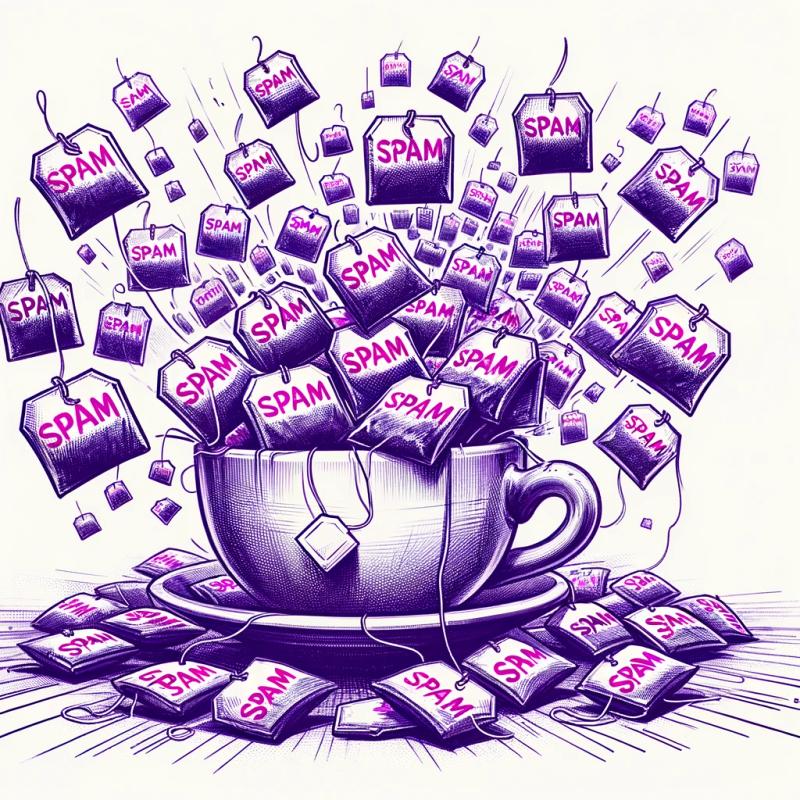
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
security-manager-apis
Readme
Developed using Python 3.8.0 and requests 2.20.1
This library/project is created to jumpstart your Orchestration API, Policy planner API, Security Manager API, or Policy Optimizer API projects.
Setup - PyPi Install:
pip install security-manager-apis
Setup - PyPi Upgrade:
To upgrade the library, run the following command from the terminal.
pip install --upgrade security-manager-apis
pip install -e .
If you don't plan to make any modifications to the project but still want to use it across your different projects, then do a local install.
pip install .
This will install all the dependencies listed in the setup.py
file. Once done
you can use the library wherever you want.
Pre-requisite - Python 3.6 or greater version should be installed on your machine.
Upgrade pip on Mac:
brew install python3
Upgrade pip on Windows:
python -m pip install --upgrade pip
Initializing a Policy Planner Class
from security_manager_apis import policy_planner
policyplan = policy_planner.PolicyPlannerApis(host: str, username: str, password: str, verify_ssl: bool, domain_id: str, workflow_name: str, suppress_ssl_warning: bool)
False
.True
.Create a Policy Planner Ticket
policyplan.create_pp_ticket(request_body: dict)
Request JSON Example:
{
"variables": {
"summary": "string",
"businessNeed": "string",
"priority": "string",
"dueDate": "YYYY-MM-DD HH:MM:SS",
"applicationName": "string",
"customer": "string",
"externalTicketId": "string",
"notes": "string",
"requesterName": "string",
"requesterEmail": "string",
"applicationOwner": "string",
"carbonCopy": [
"string",
"string"
]
},
"policyPlanRequirements": [
{
"sources": [
"string",
"string"
],
"destinations": [
"string",
"string"
],
"action": "string",
"services": [
"string",
"string"
],
"requirementType": "string",
"childKey": "string",
"variables": {}
}
]
}
Update a Policy Planner Ticket
policyplan.update_pp_ticket(ticket_id: str, request_body: dict)
Request JSON Example:
{
"variables": {
"summary": "string"
}
}
Querying for Policy Planner Tickets
policyplan.siql_query_pp_ticket(siql_query: str, page_size: int)
Retrieving a Policy Planner Ticket
policyplan.pull_pp_ticket(ticket_id: str)
Retrieving Policy Planner Ticket Event History
policyplan.pull_pp_ticket_events(ticket_id: str, page_size: int)
Retrieving Policy Planner Ticket Attachments
policyplan.pull_pp_ticket_attachements(ticket_id: str, page_size=100)
Download Policy Planner Ticket Attachments
policyplan.download_pp_ticket_attachment(self, ticket_id: str, attachment_id: str)
Coding Example:
attachment_resp = pp.download_pp_ticket_attachment(ticket_id, attachment_id)
file_name = attachment_resp.headers['filename']
open(file_name, 'wb').write(attachment_resp.content)
Assigning a Policy Planner Ticket
policyplan.assign_pp_ticket(ticket_id: str, user_id: str)
Unassigning a Policy Planner Ticket
policyplan.unassign_pp_ticket(ticket_id: str)
Adding a Requirement to a Policy Planner Ticket
policyplan.add_req_pp_ticket(ticket_id: str, req_json: dict)
Requirement JSON Example:
{
"requirements":[
{
"requirementType":"RULE",
"childKey":"add_access",
"variables":{
"expiration":"2022-01-01T00:00:00+0000"
},
