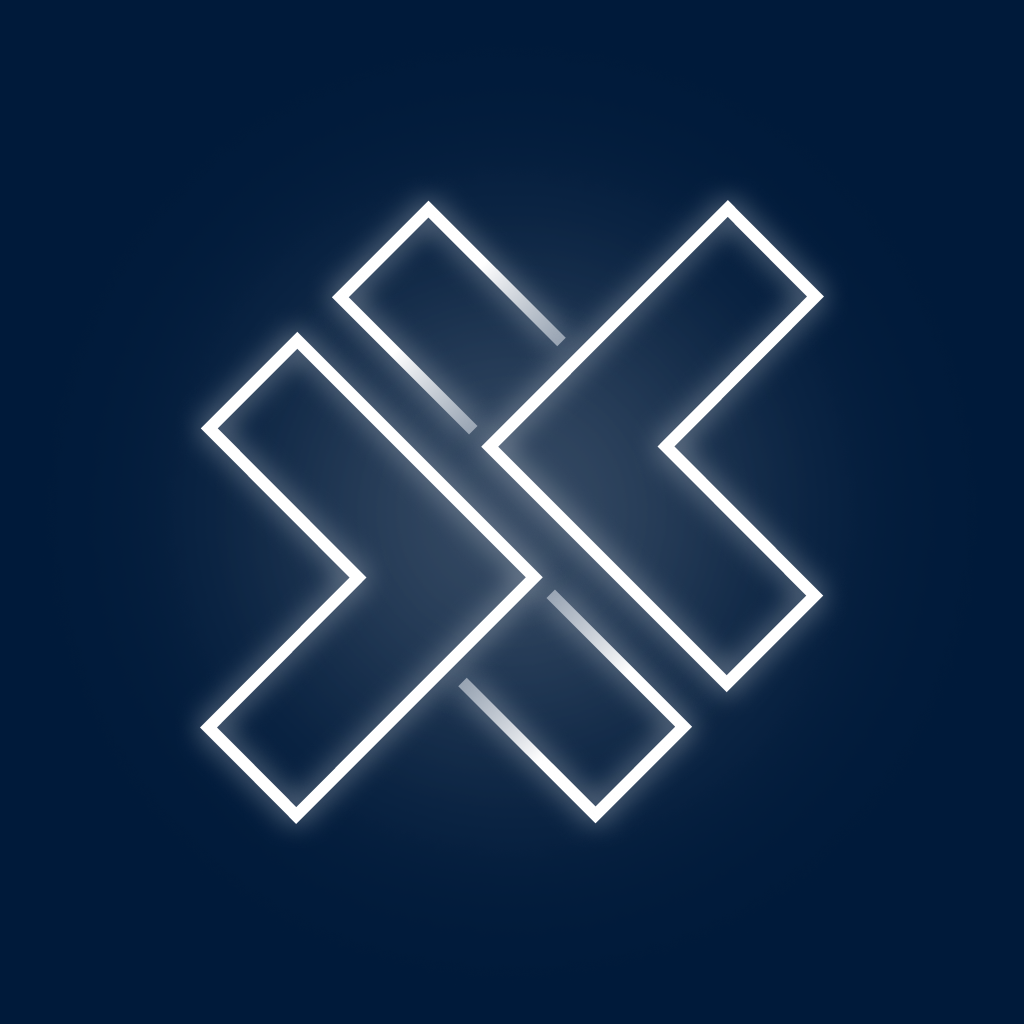
AdMob
@capacitor-community/admob
Capacitor community plugin for native AdMob.
Maintainers
Maintainer | GitHub | Social | Sponsoring Company |
---|
Masahiko Sakakibara | rdlabo | @rdlabo | RELATION DESIGN LABO, GENERAL INC. ASSOCIATION |
Maintenance Status: Actively Maintained
Demo
Demo code is here.
Screenshots
| Banner | Interstitial | Reward |
---|
iOS |  |  |  |
Android |  |  |  |
Installation
% npm install --save @capacitor-community/admob@next
% npx cap update
Android configuration
In file android/app/src/main/java/**/**/MainActivity.java
, add the plugin to the initialization list:
public class MainActivity extends BridgeActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
registerPlugin(com.getcapacitor.community.admob.AdMob.class);
}
}
In file android/app/src/main/AndroidManifest.xml
, add the following XML elements under <manifest><application>
:
<meta-data
android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="@string/admob_app_id"/>
In file android/app/src/main/res/values/strings.xml
add the following lines :
<string name="admob_app_id">[APP_ID]</string>
Don't forget to replace [APP_ID]
by your AdMob application Id.
iOS configuration
Add the following in the ios/App/App/info.plist
file inside of the outermost <dict>
:
<key>GADIsAdManagerApp</key>
<true/>
<key>GADApplicationIdentifier</key>
<string>[APP_ID]</string>
<key>NSUserTrackingUsageDescription</key>
<string>[Why you use NSUserTracking. ex: This identifier will be used to deliver personalized ads to you.]</string>
Don't forget to replace [APP_ID]
by your AdMob application Id.
