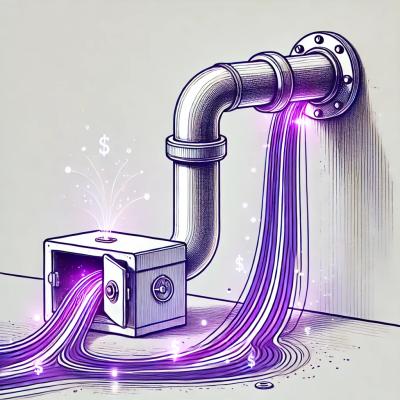
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
github.com/huandu/go-assert
assert
- Magic assert macros for GoPackage assert
provides developer a way to assert expression and output useful contextual information automatically when a case fails.
With this package, we can focus on writing test code without worrying about how to print lots of verbose debug information for debug.
Here is a quick sample.
import "github.com/huandu/go-assert"
func TestSomething(t *testing.T) {
str := Foo(42)
assert.Assert(t, str == "expected")
// This case fails with following message.
//
// Assertion failed:
// str == "expected"
// Referenced variables are assigned in following statements:
// str := Foo(42)
}
Use go get
to install this package.
go get github.com/huandu/go-assert
Current stable version is v1.*
. Old versions tagged by v0.*
are obsoleted.
If we just want to use functions like Assert
, Equal
or NotEqual
, it's recommended to import this package as .
.
import "github.com/huandu/go-assert"
func TestSomething(t *testing.T) {
a, b := 1, 2
assert.Assert(t, a > b)
// This case fails with message:
// Assertion failed:
// a > b
}
func TestAssertEquality(t *testing.T) {
assert.Equal(t, map[string]int{
"foo": 1,
"bar": -2,
}, map[string]int{
"bar": -2,
"foo": 10000,
})
// This case fails with message:
// Assertion failed:
// The value of following expression should equal.
// [1] map[string]int{
// "foo": 1,
// "bar": -2,
// }
// [2] map[string]int{
// "bar": -2,
// "foo": 10000,
// }
// Values:
// [1] -> (map[string]int)map[bar:-2 foo:1]
// [2] -> (map[string]int)map[bar:-2 foo:10000]
}
A
If we want more controls on assertion, it's recommended to wrap t
in an A
.
There are lots of useful assert methods implemented in A
.
Assert
/Eqaul
/NotEqual
: Basic assertion methods.NilError
/NonNilError
: Test if a func/method returns expected error.Use
: Track variables. If any assert method fails, all variables tracked by A
and related in assert method will be printed out automatically in assertion message.Here is a sample to demonstrate how to use A#Use
to print related variables in assertion message.
import "github.com/huandu/go-assert"
func TestSomething(t *testing.T) {
a := assert.New(t)
v1 := 123
v2 := []string{"wrong", "right"}
v3 := v2[0]
v4 := "not related"
a.Use(&v1, &v2, &v3, &v4)
a.Assert(v1 == 123 && v3 == "right")
// This case fails with following message.
//
// Assertion failed:
// v1 == 123 && v3 == "right"
// Referenced variables are assigned in following statements:
// v1 := 123
// v3 := v2[0]
// Related variables:
// v1 -> (int)123
// v2 -> ([]string)[wrong right]
// v3 -> (string)wrong
}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.