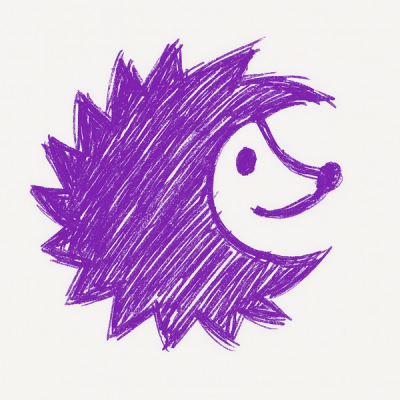
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
What it says on the tin - this generates random text slugs in Rust.
PyPi
Usable as a standalone binary, web applications as a WebAssembly module (WASM), or even as a Python module.
I needed a way to generate random slugs for a web project so thought it was a good opporunity to try out Rust's WebAssembly capabilities while also being able to use the same code as a zero-dependency python module for other projects.
pip install rustyrs
OR
python -m venv venv
source venv/bin/activate
pip install maturin
maturin develop --features python
Then from Python:
from rustyrs import random_slugs
slugs: list[str] = random_slugs(3, 5)
# slugs: ['reflecting-unsealed-mamba', 'disabling-addicting-asp', 'pliable-begotten-barnacle', 'vaulting-telepathic-caracal', 'canonical-graven-beetle']
Other features:
get_slug(word_length: int) -> str
: Generate a single slug of a specific lengthSlugGenerator(word_length: int)
: Create a generator object to generate slugs of a specific length. Will generate slugs until all unique permutations have been reached.
from rustyrs import SlugGenerator
gen = SlugGenerator(3)
print(next(gen)) # 'unwieldy-unsuspecting-ant'
combinations(word_length: int) -> int
: Get the number of possible combinations for a given word length
from rustyrs import combinations
print(combinations(2)) # 556,284
EternalSlugGenerator(word_length: int)
: Create iteration suffixed slugs forever. Guaranteed uniqueness.
from rustyrs import EternalSlugGenerator, combinations
gen = EternalSlugGenerator(2)
first = [next(gen) for _ in range(combinations(2))] # max no of combinations iterated
first[-1] # 'listening-tench-0'
next(gen) # 'existent-walrus-1'
time python -c "import rustyrs as r;a = set(r.random_slugs(2, 556_284));assert len(a) == 556_284"
real 0m0.219s
user 0m0.211s
sys 0m0.000s
time python -c "import rustyrs as r;a = set(r.random_slugs(5, 1_000_000));assert len(a) == 1_000_000"
real 0m0.667s
user 0m0.524s
sys 0m0.051s
# If wasm pack is not already installed
cargo install wasm-pack
# build the WASM module
wasm-pack build --target web --features wasm
Then from JS/TS:
import init, { random_slugs } from './pkg/rustyrs.js';
init();
const slugs: string[] = random_slugs(3, 5);
console.log(slugs);
// slugs: ['postpartum-regal-taipan', 'devastating-elven-salamander', 'immense-ambivalent-wren', 'philosophical-bandaged-gaur', 'outlaw-noncommercial-sunfish']
See index.html for a full example
cargo run --release [length in words] [number of slugs]
cargo build --release
[build path]/rustyrs [length in words] [number of slugs]
proctor-slimmer-guillemot
unsafe-warlike-avocado
garbled-pulled-stork
answerable-quick-whale
floral-apportioned-bobcat
MIT
FAQs
Generates unique slugs for various uses
We found that rustyrs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.