"destinations":[
"10.1.1.1/24"
],
"services":[
"tcp/22"
],
"sources":[
"10.0.0.0/24"
],
"action":"ACCEPT"
}
]
}
Replacing Requirements on a Policy Planner Ticket
policyplan.replace_req_pp_ticket(self, ticket_id: str, req_json: dict)
Completing a Policy Planner Ticket Task
policyplan.complete_task_pp_ticket(ticket_id: str, button_action: str)
Running PCA for a Policy Planner Ticket
policyplan.run_pca(ticket_id: str, control_types: str, enable_risk_sa: str)
Adding Attachment to a Policy Planner Ticket
policyplan.add_attachment(ticket_id: str, file_name: str, f, description: str):
Adding Attachment Code Example:
file_name = "test_file.txt"
with open(file_name) as f:
policyplan.add_attachment('38', file_name, f, 'test upload')
Uploading Requirements via CSV to Policy Planner Ticket
policyplan.csv_req_upload(ticket_id: str, file_name: str, f, behavior="append"):
append
, pass replace
to replace all requirements on the ticket with the new CSV requirementsUploading Requirements via CSV Code Example:
file_name = "test_req.csv"
with open(file_name) as f:
policyplan.csv_req_upload('1', file_name, f)
Retrieving Requirements from a Policy Planner Ticket
policyplan.get_reqs(ticket_id: str)
Retrieving Changes from a Policy Planner Ticket
policyplan.get_changes(ticket_id: str)
Updating Change on a Policy Planner Ticket
policyplan.update_change(ticket_id: str, req_id: str, change_id: str, change_json: dict)
Deleting Requirements from a Policy Planner Ticket
policyplan.del_all_reqs(ticket_id: str)
Approving Requirement in a Policy Planner Ticket
policyplan.approve_req(ticket_id: str, req_id: str)
Add Comment to Policy Planner Ticket
policyplan.add_comment(ticket_id: str, comment: str)
Retrieve All Policy Planner Ticket Comments
policyplan.get_comments(ticket_id: str)
Delete Comment from Policy Planner Ticket
policyplan.del_comment(ticket_id: str, comment_id: str)
Ending a Policy Planner Session
policyplan.logout()
Initializing a Security Manager Class
from security_manager_apis import security_manager
securitymanager = security_manager.SecurityManagerApis(host: str, username: str, password: str, verify_ssl: bool, domain_id: str, suppress_ssl_warning: bool)
False
.True
.Get List of Devices in Security Manager
securitymanager.get_devices()
Manual Device Retrieval
securitymanager.manual_device_retrieval(device_id: str)
Create Device Group
securitymanager.create_device_group(device_group_name: str)
Get Device Group by Name
securitymanager.get_device_group_by_name(device_group_name: str)
Add Device to Device Group
securitymanager.add_to_device_group(device_group_id: str, device_id: str)
Adding a Supplemental Route
securitymanager.add_supp_route(device_id: str, supplemental_route: dict)
Supplemental Route JSON Example
{
"destination": "10.0.0.25",
"deviceId": "2",
"drop": false,
"gateway": "10.0.0.26",
"interfaceName": "port1",
"metric": 3
}
Bulk Adding Supplemental Route via Text File
securitymanager.bulk_add_supp_route(f)
Supplemental Route Text File Example
deviceId,interfaceName,destination,gateway,virtualRouter,nextVirtualRouter,metric,drop
2,port1,10.0.0.25,10.0.0.26,,,4,true
2,,10.0.0.25,10.0.0.26,Default,Default,4,true
Note: The first line of this file will not be processed, it serves as an informational header.
