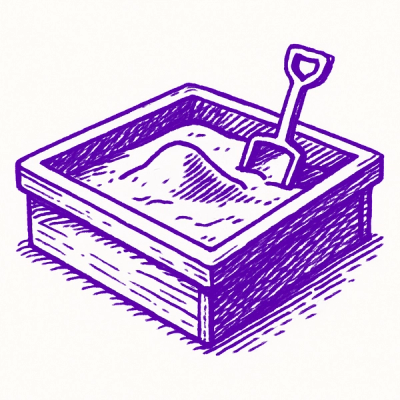
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
PostgresClient is a Python class designed to facilitate interactions with PostgreSQL databases.
To use PostgresClient, you'll first need to install the required dependencies:
pip install shipyard-postgresql
You can initialize a PostgresClient instance by providing the necessary connection parameters:
postgres = PostgresClient(
user='your_username',
pwd='your_password',
host='your_host_address',
port=5432, # default PostgreSQL port
database='your_database_name',
schema='your_schema_name' # optional, defaults to public
)
connect
Establish an active connection to postgres. If this is not explicity called beforehand, it will be handled implicitly when the first database call is sent
postgres.connect()
execute_query
Execute a SQL query on the database connection:
postgres.execute_query('drop table if exists public.demo_table')
upload
Upload data from a file to a PostgreSQL table:
file_path = 'path_to_your_file.csv'
table_name = 'your_table'
postgres.upload(file_path, table_name, insert_method = 'replace') # can also set to append
fetch
Fetch data from the database using a SQL query and return the results as a pandas dataframe:
df = postgres.fetch('select * from public.demo_table limit 1000')
close
Close the connection once finished
postgres.close()
FAQs
A local client for connecting and working with Postgresql Databases
We found that shipyard-postgresql demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.