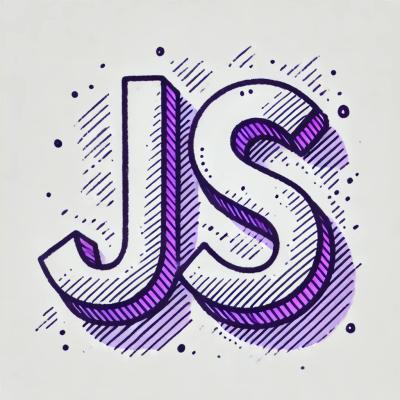
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@aws-sdk/util-stream-node
Advanced tools
[](https://www.npmjs.com/package/@aws-sdk/util-stream-node) [](https://www.npmjs.com/package/@aws
@aws-sdk/util-stream-node is a utility package from the AWS SDK for JavaScript that provides functions to work with Node.js streams. It is particularly useful for handling streaming data in AWS services.
streamToBuffer
Converts a readable stream into a buffer. This is useful when you need to process the entire content of a stream as a single buffer.
const { streamToBuffer } = require('@aws-sdk/util-stream-node');
const { Readable } = require('stream');
const readableStream = Readable.from(['Hello', ' ', 'World']);
streamToBuffer(readableStream).then(buffer => {
console.log(buffer.toString()); // Output: Hello World
});
streamToString
Converts a readable stream into a string. This is useful when you need to process the entire content of a stream as a single string.
const { streamToString } = require('@aws-sdk/util-stream-node');
const { Readable } = require('stream');
const readableStream = Readable.from(['Hello', ' ', 'World']);
streamToString(readableStream).then(string => {
console.log(string); // Output: Hello World
});
bufferToStream
Converts a buffer into a readable stream. This is useful when you need to process a buffer as a stream.
const { bufferToStream } = require('@aws-sdk/util-stream-node');
const buffer = Buffer.from('Hello World');
const stream = bufferToStream(buffer);
stream.on('data', chunk => {
console.log(chunk.toString()); // Output: Hello World
});
The 'stream-to-buffer' package provides a simple utility to convert a stream into a buffer. It is similar to the 'streamToBuffer' function in @aws-sdk/util-stream-node but is a standalone package.
The 'stream-to-string' package provides a utility to convert a stream into a string. It is similar to the 'streamToString' function in @aws-sdk/util-stream-node but is a standalone package.
The 'buffer-to-stream' package provides a utility to convert a buffer into a stream. It is similar to the 'bufferToStream' function in @aws-sdk/util-stream-node but is a standalone package.
Deprecated package
This internal package is deprecated in favor of @aws-sdk/util-stream.
Package with utilities to operate on Node.JS streams.
You probably shouldn't, at least directly.
3.374.0 (2023-07-20)
FAQs
[](https://www.npmjs.com/package/@aws-sdk/util-stream-node) [](https://www.npmjs.com/package/@aws
The npm package @aws-sdk/util-stream-node receives a total of 384,992 weekly downloads. As such, @aws-sdk/util-stream-node popularity was classified as popular.
We found that @aws-sdk/util-stream-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.