Supplemental Route Bulk Upload Code Example
with open('supp_route.txt') as f:
securitymanager.bulk_add_supp_route(f)
f.close()
Security Manager SIQL Query
securitymanager.siql_query(query_type: str, query: str, page_size: int)
Search for Device Zones
securitymanager.zone_search(device_id: str, page_size: int)
Retrieve Firewall Object
securitymanager.get_fw_obj(obj_type: str, device_id: str, match_id: str)
Retrieve Device Object
securitymanager.get_device_obj(device_id: str)
Retrieve Rule Documentation
securitymanager.get_rule_doc(device_id: str, rule_id: str)
Update Rule Documentation
securitymanager.update_rule_doc(device_id: str, rule_doc: dict)
Rule Doc JSON Example:
{
"ruleId":"16959bc0-b9f7-436b-9851-aac6f3d98963",
"deviceId":3,
"props":[
{
"ruleId":"16959bc0-b9f7-436b-9851-aac6f3d98963",
"ruleCustomPropertyDefinition":{
"id":1,
"customPropertyDefinition":{
"id":1,
"name":"Business Justification",
"key":"business_justification",
"type":"STRING_ARRAY",
"filterable":true,
"inheritFromMgmtStation":false
},
"name":"Business Justification",
"key":"business_justification",
"type":"STRING_ARRAY"
},
"customProperty":{
"id":1,
"name":"Business Justification",
"key":"business_justification",
"type":"STRING_ARRAY",
"filterable":true,
"inheritFromMgmtStation":false
},
"stringarray": ["test update"]
}
]
}
Ending a Security Manager Session
securitymanager.logout()
Initializing a Policy Optimizer Class
from security_manager_apis import policy_optimizer
policyoptimizer = policy_optimizer.PolicyOptimizerApis(host: str, username: str, password: str, verify_ssl: bool, domain_id: str, workflow_name: str, suppress_ssl_warning: bool)
False
.True
.Create a Policy Optimizer Ticket
policyoptimizer.create_pp_ticket(request_body: dict)
Request JSON Example:
{
"deviceId": 1,
"policyId": "62c7344a-31b9-40a6-8e7e-0c9cd6407fbe",
"ruleId": "16959bc0-b9f7-436b-9851-aac6f3d98963"
}
Retrieve Policy Optimizer Ticket JSON
policyoptimizer.get_po_ticket(ticket_id: str)
Assign Policy Optimizer Ticket to User
policyoptimizer.assign_po_ticket(ticket_id: str, user_id: str)
Complete a Policy Optimizer Ticket
policyoptimizer.complete_po_ticket(ticket_id: str, decision: dict)
Certify JSON Example:
{
"variables":{
"ruleDecision":"certify",
"certifyRemarks":"string",
"nextReviewDate":"2022-01-01T00:00:00-0500"
}
}
Decertify JSON Example:
{
"variables":{
"ruleDecision":"decertify",
"ruleActions":"string",
"modifyRuleOptions":"string",
"moveToPosition": "string",
"removeOther": "string",
"disableRuleOptions":"string",
"removeRuleOptions":"string",
"decertifyRuleReason":"string"
}
}
Decertify JSON Structure:
ruleActions
Options:
modifyRuleOptions
:
removeObjects
, which prompts a value for removeOther
moveToRulePosition
, which prompts a value for moveToPosition
modifyRuleOptions
, which prompts a value for other
disableRuleOptions
:
couldNotFindOwner
accessNoLongerNeeded
other
removeRuleOptions
:
accessNoLongerNeeded
accessIsTooRisky
other
Cancel a Policy Optimizer Ticket
policyoptimizer.cancel_po_ticket(ticket_id: str)
Query Policy Optimizer Tickets
policyoptimizer.siql_query_po_ticket(parameters: dict)
Params Example:
params = {'q': "review { workflow = 1 AND status ~ 'Review' }", 'pageSize': 20, 'domainId': 1, 'sortdir': 'asc'}
Ending a Policy Optimizer Session
policyoptimizer.logout()
Initializing an Orchestration API Class
from security_manager_apis import orchestration_apis
orchestration = orchestration_apis.OrchestrationApis(host: str, username: str, password: str, verify_ssl: bool, domain_id: str, suppress_ssl_warning=False)
False
.True
.Running Rule Recommendation
orchestration.rulerec_api(params: dict, req_json: dict)
Parameters Example
parameters = {'deviceGroupId': 1, 'addressMatchingStrategy': 'INTERSECTS', 'modifyBehavior': 'MODIFY', 'strategy': None}
Requirements Example
{
"requirements":[
{
"requirementType":"RULE",
"childKey":"add_access",
"variables":{
"expiration":"2022-01-01T00:00:00+0000"
},
"destinations":[
"10.1.1.1/24"
],
"services":[
"tcp/22"
],
"sources":[
"10.0.0.0/24"
],
"action":"ACCEPT"
}
]
}
Running Pre-Change Assessment
orchestration.pca_api(device_id: str, req_json: dict)
Requirements Example
{
"requirements":[
{
"requirementType":"RULE",
"childKey":"add_access",
"variables":{
"expiration":"2022-01-01T00:00:00+0000"
},
"destinations":[
"10.1.1.1/24"
],
"services":[
"tcp/22"
],
"sources":[
"10.0.0.0/24"
],
"action":"ACCEPT"
}
]
}
application.properties
- All the required URLS are placed here.get_properties_data.py
- Read the properties file data and returns a parserpolicy_planner.py
- Class to use Policy Planner APIssecurity_manager.py
- Class to use Security Manager APIspolicy_optimizer.py
- Class to use Policy Optimizer APIsorchestration_apis.py
- Class to use Crchestration APIsAs soon as you execute the command to run this library, Authentication class will be called which will internally call get_auth_token() of authentication_api.py
from authenticate_user
module only once and
auth token will be set in the headers.
Then we pass headers to the HTTP requests so that user should get authenticated and can access the endpoints safely.
MIT.
FAQs
Security Manager API
We found that security-manager-apis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.