Initialize
initialize(options: { requestTrackingAuthorization?: boolean , testingDevices?: string[]}): Promise<{ value: boolean }>
You can use option requestTrackingAuthorization
. This change permission to require AppTrackingTransparency
in iOS >= 14:
https://developers.google.com/admob/ios/ios14
Default value is true
. If you don't want to track, set requestTrackingAuthorization false
.
Send and array of device Ids in `testingDevices? to use production like ads on your specified devices -> https://developers.google.com/admob/android/test-ads#enable_test_devices
APIs
BANNER
Angular
import { AdMob, AdOptions, AdSize, AdPosition } from '@capacitor-community/admob';
@Component({
selector: 'admob',
templateUrl: 'admob.component.html',
styleUrls: ['admob.component.scss'],
})
export class AdMobComponent {
private options: AdOptions = {
adId: 'YOUR ADID',
adSize: AdSize.BANNER,
position: AdPosition.BOTTOM_CENTER,
margin: 0,
};
constructor() {
AdMob.addListener('bannerViewDidReceiveAd', (info: boolean) => {
console.log('Banner Ad Loaded');
});
AdMob.addListener('bannerViewChangeSize', (info: boolean) => {
console.log(info);
});
AdMob.showBanner(this.options);
}
}
React
This is implements simple sample from https://github.com/DavidFrahm . Thanks!
import React from 'react';
import { Redirect, Route } from 'react-router-dom';
import { IonApp, IonRouterOutlet, isPlatform } from '@ionic/react';
import { IonReactRouter } from '@ionic/react-router';
import Home from './pages/Home';
import { AdMob, AdOptions, AdSize, AdPosition } from '@capacitor-community/admob';
const App: React.FC = () => {
AdMob.initialize();
const adId = {
ios: 'ios-value-here',
android: 'android-value-here',
};
const platformAdId = isPlatform('android') ? adId.android : adId.ios;
const options: AdOptions = {
adId: platformAdId,
adSize: AdSize.BANNER,
position: AdPosition.BOTTOM_CENTER,
margin: 0,
};
AdMob.showBanner(options);
AdMob.addListener('bannerViewDidReceiveAd', (info: boolean) => {
console.log('Banner ad loaded');
});
AdMob.addListener('bannerViewChangeSize', (info: boolean) => {
console.log(info);
});
return (
<IonApp>
<IonReactRouter>
<IonRouterOutlet>
<Route path="/home" component={Home} exact={true} />
<Route exact path="/" render={() => <Redirect to="/home" />} />
</IonRouterOutlet>
</IonReactRouter>
</IonApp>
);
};
export default App;
INTERSTITIAL
import { AdMob, AdOptions } from '@capacitor-community/admob';
@Component({
selector: 'admob',
templateUrl: 'admob.component.html',
styleUrls: ['admob.component.scss'],
})
export class AppComponent {
options: AdOptions = {
adId: 'YOUR ADID',
};
constructor() {
AdMob.addListener('adDidPresentFullScreenContent', (info: boolean) => {
AdMob.showInterstitial();
});
AdMob.prepareInterstitial(this.options);
}
}
RewardVideo
import { AdMob, AdOptions } from '@capacitor-community/admob';
@Component({
selector: 'admob',
templateUrl: 'admob.component.html',
styleUrls: ['admob.component.scss'],
})
export class AdMobComponent {
options: AdOptions = {
adId: 'YOUR ADID',
};
constructor() {
AdMob.addListener('adDidPresentFullScreenContent', () => {
AdMob.showRewardVideoAd();
});
AdMob.prepareRewardVideoAd(this.options);
}
}
Index
API
initialize(...)
initialize(options: AdMobInitializationOptions) => Promise<void>
Initialize AdMob with AdMobInitializationOptions
Since: 1.1.2
Interfaces
AdMobInitializationOptions
Prop | Type | Description | Default | Since |
---|
requestTrackingAuthorization | boolean | Use or not requestTrackingAuthorization in iOS(>14) | | 1.1.2 |
testingDevices | string[] | An Array of devices IDs that will be marked as tested devices if {@link AdMobInitializationOptions.initializeForTesting} is true (Real Ads will be served to Testing devices but they will not count as 'real'). | | 1.2.0 |
initializeForTesting | boolean | If set to true, the devices on {@link AdMobInitializationOptions.testingDevices} will be registered to receive test production ads. | false | 1.2.0 |
TROUBLE SHOOTING
If you have error:
[error] Error running update: Analyzing dependencies
[!] CocoaPods could not find compatible versions for pod "Google-Mobile-Ads-SDK":
You should run pod repo update
;
License
Capacitor AdMob is MIT licensed.
Contributors ✨
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!