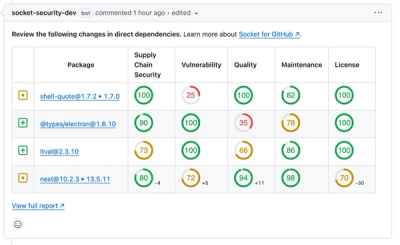
Product
A New Design for GitHub PR Comments
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.
A simple IP lookup tool written in Python with concurrency support.
SpyIP uses ip-api API to trace IP addresses. SpyIP is default synchronous and supports asynchronous operations. It is type annotated, PEP8 compliant, documented and unit tested.
Trace your own IP address
Trace your own DNS
Trace IP address by query
Trace batch IP address
Asynchronous supported
Synchronous by default
Type annotated
Unit tested
PEP8 compliant
Documented
Optimized
pip install spyip
Default synchronous
use case for normal and easy to use.
from spyip import trace_me, trace_dns, trace_ip, trace_ip_batch # default synchronous functions
me = trace_me() # trace your own IP
print(me.json(indent=4)) # print as JSON with indent
dns = trace_dns() # trace your own DNS
print(dns.json()) # print as JSON
# trace by IP address (facebook.com)
ip = trace_ip(query="31.13.64.35")
print(ip.json()) # print as JSON
# set timeout
print(
trace_ip(
query="31.13.64.35",
timeout=5,
).json()
)
# batch trace by IP address (facebook.com, google.com, github.com, microsoft.com, ...)
batch = trace_ip_batch(
query_list=[
'31.13.64.35', # facebook.com
'142.250.193.206', # google.com
'20.205.243.166', # github.com
'20.236.44.162', # microsoft.com
],
)
print(batch) # print a list of IPResponse objects. (see below)
Advance asynchronous
way to boost performance. Just a simple example to show how to use asyncio
with SpyIP
.
import asyncio
from spyip.backends.asynchronous import trace_me, trace_dns, trace_ip, trace_ip_batch # asynchronous functions
async def spy_trace_me():
result = await trace_me()
print(result)
async def spy_trace_dns():
result = await trace_dns()
print(result)
async def spy_trace_ip():
result = await trace_ip(query="31.13.64.35")
print(result)
async def spy_trace_ip_batch():
result = await trace_ip_batch(
query_list=[
'31.13.64.35', # facebook.com
'142.250.193.206', # google.com
'20.205.243.166', # github.com
'20.236.44.162', # microsoft.com
],
)
print(result)
async def main():
'''Load all tasks'''
await spy_trace_me()
await spy_trace_dns()
await spy_trace_ip()
await spy_trace_ip_batch()
asyncio.run(main()) # run the main function
Localized city
, regionName
and country
can be translated to the following languages by passing lang
argument to trace_me
, trace_ip
and trace_ip_batch
functions. Default language is en
(English). Here is the list of supported languages:
lang (ISO 639) | description |
---|---|
en | English (default) |
de | Deutsch (German) |
es | Español (Spanish) |
pt-BR | Português - Brasil (Portuguese) |
fr | Français (French) |
ja | 日本語 (Japanese) |
zh-CN | 中国 (Chinese) |
ru | Русский (Russian) |
Response objects are attrs
for defining Response
models. In SpyIP
we have 2 response objects respectively:
IPResponse
: for single IP address query
@define
class IPResponse:
"""
Example response from API:
{
"status": "success",
"continent": "Asia",
"continentCode": "AS",
"country": "India",
"countryCode": "IN",
"region": "DL",
"regionName": "National Capital Territory of Delhi",
"city": "New Delhi",
"district": "",
"zip": "110001",
"lat": 28.6139,
"lon": 77.209,
"timezone": "Asia/Kolkata",
"offset": 19800,
"currency": "INR",
"isp": "Google LLC",
"org": "Google LLC",
"as": "AS15169 Google LLC",
"asname": "GOOGLE",
"mobile": false,
"proxy": false,
"hosting": true,
"query": "142.250.193.206",
}
"""
status: str = field(metadata={'description': 'Status of the request.'})
continent: str = field(metadata={'description': 'Continent name.'})
continentCode: str = field(metadata={'description': 'Continent code.'})
country: str = field(metadata={'description': 'Country name.'})
countryCode: str = field(metadata={'description': 'Country code.'})
region: str = field(metadata={'description': 'Region code.'})
regionName: str = field(metadata={'description': 'Region name.'})
city: str = field(metadata={'description': 'City name.'})
district: str = field(metadata={'description': 'District name.'})
zip_: str = field(metadata={'description': 'Zip code.'}, alias='zip')
lat: float = field(metadata={'description': 'Latitude.'})
lon: float = field(metadata={'description': 'Longitude.'})
timezone: str = field(metadata={'description': 'Timezone.'})
offset: int = field(metadata={'description': 'Offset.'})
currency: str = field(metadata={'description': 'Currency.'})
isp: str = field(metadata={'description': 'ISP name.'})
org: str = field(metadata={'description': 'Organization name.'})
as_: str = field(metadata={'description': 'AS number and name.'}, alias='as_')
asname: str = field(metadata={'description': 'AS name.'})
mobile: bool = field(metadata={'description': 'Mobile status.'})
proxy: bool = field(metadata={'description': 'Proxy status.'})
hosting: bool = field(metadata={'description': 'Hosting status.'})
query: str = field(metadata={'description': 'IP address.'})
DNSResponse
: for DNS query
@define
class DNSResponse:
"""
Example response from API:
"dns": {
"ip": "74.125.73.83",
"geo": "United States - Google"
}
"""
ip: str = field(metadata={'description': 'IP address.'})
geo: str = field(metadata={'description': 'Geo location.'})
In batch query, trace_ip_batch
returns a list of IPResponse
objects (List[IPResponse]
). You can just iterate over the list and use as you need.
SpyIP
has 3 custom exceptions:
TooManyRequests
- raised when you exceed the API rate limit.ConnectionTimeout
- raised when connection times out.StatusError
- raised when API returns an error status.Test cases are located in tests
directory. You can run tests with the following commands:
# run all tests in tests directory at once
python -m unittest discover -s tests
# run asynchronous tests
python tests/test_asynchronous.py
# run synchronous tests
python tests/test_synchronous.py
Types | Tests | Time |
---|---|---|
Total tests | 12 | 6.188s |
Asynchronous | 6 | 2.751s |
Synchronous | 6 | 2.968s |
Note: This time comparison is based on network performance and hardware capabilities. I am using a very old low-configuration laptop and running on Python 3.11.7. This result may vary depending on your system resources.
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change. If you have any idea, suggestion or question, feel free to open an issue. Please make sure to update tests as appropriate.
This project is licensed under the terms of the MIT license.
FAQs
A simple IP lookup tool written in Python with concurrency support.
We found that spyip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.
Product
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
Security News
Slopsquatting is a new supply chain threat where AI-assisted code generators recommend hallucinated packages that attackers register and weaponize.