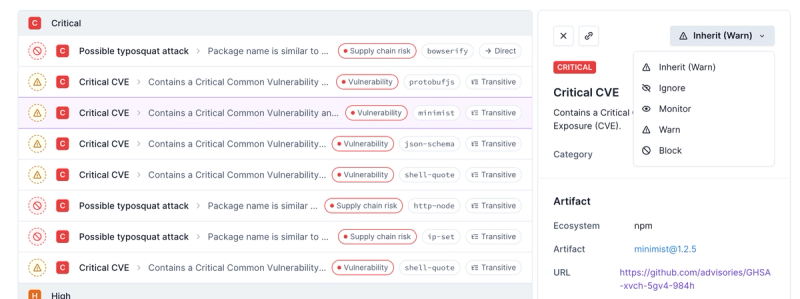
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@aws-cdk/aws-kms
Advanced tools
Package description
@aws-cdk/aws-kms is an AWS CDK library that allows you to define and manage AWS Key Management Service (KMS) resources in your AWS infrastructure as code. It provides constructs for creating and managing KMS keys, aliases, and grants, enabling secure encryption and decryption of data.
Create a KMS Key
This code sample demonstrates how to create a new KMS key with key rotation enabled and an alias using the AWS CDK.
const cdk = require('@aws-cdk/core');
const kms = require('@aws-cdk/aws-kms');
const app = new cdk.App();
const stack = new cdk.Stack(app, 'MyStack');
const key = new kms.Key(stack, 'MyKey', {
enableKeyRotation: true,
alias: 'alias/my-key'
});
app.synth();
Create a KMS Alias
This code sample demonstrates how to create a new KMS alias that points to an existing KMS key using the AWS CDK.
const cdk = require('@aws-cdk/core');
const kms = require('@aws-cdk/aws-kms');
const app = new cdk.App();
const stack = new cdk.Stack(app, 'MyStack');
const key = new kms.Key(stack, 'MyKey');
const alias = new kms.Alias(stack, 'MyAlias', {
aliasName: 'alias/my-alias',
targetKey: key
});
app.synth();
Grant Permissions to a KMS Key
This code sample demonstrates how to grant encrypt and decrypt permissions to an IAM user for a KMS key using the AWS CDK.
const cdk = require('@aws-cdk/core');
const kms = require('@aws-cdk/aws-kms');
const iam = require('@aws-cdk/aws-iam');
const app = new cdk.App();
const stack = new cdk.Stack(app, 'MyStack');
const key = new kms.Key(stack, 'MyKey');
const user = new iam.User(stack, 'MyUser');
key.grantEncryptDecrypt(user);
app.synth();
The aws-sdk package is the official AWS SDK for JavaScript, which provides a comprehensive set of tools for interacting with AWS services, including KMS. Unlike @aws-cdk/aws-kms, which is used for defining infrastructure as code, aws-sdk is used for making API calls to AWS services directly from your application code.
The serverless package is a framework for building and deploying serverless applications on AWS and other cloud providers. It includes support for managing AWS KMS keys as part of your serverless infrastructure. While it provides similar functionality for managing KMS keys, it is more focused on serverless architectures compared to the broader infrastructure management capabilities of @aws-cdk/aws-kms.
Terraform is an open-source infrastructure as code tool that allows you to define and manage cloud resources, including AWS KMS keys, using a declarative configuration language. It provides similar functionality to @aws-cdk/aws-kms but uses a different syntax and approach to infrastructure management.
Changelog
1.72.0 (2020-11-06)
enableHttpEndpoint
renamed to enableDataApi
outputLocation
in the experimental Athena StartQueryExecution
has been changed to s3.Location
from string
Environment
from attributes (#10932) (d395b5e), closes #10931--no-previous-parameters
incorrectly skips updates (#11288) (1bfc649)Readme
Define a KMS key:
import * as kms from '@aws-cdk/aws-kms';
new kms.Key(this, 'MyKey', {
enableKeyRotation: true
});
Add a couple of aliases:
const key = new kms.Key(this, 'MyKey');
key.addAlias('alias/foo');
key.addAlias('alias/bar');
see Trust Account Identities for additional details
To use a KMS key in a different stack in the same CDK application, pass the construct to the other stack:
see Trust Account Identities for additional details
To use a KMS key that is not defined in this CDK app, but is created through other means, use
Key.fromKeyArn(parent, name, ref)
:
const myKeyImported = kms.Key.fromKeyArn(this, 'MyImportedKey', 'arn:aws:...');
// you can do stuff with this imported key.
myKeyImported.addAlias('alias/foo');
Note that a call to .addToPolicy(statement)
on myKeyImported
will not have
an affect on the key's policy because it is not owned by your stack. The call
will be a no-op.
If a Key has an associated Alias, the Alias can be imported by name and used in place of the Key as a reference. A common scenario for this is in referencing AWS managed keys.
const myKeyAlias = kms.Alias.fromAliasName(this, 'myKey', 'alias/aws/s3');
const trail = new cloudtrail.Trail(this, 'myCloudTrail', {
sendToCloudWatchLogs: true,
kmsKey: myKeyAlias
});
Note that calls to addToResourcePolicy
and grant*
methods on myKeyAlias
will be
no-ops, and addAlias
and aliasTargetKey
will fail, as the imported alias does not
have a reference to the underlying KMS Key.
KMS keys can be created to trust IAM policies. This is the default behavior in the console and is described here. This same behavior can be enabled by:
new Key(stack, 'MyKey', { trustAccountIdentities: true });
Using trustAccountIdentities
solves many issues around cyclic dependencies
between stacks. The most common use case is creating an S3 Bucket with CMK
default encryption which is later accessed by IAM roles in other stacks.
stack-1 (bucket and key created)
// ... snip
const myKmsKey = new kms.Key(this, 'MyKey', { trustAccountIdentities: true });
const bucket = new Bucket(this, 'MyEncryptedBucket', {
bucketName: 'myEncryptedBucket',
encryption: BucketEncryption.KMS,
encryptionKey: myKmsKey
});
stack-2 (lambda that operates on bucket and key)
// ... snip
const fn = new lambda.Function(this, 'MyFunction', {
runtime: lambda.Runtime.NODEJS_10_X,
handler: 'index.handler',
code: lambda.Code.fromAsset(path.join(__dirname, 'lambda-handler')),
});
const bucket = s3.Bucket.fromBucketName(this, 'BucketId', 'myEncryptedBucket');
const key = kms.Key.fromKeyArn(this, 'KeyId', 'arn:aws:...'); // key ARN passed via stack props
bucket.grantReadWrite(fn);
key.grantEncryptDecrypt(fn);
The challenge in this scenario is the KMS key policy behavior. The simple way to understand
this, is IAM policies for account entities can only grant the permissions granted to the
account root principle in the key policy. When trustAccountIdentities
is true,
the following policy statement is added:
{
"Sid": "Enable IAM User Permissions",
"Effect": "Allow",
"Principal": {"AWS": "arn:aws:iam::111122223333:root"},
"Action": "kms:*",
"Resource": "*"
}
As the name suggests this trusts IAM policies to control access to the key. If account root does not have permissions to the specific actions, then the key policy and the IAM policy for the entity (e.g. Lambda) both need to grant permission.
FAQs
The CDK Construct Library for AWS::KMS
The npm package @aws-cdk/aws-kms receives a total of 136,867 weekly downloads. As such, @aws-cdk/aws-kms popularity was classified as popular.
We found that @aws-cdk/aws-kms demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.