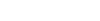
Anvil Encryption

This library is a small wrapper around the pypa/cryptography
library.
It offers convenience methods for encrypting and decrypting arbitrary payloads in both AES and RSA.
For use encrypting / decrypting payloads in Anvil, you can generate an RSA keypair from
your organization's settings page.
Anvil provides easy APIs for all things paperwork.
- PDF filling API - fill out a PDF template with a web request
and structured JSON data.
- PDF generation API - send markdown or HTML and Anvil will
render it to a PDF.
- Etch E-sign with API - customizable, embeddable, e-signature platform with
an API to control the signing process end-to-end.
- Anvil Workflows (w/ API) - Webforms + PDF + E-sign with a powerful
no-code builder. Easily collect structured data, generate PDFs, and request signatures.
Learn more about Anvil on our Anvil developer page.
Setup
Requirements
Installation
Install it directly into an activated virtual environment:
$ pip install python-anvil-encryption
or add it to your Poetry project:
$ poetry add python-anvil-encryption
Usage
This usage example is also in a runnable form in the examples/
directory.
import os
from python_anvil_encryption import encryption
CURRENT_PATH = os.path.dirname(os.path.realpath(__file__))
public_key = None
private_key = None
with open(os.path.join(CURRENT_PATH, "keys/public.pub"), "rb") as pub_file:
public_key = pub_file.read()
with open(os.path.join(CURRENT_PATH, "./keys/private.pem"), "rb") as priv_file:
private_key = priv_file.read()
message = b"Super secret message"
encrypted_message = encryption.encrypt_rsa(public_key, message)
decrypted_message = encryption.decrypt_rsa(private_key, encrypted_message)
assert decrypted_message == message
print(f"Are equal? {decrypted_message == message}")
aes_key = encryption.generate_aes_key()
aes_encrypted_message = encryption.encrypt_aes(aes_key, message)
decrypted_message = encryption.decrypt_aes(
aes_key.hex().encode(),
aes_encrypted_message
)
assert decrypted_message == message
print(f"Are equal? {decrypted_message == message}")