spark-nlp-display
A library for the simple visualization of different types of Spark NLP annotations.
Supported Visualizations:
- Dependency Parser
- Named Entity Recognition
- Entity Resolution
- Relation Extraction
- Assertion Status
Complete Tutorial

https://github.com/JohnSnowLabs/spark-nlp-display/blob/main/tutorials/Spark_NLP_Display.ipynb
Requirements
- spark-nlp
- ipython
- svgwrite
- pandas
- numpy
Installation
pip install spark-nlp-display
How to use
Databricks
For all modules, pass in the additional parameter "return_html=True" in the display function and use Databrick's function displayHTML() to render visualization as explained below:
from sparknlp_display import NerVisualizer
ner_vis = NerVisualizer()
ner_vis.set_label_colors({'LOC':'#800080', 'PER':'#77b5fe'})
pipeline_result = ner_light_pipeline.fullAnnotate(text)
vis_html = ner_vis.display(pipeline_result[0],
label_col='entities',
document_col='document',
labels=['PER'],
return_html=True)
displayHTML(vis_html)

Jupyter
To save the visualization as html, provide the export file path: save_path='./export.html'
for each visualizer.
Dependency Parser
from sparknlp_display import DependencyParserVisualizer
dependency_vis = DependencyParserVisualizer()
pipeline_result = dp_pipeline.fullAnnotate(text)
dependency_vis.display(pipeline_result[0],
pos_col = 'pos',
dependency_col = 'dependency',
dependency_type_col = 'dependency_type',
save_path='./export.html'
)
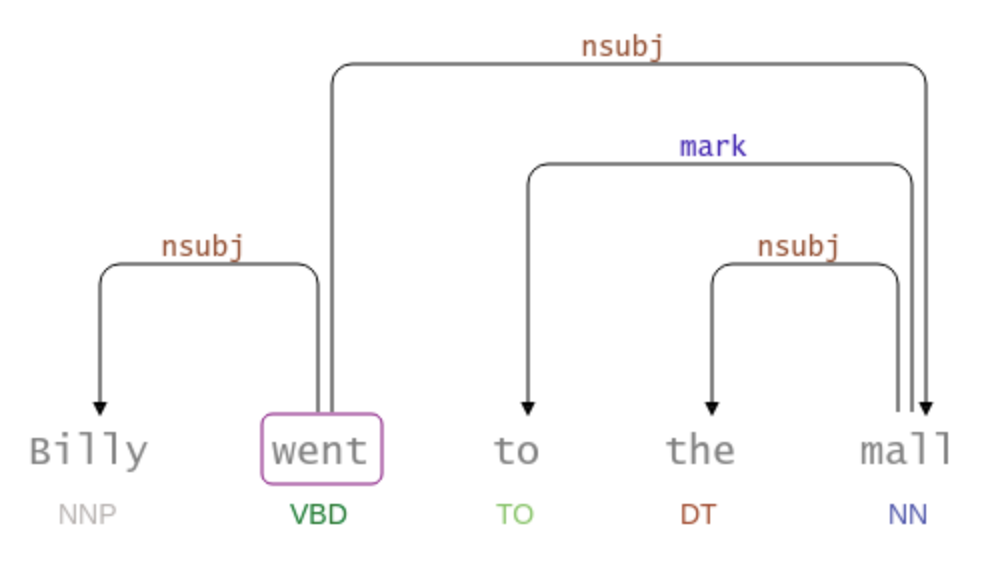
Named Entity Recognition
from sparknlp_display import NerVisualizer
ner_vis = NerVisualizer()
pipeline_result = ner_light_pipeline.fullAnnotate(text)
ner_vis.display(pipeline_result[0],
label_col='entities',
document_col='document',
labels=['PER'],
save_path='./export.html'
)
ner_vis.set_label_colors({'LOC':'#800080', 'PER':'#77b5fe'})

Entity Resolution
from sparknlp_display import EntityResolverVisualizer
er_vis = EntityResolverVisualizer()
pipeline_result = er_light_pipeline.fullAnnotate(text)
er_vis.display(pipeline_result[0],
label_col='entities',
resolution_col = 'resolution',
document_col='document',
save_path='./export.html'
)
er_vis.set_label_colors({'TREATMENT':'#800080', 'PROBLEM':'#77b5fe'})
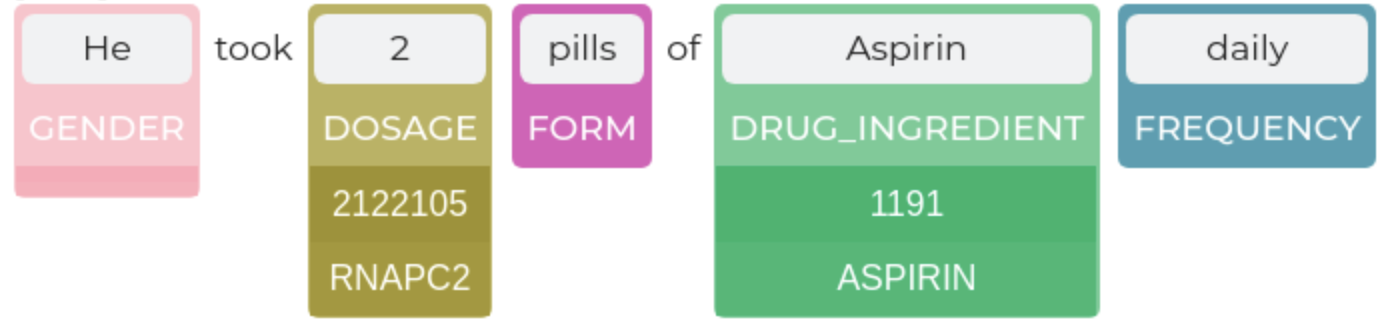
from sparknlp_display import RelationExtractionVisualizer
re_vis = RelationExtractionVisualizer()
pipeline_result = re_light_pipeline.fullAnnotate(text)
re_vis.display(pipeline_result[0],
relation_col = 'relations',
document_col = 'document',
show_relations=True,
save_path='./export.html'
)

Assertion Status
from sparknlp_display import AssertionVisualizer
assertion_vis = AssertionVisualizer()
pipeline_result = ner_assertion_light_pipeline.fullAnnotate(text)
assertion_vis.display(pipeline_result[0],
label_col = 'entities',
assertion_col = 'assertion',
document_col = 'document',
save_path='./export.html'
)
assertion_vis.set_label_colors({'TREATMENT':'#008080', 'problem':'#800080'})
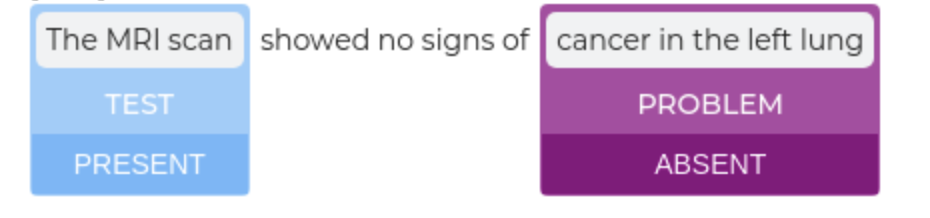