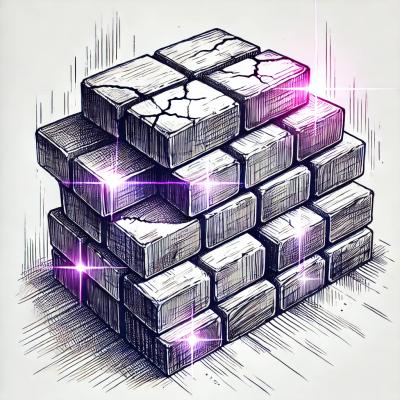
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@buildwars/gw-templates
Advanced tools
A Guild Wars build template encoder/decoder
Encodes and decodes Guild Wars skill and equipment templates, as well as paw·ned² team builds.
ext-sodium
alternatively:
composer require buildwars/gw-templates
{
"require": {
"php": "^8.1",
"buildwars/gw-templates": "^1.0"
}
}
Note: check the releases for valid versions.
npm install @buildwars/gw-templates
{
"dependencies": {
"@buildwars/gw-templates": "^1.0"
}
}
Encode
$code = (new SkillTemplate)->encode(
prof_pri: 7,
prof_sec: 1,
attributes: [29 => 12, 31 => 3, 35 => 12],
skills: [782, 780, 775, 1954, 952, 2356, 1649, 1018],
);
// -> base64 skill template
let code = new SkillTemplate().encode(
7,
1,
{'29': 12, '31': 3, '35': 12},
[782, 780, 775, 1954, 952, 2356, 1649, 1018],
);
// -> base64 skill template
Decode
$skills = (new SkillTemplate)->decode('OwFj0xfzITOMMMHMie4O0kxZ6PA');
let skills = new SkillTemplate().decode('OwFj0xfzITOMMMHMie4O0kxZ6PA');
Array
(
[code] => OwFj0xfzITOMMMHMie4O0kxZ6PA
[prof_pri] => 7
[prof_sec] => 1
[attributes] => Array
(
[29] => 12
[31] => 3
[35] => 12
)
[skills] => Array
(
[0] => 782
[1] => 780
[2] => 775
[3] => 1954
[4] => 952
[5] => 2356
[6] => 1649
[7] => 1018
)
)
Please note that the codes might not necessarily match between decode/encode.
Encode
$equipmentTemplate = new EquipmentTemplate;
// add iems (will overwrite previous items with same slot id)
$equipmentTemplate->addItem(
id: 279,
color: 0,
mods: [190, 204, 329],
);
// ... add more items
$code = $equipmentTemplate->encode(); // -> base64 equipment template
let equipmentTemplate = new EquipmentTemplate();
// add iems (will overwrite previous items with same slot id)
equipmentTemplate.addItem(279, 0, [190, 204, 329]);
// ... add more items
let code = equipmentTemplate.encode(); // -> base64 equipment template
Decode
$equipment = (new EquipmentTemplate)->decode('PkpxFP9FzSqIlpI90MlpIDLfopInVBgpILlLlpIFF');
let equipment = new EquipmentTemplate().decode('PkpxFP9FzSqIlpI90MlpIDLfopInVBgpILlLlpIFF');
Note: the keys of the returned array are the slot IDs (0-6) - they may not be sequential or ordered
Array
(
[0] => Array
(
[id] => 279
[slot] => 0
[color] => 9
[mods] => Array
(
[0] => 190
[1] => 204
[2] => 329
)
)
...more items...
