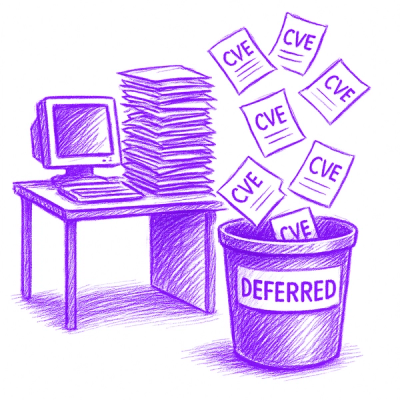
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
A Python package with simple implementation of a singly linked list with various utility functions.
A simple implementation of a singly linked list with various utility functions.
You can install the package via pip:
pip install singly_linked_list
Here’s a quick example of how to use the SinglyLinkList class:
from singly_linked_list import SinglyLinkList
# Create a new linked list
linked_list = SinglyLinkList()
# Add elements
linked_list.push(10) # Add to front
linked_list.append(20) # Add to end
linked_list.insert(10, 15) # Insert after 10
# Print the list
print(linked_list.printList()) # Output: [15, 10, 20]
# Remove an element
linked_list.remove(10)
print(linked_list.printList()) # Output: [15, 20]
The SinglyLinkList class provides methods for manipulating linked lists as described in the features section. Each method has its own functionality:
Initialization: SinglyLinkList()
Add Nodes:
push(data):
Add a new node at the beginning.append(data):
Add a new node at the end.insert(after_element, data):
Insert a new node after a specified element.Remove Nodes:
remove(element):
Remove the first occurrence of an element.delete():
Delete the entire linked list.Traversal and Querying:
printList():
Return a list of node values.length():
Return the number of nodes in the list.getNth(n):
Get the nth node's data.getNthFromLast(n):
Get the nth node's data from the end.Utilities:
isPalindrome():
Check if the list is a palindrome.checkLoop():
Check for loops in the list.lengthOfLoop():
Find the length of a loop if it exists.uniqueSorted():
Retrieve unique elements in sorted order.Modify List:
reverseList():
Reverse the order of nodes.segregateEvenOdd():
Separate even and odd nodes.Merging Lists:
mergeTwoLists(l1, l2):
Merge two sorted linked lists.Contributions are welcome! Please open an issue or submit a pull request on GitHub.
This project is licensed under the MIT License.
FAQs
A Python package with simple implementation of a singly linked list with various utility functions.
We found that py-linked-list demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.