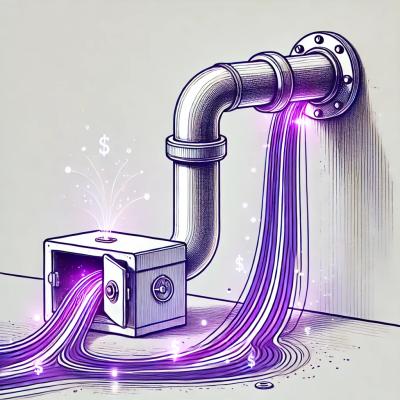
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
A Python package that converts table images into HTML format using Object Detection model and OCR.
pip install table2html
from table2html import Table2HTML
table_config = {
"model_path": r"table2html\models\det_table_v1.pt",
"confidence_threshold": 0.25,
"iou_threshold": 0.7,
}
row_config = {
"model_path": r"table2html\models\det_row_v0.pt",
"confidence_threshold": 0.25,
"iou_threshold": 0.7,
"task": "detect",
}
column_config = {
"model_path": r"table2html\models\det_col_v0.pt",
"confidence_threshold": 0.25,
"iou_threshold": 0.7,
"task": "detect",
}
table2html = Table2HTML(table_config, row_config, column_config)
image = cv2.imread(r"table2html\images\sample.jpg")
detection_data = table2html.TableDetect(image)
# Output: [{"table_bbox": Tuple[int]}]
# Visualize table detection (first table)
from table2html.source import visualize_boxes
cv2.imwrite(
"table_detection.jpg",
visualize_boxes(
image,
[detection_data[0]["table_bbox"]],
color=(0, 0, 255),
thickness=1
)
)
Table detection result:
data = table2html.StructureDetect(image)
# Output: {
# "cells": List[Dict],
# "num_rows": int,
# "num_cols": int,
# "html": str
# }
# Visualize structure detection
from table2html.source import visualize_boxes
cv2.imwrite(
"structure_detection.jpg",
visualize_boxes(
image,
[cell['box'] for cell in data['cells']],
color=(0, 255, 0),
thickness=1
)
)
# Write HTML output
with open('table.html', 'w') as f:
f.write(data["html"])
Structure detection result:
HTML output: extracted html.
Note: The cell coordinates are relative to the cropped table image.
table_crop_padding = 15
detection_data = table2html(image, table_crop_padding)
# Output: [{
# "table_bbox": Tuple[int],
# "cells": List[Dict],
# "num_rows": int,
# "num_cols": int,
# "html": str
# }]
for i, data in enumerate(detection_data):
table_image = crop_image(image, data["table_bbox"], table_crop_padding)
cv2.imwrite(
"table_detection.jpg",
visualize_boxes(
image,
[data["table_bbox"]],
color=(0, 0, 255),
thickness=1
)
)
cv2.imwrite(
"structure_detection.jpg",
visualize_boxes(
table_image,
[cell['box'] for cell in data['cells']],
color=(0, 255, 0),
thickness=1
)
)
with open(f"table_{i}.html", "w") as f:
f.write(data["html"])
image
: numpy.ndarray (OpenCV/cv2 image format)A list of extracted tables in structured:
table_bbox
: Tuple[int] - Bounding box coordinates (x1, y1, x2, y2) of the tablecells
: List[Dict] - List of cell dictionaries, where each dictionary contains:
row
: int - Row indexcolumn
: int - Column indexbox
: Tuple[int] - Bounding box coordinates (x1, y1, x2, y2)text
: str - Cell text contentnum_rows
: int - Number of rows in the tablenum_cols
: int - Number of columns in the tablehtml
: str - HTML representation of the tableThis project is licensed under the Apache License 2.0. See the LICENSE file for details.
FAQs
Detect and convert table image to html table
We found that table2html demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.