)
Encode
$pwndTemplate = new PwndTemplate;
$pwndTemplate->addBuild(
skills: 'OwFj0xfzITOMMMHMie4O0kxZ6PA',
equipment: 'PkpxFP9FzSqIlpI90MlpIDLfopInVBgpILlLlpIFF',
weaponsets: ['PcZQ8zoRpkC'],
player: '<assigned player/hero>',
description: "<build name>\r\n<description>",
);
// add more builds (up to 12)
$pwnd = $pwndTemplate->encode(); // -> pwnd template code
let pwndTemplate = new PwndTemplate();
pwndTemplate.addBuild(
'OwFj0xfzITOMMMHMie4O0kxZ6PA',
'PkpxFP9FzSqIlpI90MlpIDLfopInVBgpILlLlpIFF',
['PcZQ8zoRpkC'],
'<assigned player/hero>',
'<build name>\r\n<description>',
);
// add more builds (up to 12)
let pwnd = pwndTemplate.encode(); // -> pwnd template code
Decode
The paw-ned² template:
pwnd0000?download paw·ned² @ www.gw-tactics.de Copyright numma_cway aka Redeemer
>aOwFj0xfzITOMMMHMie4O0k6PxZpPkpxFP9FzSqIlpI90MlpIDLfopInVBgpILlLlpIFFAAACgJAAMM
SAtIFdvdEEKZOAOj4wiM5MXTMm3cZS9dJOu5BpPkppFFEqtEAFEqncAFEaqmAFEaY7/EEaYRIHeqXjEA
AACAgAATMiAtIFNvUy9TbWl0ZQoZOQNEApwT2zQDmemuhQOIDQEQjoPgp5PCicJCDBR6JzigItw4SQkh
tDIIyMgJHeqXjEPPgpghmZ9phOzriUAACIhAAOMyAtIFBhbml4CgZOQNDAcw9QvAIg5ZjOkAcQOBoRoP
gpZQCikJCXBR6JnrgItw0VQkht3KIywCKHeqXjEQPkpwRNz6TjdMvKSBAABMAAONCAtIEluZXAxCgZOQ
NDAawDSvAIg5ZrAFgZAEBoRoPgpZQCikJCXBR6JnrgItw0VQkht3KIywCKHeqXjEQPkpwRNz6TjdMvKS
BAACMBAAONSAtIEluZXAyCgbOAhkQkGZIfMzdwQM0qqSzJnw7iBoPgpZRCi8JiYBR6JXsgI7wMWQkhtD
LISOALHeqXjELPkZwUP9akeKAACgJAALNiAtIEJpUAoZOAWiQyhMp7INN5I8Y5wJOOZNBpPkpxUP96Xf
q4npI908npIDLropIvV3npIDr7npITFAAACEBAAONyAtIFJlc3RvCgXOAOiAyk8gNtehzWilD56MvYpP
kp5EFEKuEAFEqncAFEaqmAFEaY7/EEaYBIHiKbkILPkZAIP9akeKAACgBAAKOCAtIFNUCgYOABCY4xEA
glAj4ngdQVFAQZAoPgpxlne9rPVaYKSPNvMFJYJRmiEKtATRGW7ipI7AAAAAABgNSAtIE1vUApzZWNvb
mRhcnkgcHJvZmVzc2lvbiBhbmQgZWxpdGUgc2tpbGwgYXJlIGZyZWUsIGJhcmJzIGlzIG9wdGlvbmFsY
OgNDwcjvOkk6hWEqtp9H0iaBpPkpBUPbTkiqwmpI900mpIDLbipIvSvmpIDrzmpINBAAADAAgAAMNyAt
IEUvTW8K<
$team = (new PwndTemplate)->decode($pwnd);
let team = new PwndTemplate().decode(pwnd);
Array
(
[0] => Array
(
[skills] => OwFj0xfzITOMMMHMie4O0k6PxZ
[equipment] => PkpxFP9FzSqAA5AAJBAZBApBAJ
[weaponsets] => Array
(
[0] =>
[1] =>
[2] =>
)
[player] => Player
[description] => 1 - WotA
)
...more builds...
)
Use at your own risk!
FAQs
A Guild Wars build template encoder/decoder.
The npm package @buildwars/gw-templates receives a total of 0 weekly downloads. As such, @buildwars/gw-templates popularity was classified as not popular.
We found that @buildwars/gw-templates demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.