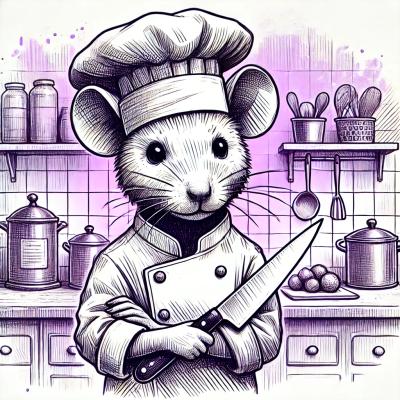
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Create default user avatars from a given string
This package creates simple user avatars that can be used in web-applications.
Avatars are generated from the first letter of a given string input.
Pyavatar is on Pypi so all you need is:
pip install pyavatar
Generate an avatar
>>> from pyavatar import PyAvatar
>>>
>>> avatar = PyAvatar("smallwat3r", size=250) # use a specific size
>>> avatar = PyAvatar("smallwat3r", capitalize=False) # without capitalization
>>> avatar = PyAvatar("smallwat3r", color=(40, 176, 200)) # use a specific color
>>> avatar = PyAvatar("smallwat3r", fontpath="/Users/me/fonts/myfont.ttf") # use a specific font
Change the avatar color
>>> avatar.color
(191, 91, 81)
>>> avatar.change_color() # random color
>>> avatar.color
(203, 22, 126)
>>> avatar.change_color("#28b0c8") # using an hex color code
>>> avatar.color
'#28b0c8'
Save the avatar as a base64 image
>>> image = avatar.base64_image("jpeg")
'data:image/jpeg;base64,/9j/4AAQSkZJRgABAQAAAQABAAD/2wBDAAgGBg ...'
You can then render it in an html tag with Jinja or another template engine
<img src="{{ image }}" alt="My avatar" />
Or save it as a bytes array
>>> avatar.stream("png")
b'\x89PNG\r\n\x1a\n\x00\x00\x00\rIHDR\x00\x00\x00\xfa\x00\x00 ...'
Or save it as a file locally
>>> import os
>>> avatar.save(f"{os.getcwd()}/me.png")
Make sure you're using a version of Python >= 3.10
make venv deps
Unit tests
make tests
Mypy
make mypy
Ruff
make ruff
Run all the above
make ci
We're using YAPF to format the code
make yapf
make release
FAQs
Generate simple user avatars from an input string.
We found that pyavatar demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.