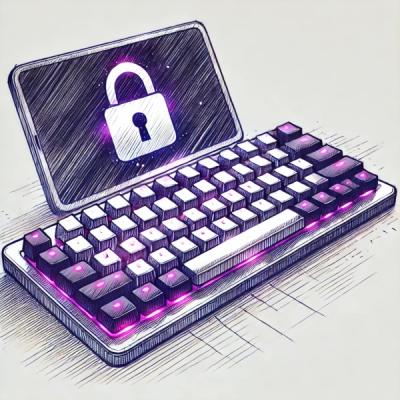
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
@aws-cdk/aws-iam
Advanced tools
Package description
@aws-cdk/aws-iam is an AWS Cloud Development Kit (CDK) library that allows you to define AWS Identity and Access Management (IAM) resources in your CDK applications. This package provides constructs for creating and managing IAM roles, users, policies, and groups, enabling you to manage permissions and access control in your AWS environment programmatically.
Create IAM Role
This code sample demonstrates how to create an IAM role that can be assumed by EC2 instances and has read-only access to Amazon S3.
const iam = require('@aws-cdk/aws-iam');
const cdk = require('@aws-cdk/core');
class MyStack extends cdk.Stack {
constructor(scope, id, props) {
super(scope, id, props);
new iam.Role(this, 'MyRole', {
assumedBy: new iam.ServicePrincipal('ec2.amazonaws.com'),
managedPolicies: [
iam.ManagedPolicy.fromAwsManagedPolicyName('AmazonS3ReadOnlyAccess')
]
});
}
}
const app = new cdk.App();
new MyStack(app, 'MyStack');
Create IAM User
This code sample demonstrates how to create an IAM user with administrator access.
const iam = require('@aws-cdk/aws-iam');
const cdk = require('@aws-cdk/core');
class MyStack extends cdk.Stack {
constructor(scope, id, props) {
super(scope, id, props);
new iam.User(this, 'MyUser', {
userName: 'my-user',
managedPolicies: [
iam.ManagedPolicy.fromAwsManagedPolicyName('AdministratorAccess')
]
});
}
}
const app = new cdk.App();
new MyStack(app, 'MyStack');
Attach Inline Policy to Role
This code sample demonstrates how to create an IAM role and attach an inline policy that allows listing objects in a specific S3 bucket.
const iam = require('@aws-cdk/aws-iam');
const cdk = require('@aws-cdk/core');
class MyStack extends cdk.Stack {
constructor(scope, id, props) {
super(scope, id, props);
const role = new iam.Role(this, 'MyRole', {
assumedBy: new iam.ServicePrincipal('lambda.amazonaws.com')
});
role.addToPolicy(new iam.PolicyStatement({
actions: ['s3:ListBucket'],
resources: ['arn:aws:s3:::my-bucket']
}));
}
}
const app = new cdk.App();
new MyStack(app, 'MyStack');
The aws-sdk package is the official AWS SDK for JavaScript, which allows you to interact with AWS services, including IAM, using JavaScript. Unlike @aws-cdk/aws-iam, which is used for defining and deploying AWS infrastructure, aws-sdk is used for making API calls to AWS services.
The serverless framework is a toolkit for deploying and operating serverless architectures, including AWS Lambda functions and associated IAM roles and policies. It provides a higher-level abstraction compared to @aws-cdk/aws-iam and is focused on serverless applications.
Terraform is an open-source infrastructure as code software tool that provides a consistent CLI workflow to manage hundreds of cloud services, including AWS IAM. It is similar to @aws-cdk/aws-iam in that it allows you to define and manage AWS infrastructure, but it is not limited to AWS and supports multiple cloud providers.
Changelog
Readme
Define a role and add permissions to it. This will automatically create and attach an IAM policy to the role:
Define a policy and attach it to groups, users and roles. Note that it is possible to attach
the policy either by calling xxx.attachInlinePolicy(policy)
or policy.attachToXxx(xxx)
.
attaching policies to user and group
Managed policies can be attached using xxx.attachManagedPolicy(arn)
:
If you need to create roles that will be assumed by 3rd parties, it is generally a good idea to require an ExternalId
to assume them. Configuring
an ExternalId
works like this:
When defining policy statements as part of an AssumeRole policy or as part of a
resource policy, statements would usually refer to a specific IAM principal
under Principal
.
IAM principals are modeled as classes that derive from the iam.PolicyPrincipal
abstract class. Principal objects include principal type (string) and value
(array of string), optional set of conditions and the action that this principal
requires when it is used in an assume role policy document.
To add a principal to a policy statement you can either use the abstract
statement.addPrincipal
, one of the concrete addXxxPrincipal
methods:
addAwsPrincipal
, addArnPrincipal
or new ArnPrincipal(arn)
for { "AWS": arn }
addAwsAccountPrincipal
or new AccountPrincipal(accountId)
for { "AWS": account-arn }
addServicePrincipal
or new ServicePrincipal(service)
for { "Service": service }
addAccountRootPrincipal
or new AccountRootPrincipal()
for { "AWS": { "Ref: "AWS::AccountId" } }
addCanonicalUserPrincipal
or new CanonicalUserPrincipal(id)
for { "CanonicalUser": id }
addFederatedPrincipal
or new FederatedPrincipal(federated, conditions, assumeAction)
for
{ "Federated": arn }
and a set of optional conditions and the assume role action to use.addAnyPrincipal
or new AnyPrincipal
for { "AWS": "*" }
If multiple principals are added to the policy statement, they will be merged together:
const statement = new PolicyStatement();
statement.addServicePrincipal('cloudwatch.amazonaws.com');
statement.addServicePrincipal('ec2.amazonaws.com');
statement.addAwsPrincipal('arn:aws:boom:boom');
Will result in:
{
"Principal": {
"Service": [ "cloudwatch.amazonaws.com", "ec2.amazonaws.com" ],
"AWS": "arn:aws:boom:boom"
}
}
The CompositePrincipal
class can also be used to define complex principals, for example:
const role = new iam.Role(this, 'MyRole', {
assumedBy: new iam.CompositePrincipal(
new iam.ServicePrincipal('ec2.amazonawas.com'),
new iam.AccountPrincipal('1818188181818187272')
)
});
FAQs
Unknown package
The npm package @aws-cdk/aws-iam receives a total of 130,696 weekly downloads. As such, @aws-cdk/aws-iam popularity was classified as popular.
We found that @aws-cdk/aws-iam demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.