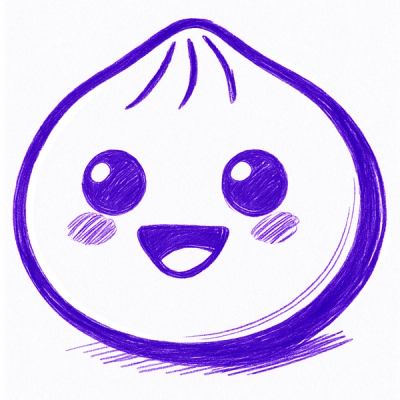
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
github.com/shinexia/executors-go
Implement a stateful task executor, Executor
, with the following features:
func(sin any) (sout any, err error)
.Pipe
(sequential), Map
(data parallel), and Parallel
(compute parallel) methods.import (
"fmt"
"math/rand"
"github.com/shinexia/executors-go"
)
task := executors.Pipe(
// split
func(sin int) (any, error) {
if rand.Intn(100) < 50 {
// must return the original sin if error
return sin, fmt.Errorf("split error")
}
out := make([]int, sin)
for i := range sin {
out[i] = i
}
return out, nil
},
// map
executors.Map(func(sin int) (any, error) {
if n := rand.Intn(100); n < 50 {
// must return the original sin if error
return sin, fmt.Errorf("map error")
}
return sin * 100, nil
}),
// reduce
func(sin []int) (any, error) {
if n := rand.Intn(100); n < 50 {
// must return the original sin if error
return sin, fmt.Errorf("reduce error")
}
return executors.Sum(sin), nil
},
)
var snapshot []byte = nil
var result int
for {
var sin any = nil
if len(snapshot) == 0 {
sin = 10
} else {
// load state from snapshot
state := &executors.TaskState{}
json.Unmarshal(snapshot, state)
sin = state.Stateful
}
sout, err := executors.Run("test", task, sin, executors.WithCallback(func(state *executors.TaskState, err error) {
// dump sout to a file or db
snapshot, _ = json.Marshal(state)
}))
if err != nil {
fmt.Printf("retring, sout: %v, err: %v\n", executors.ToJSON(sout), err)
if executors.IsRuntimeError(err) {
t.Fatalf("runtime error: %+v", err)
}
continue
}
result, _ = executors.Cast[int](sout)
fmt.Printf("succeed, sout: %v\n", sout)
break
}
if result != 4500 {
t.Errorf("result: %v, expect: 4500", result)
}
See examples_test.go
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.