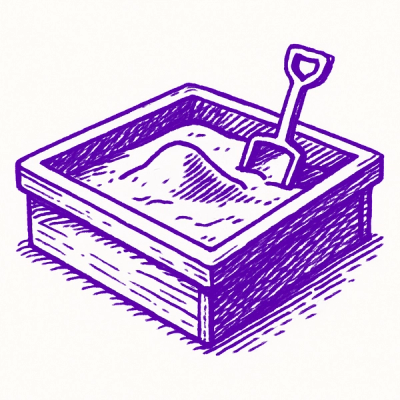
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
A comprehensive Python client for the Shotcut.in URL shortener API. This package provides an intuitive interface to interact with all Shotcut.in API endpoints.
Get Your API Key Here
pip install shotcut-python
from shotcut import ShotcutAPI
# Initialize the client
api = ShotcutAPI(api_key="your_api_key_here")
# Shorten a URL
response = api.shorten_link(
url="https://example.com",
custom="my-custom-alias"
)
print(response['shorturl'])
# Create a QR code
qr = api.create_qr_code(
type="link",
data="https://example.com",
background="rgb(255,255,255)",
foreground="rgb(0,0,0)"
)
print(qr['link'])
# Get account information
account = api.get_account()
# Update account details
api.update_account(
email="newemail@example.com",
password="newpassword123"
)
# Create a shortened URL
url = api.shorten_link(
url="https://example.com",
custom="my-link",
password="secret123",
expiry="2025-12-31",
domain="custom.com"
)
# List all URLs
urls = api.list_links(
limit=20,
page=1,
order="clicks"
)
# Get single URL details
url_details = api.get_link(link_id=123)
# Update URL
api.update_link(
link_id=123,
url="https://newexample.com",
password="newpassword123"
)
# Delete URL
api.delete_link(link_id=123)
# Create QR code
qr = api.create_qr_code(
type="link",
data="https://example.com",
background="rgb(255,255,255)",
foreground="rgb(0,0,0)",
logo="https://example.com/logo.png"
)
# List QR codes
qr_codes = api.list_qr_codes(limit=20, page=1)
# Get single QR code
qr_details = api.get_qr_code(qr_id=123)
# Update QR code
api.update_qr_code(
qr_id=123,
data="https://newexample.com",
background="rgb(0,0,255)"
)
# Delete QR code
api.delete_qr_code(qr_id=123)
# Create campaign
campaign = api.create_campaign(
name="Summer Sale 2025",
slug="summer-sale",
public=True
)
# List campaigns
campaigns = api.list_campaigns(limit=20, page=1)
# Assign link to campaign
api.assign_link_to_campaign(
campaign_id=123,
link_id=456
)
# Update campaign
api.update_campaign(
campaign_id=123,
name="Winter Sale 2025",
public=False
)
# Delete campaign
api.delete_campaign(campaign_id=123)
# List domains
domains = api.list_domains(limit=20, page=1)
# Create domain
domain = api.create_domain(
domain="short.example.com",
redirect_root="https://example.com",
redirect_404="https://example.com/404"
)
# Update domain
api.update_domain(
domain_id=123,
redirect_root="https://newexample.com"
)
# Delete domain
api.delete_domain(domain_id=123)
# Create channel
channel = api.create_channel(
name="Marketing",
description="Marketing campaign links",
color="rgb(255,0,0)",
starred=True
)
# List channels
channels = api.list_channels(limit=20, page=1)
# List channel items
items = api.list_channel_items(
channel_id=123,
limit=20,
page=1
)
# Update channel
api.update_channel(
channel_id=123,
name="Sales",
starred=False
)
# Delete channel
api.delete_channel(channel_id=123)
# Create pixel
pixel = api.create_pixel(
type="facebook",
name="FB Conversion Pixel",
tag="123456789"
)
# List pixels
pixels = api.list_pixels(limit=20, page=1)
# Update pixel
api.update_pixel(
pixel_id=123,
name="Updated FB Pixel",
tag="987654321"
)
# Delete pixel
api.delete_pixel(pixel_id=123)
# List splash pages
splash_pages = api.list_splash(limit=20, page=1)
# List overlays
overlays = api.list_overlays(limit=20, page=1)
from shotcut import ShotcutAPI, ShotcutAPIError
try:
api = ShotcutAPI(api_key="your_key")
response = api.create_link(url="https://example.com")
except ShotcutAPIError as e:
print(f"API Error: {str(e)}")
except RateLimitError as e:
print(f"Rate limit exceeded. Reset at {e.reset_time}")
except AuthenticationError as e:
print("Invalid API key")
except ValidationError as e:
print(f"Invalid data: {str(e)}")
To contribute to this project:
git clone https://github.com/Shotcut-Track/shotcutapi-python.git
pip install -e ".[dev]"
pytest
MIT License - see LICENSE file for details.
FAQs
Python client for the Shotcut.in URL shortener API
We found that shotcut-python demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.