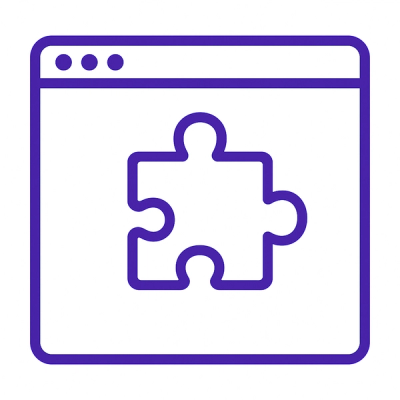
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
An Unofficial Asynchronous Python version of Adlinkfly and Alternative Website API wrapper
A Unofficial Wrapper for Adlinkfly Site and Alternative Sites
·
Report Bug / Request Feature
·
Usage
·
Reference
An Unofficial Python version of Adlinkfly and Alternative Website API wrapper. Used to Short your long link and let you earn from it.
Install shortzy with pip
pip install shortzy
To Upgrade
pip install --upgrade shortzy
from shortzy import Shortzy
import asyncio
shortzy = Shortzy('<YOUR API KEY>')
async def main():
link = await shortzy.convert('https://example.com/')
print(link)
asyncio.run(main())
Output: https://droplink.co/mVkra
Contributions are always welcome!
from shortzy import Shortzy
import asyncio
shortzy = Shortzy(api_key="Your API Key", base_site="droplink.co")
# Base site defaults to "droplink.co". You can add your own site here which is alternative to this default site
# Please Refer https://github.com/kevinnadar22/shortzy#available-websites for more information
convert(link, alias, silently_fail, quick_link) -> str
Parameter | Type | Description |
---|---|---|
link | string | Required. Long URL Link |
alias | string | Custom alias for the link |
silently_fail | bool | Raise an exception or not if error ocuurs |
quick_link | bool | Returns the quick link |
Example:
async def main():
link = await shortzy.convert('https://www.youtube.com/watch?v=d8RLHL3Lizw')
print(link)
asyncio.run(main())
## Output: https://droplink.co/Ly4fCxZ
## Quick Link: https://droplink.co/st?api=<YOUR API KEY>&url=https://www.youtube.com/watch?v=d8RLHL3Lizw
bulk_convert(urls:list, silently_fail, quick_link:bool=False) -> list
Parameter | Type | Description |
---|---|---|
urls | list | Required. List of URLs to convert |
Example:
async def main():
links = ['https://github.com/', 'https://twitter.com/', 'https://google.com/']
link = await shortzy.bulk_convert(links)
print(link)
asyncio.run(main())
## Output: ['https://droplink.co/ihu1e', 'https://droplink.co/AkY2Nt', 'https://droplink.co/mK1eVTV']
convert_from_text(text:str, silently_fail:bool=True, quick_link:bool=False) -> str
Parameter | Type | Description |
---|---|---|
text | str | Required. Text containing Long URLS to short |
Example:
async def main():
text = """
Unstoppable:-https://www.youtube.com/watch?v=330xlOv8p9M
Night Changes:-https://www.youtube.com/watch?v=syFZfO_wfMQ
"""
link = await shortzy.convert_from_text(text)
print(link)
asyncio.run(main())
# Output:
# "Unstoppable:-https://droplink.co/T6jbHlU
# Night Changes:-https://droplink.co/ajIRE"
get_quick_link(link:str)
Parameter | Type | Description |
---|---|---|
link | str | Required. Long Link |
Example:
async def main():
link = "https://www.youtube.com/watch?v=syFZfO_wfMQ"
quick_link = await shortzy.get_quick_link(link)
print(quick_link)
asyncio.run(main())
## Quick Link: https://droplink.co/st?api=<YOUR API KEY>&url=https://www.youtube.com/watch?v=syFZfO_wfMQ
For support, email jesikamaraj@gmail.com or PM Dev
Licensed under GNU AGPL v3.0.
Selling The Codes To Other People For Money Is Strictly Prohibited.
FAQs
An Unofficial Asynchronous Python version of Adlinkfly and Alternative Website API wrapper
We found that shortzy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.