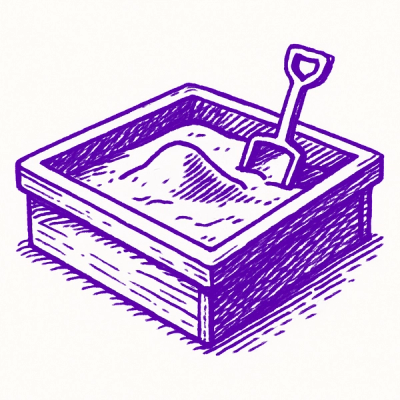
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@asphalt-react/breadcrumb
Advanced tools
Breadcrumb gives users navigational awareness inside the app. It is collection of Crumbs & Separators. Breadcrumb uses /
as separator by default but it also enables customizing it. Crumbs work with both an anchor tag or any client side routers. Crumbs can also have a non link text as well.
Breadcrumb adds a scroll when the number of Crumbs exceeds the space available, Additionally you can collapse some Crumbs to have compact form. Breadcrumb is a controlled component.
import { Breadcrumb, Crumb, CrumbLink } from "@asphalt-react/breadcrumb"
<Breadcrumb
crumbs={[
<Crumb key="0">
<CrumbLink asProps={{ href: "/" }}>
Home
</CrumbLink>,
</Crumb>,
<Crumb key="1">
<CrumbLink asProps={{ href: "/blog" }}>
Blog
</CrumbLink>
</Crumb>,
<Crumb key="2">
<CrumbLink asProps={{ href: "/blog/boom" }}>
Boom
</CrumbLink>
</Crumb>
]}
/>
Breadcrumb component comes with:
BaseBreadcrumb
is a wrapper component which renders an ordered list containing its children
.
Use Crumb
to create a list item for Breadcrumb.
Use CrumbLink
to create link for Breadcrumb.
You can provide custom separator using Separator
.
Breadcrumbs can render in collapsed mode. Breadcrumb doesn't manage the collapsed state on its own it depends on collapsed
prop to change the state from expanded to collapsed and vice versa. In collapsed state you can choose to hide specific Crumbs using collapseIndices
prop and render a button instead. This button invokes onCollapse
callback to expand the hidden Crumbs. You can also pass any custom node using collapseAs
prop but in that case you need to handle the interaction on your own.
import { Breadcrumb, Crumb, CrumbLink } from "@asphalt-react/breadcrumb"
export default App() {
const [collapsed, setCollapsed] = React.useState(true)
return (
<Breadcrumb
collapsed={collapsed}
collapseIndices={[1]}
onCollapse={() => setCollapsed(false)}
crumbs={[
<Crumb key="0">
<CrumbLink as={Link} asProps={{ to: "/" }}>
Home
</CrumbLink>
</Crumb>,
<Crumb key="1">
<CrumbLink as={Link} asProps={{ to: "/blog" }}>
Blog
</CrumbLink>
</Crumb>,
<Crumb key="2">
<CrumbLink as={Link} asProps={{ to: "/blog/boom" }}>
Boom
</CrumbLink>
</Crumb>,
]}
/>
)
}
aria-labels
.aria-hidden
to hide it from assistive technology.List of crumbs.
type | required | default |
---|---|---|
arrayOf | true | N/A |
Makes Breadcrumb collapse.
type | required | default |
---|---|---|
bool | false | false |
React node for separator.
type | required | default |
---|---|---|
node | false | "/" |
React node render in the collapsed state of Breadcrumb
type | required | default |
---|---|---|
node | false | null |
crumbs indices to collapse, must be in range and consecutive numbers.
type | required | default |
---|---|---|
arrayOf | false | [] |
function to call when collapseAs is clicked.
type | required | default |
---|---|---|
func | false | null |
BaseBreadcrumb is base wrapper component which renders an ordered list containing its children
. It adds scroll on overflow to make its content visible.
React node to render as children
type | required | default |
---|---|---|
node | true | N/A |
Component to add a list item in Breadcrumb.
React node to render link content.
type | required | default |
---|---|---|
node | true | N/A |
Component to add link in Crumb
. CrumbLink
handles the link pseudo-classes and additionally you can remove the ":visited" pseudo-class using visited
prop.
React node to render as children.
type | required | default |
---|---|---|
node | true | N/A |
Html element/React component to replace the default element. Using this prop, you can use your router's link element, such as "react-router-dom"'s Link element.
type | required | default |
---|---|---|
elementType | false | "a" |
Pass the props such as "href", "id" for the custom link element passed in as
prop.
type | required | default |
---|---|---|
object | false | {} |
shows visited state for the link.
type | required | default |
---|---|---|
bool | false | true |
Component to add text in Crumb
list item.
React node to render as children.
type | required | default |
---|---|---|
node | true | N/A |
Component to add the separator symbol between crumbs.
React node to render as children.
type | required | default |
---|---|---|
node | true | N/A |
FAQs
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.