JSON file after writing to it automatically, so you can store it in a variable like this:
new_returned_data = json_file.write(data_to_write, indent=4)
# Or, you can simply do this in the good old way:
json_file.write(data_to_write, indent=4)
new_data = json_file.load()
# NOTE: The method shown above will completely replace the old data with the new data. In case you want to append some data to a JSON file which of course, already contains some data, you can simply load that data first:
data = json_file.load()
# Then add some data to it:
data["......"] = "......"
data["woah this is some new data"] = "I am the value of the new data!"
# Then write this data back to the JSON File
json_file.write(data, indent=4)
JSONKit also comes with pretty printing which is handled by the rich library!
from jsonkit import JSONFile
# Intialize the JSONFile object
json_file = JSONFile('example.json')
# Pretty print all of the data in 'example.json' file to the console
# This will print the data with syntax hughlighting!
json_file.print_data()
JSONKit can also be used asynchronously using the AsyncJSONFile
class!
from jsonkit import AsyncJSONFile
import asyncio
# Create an instance of AsyncJSONFile
async_json_file = AsyncJSONFile('example.json')
# All of the methods that are available in 'JSONFile' class are also available here!
async def main():
# Loading data
data = await async_json_file.load()
# Writing data
data_to_insert = {
"...": "..."
}
await async_json_file.write(data_to_insert, indent=4)
# Pretty printing data
await async_json_file.print_data()
# Running the main function
asyncio.run(main())
JSONKit is licensed under the MIT License. See the LICENSE file for more details.
Contributions are welcome! Please open an issue or submit a pull request.
FAQs
A library for enhanced JSON file handling with support for datetime and UUID objects, both synchronously and asynchronously.
We found that py-jsonkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.