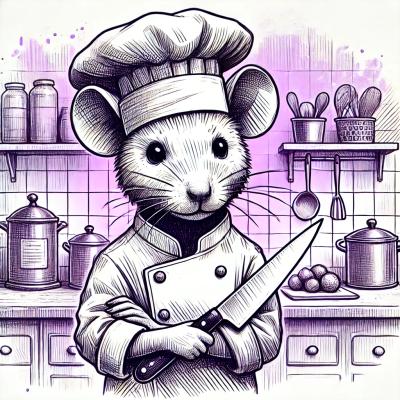
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Representation of identifier of a part of BEM entity.
$ npm install --save @bem/cell
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', elem: 'text', mod: { name: 'theme', val: 'simple' } }),
tech: 'css',
layer: 'common'
});
cell.entity; // ➜ BemEntityName { block: 'button', elem: 'text' }
cell.tech; // css
cell.layer; // common
cell.id; // button__text@common.css
cell.block; // → button
cell.elem; // → text
cell.mod; // → { name: 'theme', val: 'simple' }
Parameter | Type | Description |
---|---|---|
obj.entity | BemEntityName | Representation of entity name |
obj.tech | string | Tech of cell |
obj.layer | string | Layer of cell |
Returns the name of entity.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', elem: 'text' })
});
cell.entity; // ➜ BemEntityName { block: 'button', elem: 'text' }
Returns the tech of cell.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', elem: 'text' }),
tech: 'css'
});
cell.tech; // ➜ css
Returns the layer of this cell.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', elem: 'text' }),
layer: 'desktop'
});
cell.layer; // ➜ desktop
Returns the identifier of this cell.
Important: should only be used to determine uniqueness of cell.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', elem: 'text' }),
tech: 'css',
layer: 'desktop'
});
cell.id; // ➜ "button__text@desktop.css"
Returns a string representing this cell.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', mod: 'focused' }),
tech: 'css',
layer: 'desktop'
});
cell.toString(); // button_focused@desktop.css
Returns an object representing this cell.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', mod: 'focused' }),
tech: 'css',
layer: 'desktop'
});
cell.valueOf();
// ➜ { entity: { block: 'button', mod: { name: 'focused', value: true } }, tech: 'css', layer: 'desktop' }
Returns an object for JSON.stringify()
purpose.
Determines whether specified cell is deep equal to cell or not.
Parameter | Type | Description |
---|---|---|
cell | BemCell | The cell to compare. |
const BemCell = require('@bem/cell');
const buttonCell1 = BemCell.create({ block: 'button', tech: 'css', layer: 'desktop' });
const buttonCell2 = BemCell.create({ block: 'button', tech: 'css', layer: 'desktop' });
const inputCell = BemCell.create({ block: 'input', tech: 'css', layer: 'common' });
buttonCell1.isEqual(buttonCell2); // true
buttonCell1.isEqual(inputCell); // false
Determines whether specified cell is instance of BemCell.
Parameter | Type | Description |
---|---|---|
cell | BemCell | The cell to check. |
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'button', elem: 'text' })
});
BemCell.isBemCell(cell); // true
BemCell.isBemCell({}); // false
Creates BemCell instance by any object representation.
Helper for sugar-free simplicity.
Parameter | Type | Description |
---|---|---|
object | object | Representation of entity name. |
Passed Object could have fields for BemEntityName and cell itself:
Object field | Type | Description |
---|---|---|
block | string | The block name of entity. |
elem | string | The element name of entity. |
mod | string , object | The modifier of entity. If specified value is string then it will be equivalent to { name: string, val: true } . |
val | string | The modifier value of entity. Used if mod is a string. |
mod.name | string | The modifier name of entity. |
mod.val | * | The modifier value of entity. |
modName | string | The modifier name of entity. Used if mod.name wasn't specified. |
modVal | * | The modifier value of entity. Used if neither mod.val nor val were not specified. |
tech | string | Technology of cell. |
layer | string | Layer of cell. |
const BemCell = require('@bem/cell');
BemCell.create({ block: 'my-button', mod: 'theme', val: 'red', tech: 'css', layer: 'common' });
BemCell.create({ block: 'my-button', modName: 'theme', modVal: 'red', tech: 'css', layer: 'common' });
BemCell.create({ entity: { block: 'my-button', modName: 'theme', modVal: 'red' }, tech: 'css' }); // valueOf() format
// → BemCell { entity: { block: 'my-button', mod: { name: 'theme', val: 'red' } }, tech: 'css', layer: 'common' }
In Node.js, console.log()
calls util.inspect()
on each argument without a formatting placeholder.
BemCell
has inspect()
method to get custom string representation of the object.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'input', mod: 'available' }),
tech: 'css'
});
console.log(cell);
// ➜ BemCell { entity: { block: 'input', mod: { name: 'available' } }, tech: 'css' }
You can also convert BemCell
object to a string
.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'input', mod: 'available' }),
tech: 'css'
});
console.log(`cell: ${cell}`);
// ➜ cell: input_available.css
Also BemCell
has toJSON
method to support JSON.stringify()
behaviour.
const BemCell = require('@bem/cell');
const BemEntityName = require('@bem/entity-name');
const cell = new BemCell({
entity: new BemEntityName({ block: 'input', mod: 'available' }),
tech: 'css'
});
console.log(JSON.stringify(cell));
// ➜ {"entity":{"block":"input","mod":{"name":"available","val":true}},"tech":"css"}
Deprecation is performed with depd To silencing deprecation warnings from being output simply use this. Details
NO_DEPRECATION=@bem/cell node app.js
Code and documentation © 2016 YANDEX LLC. Code released under the Mozilla Public License 2.0.
FAQs
Representation of identifier of a part of BEM entity.
We found that @bem/cell demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.