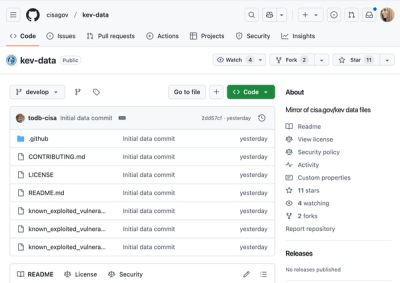
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
@aws-sdk/client-network-firewall
Advanced tools
AWS SDK for JavaScript Network Firewall Client for Node.js, Browser and React Native
AWS SDK for JavaScript NetworkFirewall Client for Node.js, Browser and React Native.
This is the API Reference for Network Firewall. This guide is for developers who need detailed information about the Network Firewall API actions, data types, and errors.
The REST API requires you to handle connection details, such as calculating signatures, handling request retries, and error handling. For general information about using the Amazon Web Services REST APIs, see Amazon Web Services APIs.
To view the complete list of Amazon Web Services Regions where Network Firewall is available, see Service endpoints and quotas in the Amazon Web Services General Reference.
To access Network Firewall using the IPv4 REST API endpoint:
https://network-firewall..amazonaws.com
To access Network Firewall using the Dualstack (IPv4 and IPv6) REST API endpoint:
https://network-firewall..aws.api
Alternatively, you can use one of the Amazon Web Services SDKs to access an API that's tailored to the programming language or platform that you're using. For more information, see Amazon Web Services SDKs.
For descriptions of Network Firewall features, including and step-by-step instructions on how to use them through the Network Firewall console, see the Network Firewall Developer Guide.
Network Firewall is a stateful, managed, network firewall and intrusion detection and prevention service for Amazon Virtual Private Cloud (Amazon VPC). With Network Firewall, you can filter traffic at the perimeter of your VPC. This includes filtering traffic going to and coming from an internet gateway, NAT gateway, or over VPN or Direct Connect. Network Firewall uses rules that are compatible with Suricata, a free, open source network analysis and threat detection engine.
You can use Network Firewall to monitor and protect your VPC traffic in a number of ways. The following are just a few examples:
Allow domains or IP addresses for known Amazon Web Services service endpoints, such as Amazon S3, and block all other forms of traffic.
Use custom lists of known bad domains to limit the types of domain names that your applications can access.
Perform deep packet inspection on traffic entering or leaving your VPC.
Use stateful protocol detection to filter protocols like HTTPS, regardless of the port used.
To enable Network Firewall for your VPCs, you perform steps in both Amazon VPC and in Network Firewall. For information about using Amazon VPC, see Amazon VPC User Guide.
To start using Network Firewall, do the following:
(Optional) If you don't already have a VPC that you want to protect, create it in Amazon VPC.
In Amazon VPC, in each Availability Zone where you want to have a firewall endpoint, create a subnet for the sole use of Network Firewall.
In Network Firewall, create stateless and stateful rule groups, to define the components of the network traffic filtering behavior that you want your firewall to have.
In Network Firewall, create a firewall policy that uses your rule groups and specifies additional default traffic filtering behavior.
In Network Firewall, create a firewall and specify your new firewall policy and VPC subnets. Network Firewall creates a firewall endpoint in each subnet that you specify, with the behavior that's defined in the firewall policy.
In Amazon VPC, use ingress routing enhancements to route traffic through the new firewall endpoints.
To install this package, simply type add or install @aws-sdk/client-network-firewall using your favorite package manager:
npm install @aws-sdk/client-network-firewall
yarn add @aws-sdk/client-network-firewall
pnpm add @aws-sdk/client-network-firewall
The AWS SDK is modulized by clients and commands.
To send a request, you only need to import the NetworkFirewallClient
and
the commands you need, for example ListFirewallsCommand
:
// ES5 example
const { NetworkFirewallClient, ListFirewallsCommand } = require("@aws-sdk/client-network-firewall");
// ES6+ example
import { NetworkFirewallClient, ListFirewallsCommand } from "@aws-sdk/client-network-firewall";
To send a request, you:
send
operation on client with command object as input.destroy()
to close open connections.// a client can be shared by different commands.
const client = new NetworkFirewallClient({ region: "REGION" });
const params = {
/** input parameters */
};
const command = new ListFirewallsCommand(params);
We recommend using await operator to wait for the promise returned by send operation as follows:
// async/await.
try {
const data = await client.send(command);
// process data.
} catch (error) {
// error handling.
} finally {
// finally.
}
Async-await is clean, concise, intuitive, easy to debug and has better error handling as compared to using Promise chains or callbacks.
You can also use Promise chaining to execute send operation.
client.send(command).then(
(data) => {
// process data.
},
(error) => {
// error handling.
}
);
Promises can also be called using .catch()
and .finally()
as follows:
client
.send(command)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
})
.finally(() => {
// finally.
});
We do not recommend using callbacks because of callback hell, but they are supported by the send operation.
// callbacks.
client.send(command, (err, data) => {
// process err and data.
});
The client can also send requests using v2 compatible style. However, it results in a bigger bundle size and may be dropped in next major version. More details in the blog post on modular packages in AWS SDK for JavaScript
import * as AWS from "@aws-sdk/client-network-firewall";
const client = new AWS.NetworkFirewall({ region: "REGION" });
// async/await.
try {
const data = await client.listFirewalls(params);
// process data.
} catch (error) {
// error handling.
}
// Promises.
client
.listFirewalls(params)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
});
// callbacks.
client.listFirewalls(params, (err, data) => {
// process err and data.
});
When the service returns an exception, the error will include the exception information, as well as response metadata (e.g. request id).
try {
const data = await client.send(command);
// process data.
} catch (error) {
const { requestId, cfId, extendedRequestId } = error.$metadata;
console.log({ requestId, cfId, extendedRequestId });
/**
* The keys within exceptions are also parsed.
* You can access them by specifying exception names:
* if (error.name === 'SomeServiceException') {
* const value = error.specialKeyInException;
* }
*/
}
Please use these community resources for getting help. We use the GitHub issues for tracking bugs and feature requests, but have limited bandwidth to address them.
aws-sdk-js
on AWS Developer Blog.aws-sdk-js
.To test your universal JavaScript code in Node.js, browser and react-native environments, visit our code samples repo.
This client code is generated automatically. Any modifications will be overwritten the next time the @aws-sdk/client-network-firewall
package is updated.
To contribute to client you can check our generate clients scripts.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE for more information.
FAQs
AWS SDK for JavaScript Network Firewall Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-network-firewall receives a total of 30,218 weekly downloads. As such, @aws-sdk/client-network-firewall popularity was classified as popular.
We found that @aws-sdk/client-network-firewall demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.