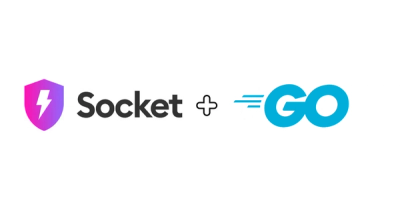
Product
Go Support Is Now Generally Available
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.
Install from PyPi, as ravendb.
pip install ravendb
Python client API (v7.0) for RavenDB , a NoSQL document database.
Type-hinted entire project and API results - using the API is now much more comfortable with IntelliSense
Subscriptions
Querying
Major bugfixes
Spatial querying and indexing
Highlighting fixes
Custom document parsers & loaders
New features
Improvements
Lazy Operations
Structure
ravendb
module...and many bugfixes
Simpler, well type hinted querying
Group by, aggregations
Spatial querying
Boost, fuzzy, proximity
Subclauses support
Store fields, index fields, pick analyzers & more using AbstractIndexCreationTask
Full indexes CRUD
Index related commands (priority, erros, start/stop, pause, lock)
Additional assemblies, map-reduce, index query with results "of_type"
Attachments
HTTPS
Lazy load
Cluster Transactions, Compare Exchange
from ravendb import DocumentStore
URLS = ["https://raven.server.url"]
DB_NAME = "SecuredDemo"
CERT_PATH = "path\\to\\cert.pem"
class User:
def __init__(self, name: str, tag: str):
self.name = name
self.tag = tag
store = DocumentStore(URLS, DB_NAME)
store.certificate_pem_path = CERT_PATH
store.initialize()
user = User("Gracjan", "Admin")
with store.open_session() as session:
session.store(user, "users/1")
session.save_changes()
with store.open_session() as session:
user = session.load("users/1", User)
assert user.name == "Gracjan"
assert user.tag == "Admin"
https://ravendb.net/docs/article-page/5.3/python
https://github.com/ravendb/ravendb-python-client
FAQs
Python client for RavenDB NoSQL Database
We found that ravendb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.
Security News
vlt adds real-time security selectors powered by Socket, enabling developers to query and analyze package risks directly in their dependency graph.
Security News
CISA extended MITRE’s CVE contract by 11 months, avoiding a shutdown but leaving long-term governance and coordination issues unresolved.