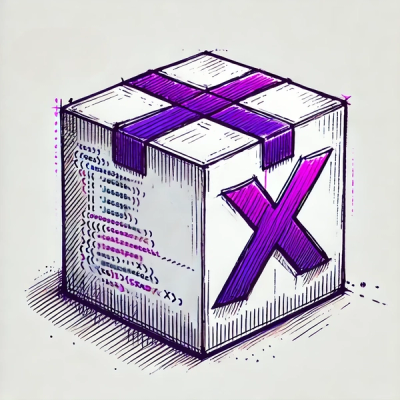
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@datafire/adafruit
Advanced tools
Client library for Adafruit IO REST API
npm install --save @datafire/adafruit
let adafruit = require('@datafire/adafruit').create({
HeaderKey: "",
HeaderSignature: "",
QueryKey: ""
});
.then(data => {
console.log(data);
});
The Adafruit IO HTTP API provides access to your Adafruit IO data from any programming language or hardware environment that can speak HTTP. The easiest way to get started is with an Adafruit IO learn guide and a simple Internet of Things capable device like the Feather Huzzah.
This API documentation is hosted on GitHub Pages and is available at https://github.com/adafruit/io-api. For questions or comments visit the Adafruit IO Forums or the adafruit-io channel on the Adafruit Discord server.
Authentication for every API request happens through the X-AIO-Key
header or query parameter and your IO API key. A simple cURL request to get all available feeds for a user with the username "io_username" and the key "io_key_12345" could look like this:
$ curl -H "X-AIO-Key: io_key_12345" https://io.adafruit.com/api/v2/io_username/feeds
Or like this:
$ curl "https://io.adafruit.com/api/v2/io_username/feeds?X-AIO-Key=io_key_12345
Using the node.js request library, IO HTTP requests are as easy as:
var request = require('request');
var options = {
url: 'https://io.adafruit.com/api/v2/io_username/feeds',
headers: {
'X-AIO-Key': 'io_key_12345',
'Content-Type': 'application/json'
}
};
function callback(error, response, body) {
if (!error && response.statusCode == 200) {
var feeds = JSON.parse(body);
console.log(feeds.length + " FEEDS AVAILABLE");
feeds.forEach(function (feed) {
console.log(feed.name, feed.key);
})
}
}
request(options, callback);
Using the ESP8266 Arduino HTTPClient library, an HTTPS GET request would look like this (replacing ---
with your own values in the appropriate locations):
/// based on
/// https://github.com/esp8266/Arduino/blob/master/libraries/ESP8266HTTPClient/examples/Authorization/Authorization.ino
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <ESP8266WiFiMulti.h>
#include <ESP8266HTTPClient.h>
ESP8266WiFiMulti WiFiMulti;
const char* ssid = "---";
const char* password = "---";
const char* host = "io.adafruit.com";
const char* io_key = "---";
const char* path_with_username = "/api/v2/---/dashboards";
// Use web browser to view and copy
// SHA1 fingerprint of the certificate
const char* fingerprint = "77 00 54 2D DA E7 D8 03 27 31 23 99 EB 27 DB CB A5 4C 57 18";
void setup() {
Serial.begin(115200);
for(uint8_t t = 4; t > 0; t--) {
Serial.printf("[SETUP] WAIT %d...\n", t);
Serial.flush();
delay(1000);
}
WiFi.mode(WIFI_STA);
WiFiMulti.addAP(ssid, password);
// wait for WiFi connection
while(WiFiMulti.run() != WL_CONNECTED) {
Serial.print('.');
delay(1000);
}
Serial.println("[WIFI] connected!");
HTTPClient http;
// start request with URL and TLS cert fingerprint for verification
http.begin("https://" + String(host) + String(path_with_username), fingerprint);
// IO API authentication
http.addHeader("X-AIO-Key", io_key);
// start connection and send HTTP header
int httpCode = http.GET();
// httpCode will be negative on error
if(httpCode > 0) {
// HTTP header has been send and Server response header has been handled
Serial.printf("[HTTP] GET response: %d\n", httpCode);
// HTTP 200 OK
if(httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
}
http.end();
}
}
void loop() {}
We have client libraries to help you get started with your project: Python, Ruby, Arduino C++, Javascript, and Go are available. They're all open source, so if they don't already do what you want, you can fork and add any feature you'd like.
Get information about the current user
adafruit.currentUser(null, context)
This action has no parameters
Send data to a feed via webhook URL.
adafruit.createWebhookFeedData({
"payload": {}
}, context)
object
object
string
The raw data webhook receiver accepts POST requests and stores the raw request body on your feed. This is useful when you don't have control of the webhook sender. If feed history is turned on, payloads will be truncated at 1024 bytes. If feed history is turned off, payloads will be truncated at 100KB.
adafruit.createRawWebhookFeedData(null, context)
This action has no parameters
Delete all your activities.
adafruit.destroyActivities({
"username": ""
}, context)
object
string
: a valid username stringOutput schema unknown
The Activities endpoint returns information about the user's activities.
adafruit.allActivities({
"username": ""
}, context)
object
string
: a valid username stringstring
: Start time for filtering, returns records created after given time.string
: End time for filtering, returns records created before give time.integer
: Limit the number of records returned.array
The Activities endpoint returns information about the user's activities.
adafruit.getActivity({
"username": "",
"type": ""
}, context)
object
string
: a valid username stringstring
string
: Start time for filtering, returns records created after given time.string
: End time for filtering, returns records created before give time.integer
: Limit the number of records returned.array
The Dashboards endpoint returns information about the user's dashboards.
adafruit.allDashboards({
"username": ""
}, context)
object
string
: a valid username stringarray
Create a new Dashboard
adafruit.createDashboard({
"username": "",
"dashboard": {}
}, context)
object
string
: a valid username stringobject
string
string
string
The Blocks endpoint returns information about the user's blocks.
adafruit.allBlocks({
"username": "",
"dashboard_id": ""
}, context)
object
string
: a valid username stringstring
array
Create a new Block
adafruit.createBlock({
"username": "",
"block": {},
"dashboard_id": ""
}, context)
object
string
: a valid username stringobject
array
object
string
string
number
number
string
string
string
object
number
number
number
string
string
Delete an existing Block
adafruit.destroyBlock({
"username": "",
"dashboard_id": "",
"id": ""
}, context)
object
string
: a valid username stringstring
string
string
Returns Block based on ID
adafruit.getBlock({
"username": "",
"dashboard_id": "",
"id": ""
}, context)
object
string
: a valid username stringstring
string
Update properties of an existing Block
adafruit.updateBlock({
"username": "",
"dashboard_id": "",
"id": "",
"block": {}
}, context)
object
string
: a valid username stringstring
string
object
array
object
string
string
number
number
string
string
string
object
number
number
number
string
Replace an existing Block
adafruit.replaceBlock({
"username": "",
"dashboard_id": "",
"id": "",
"block": {}
}, context)
object
string
: a valid username stringstring
string
object
array
object
string
string
number
number
string
string
string
object
number
number
number
string
Delete an existing Dashboard
adafruit.destroyDashboard({
"username": "",
"id": ""
}, context)
object
string
: a valid username stringstring
string
Returns Dashboard based on ID
adafruit.getDashboard({
"username": "",
"id": ""
}, context)
object
string
: a valid username stringstring
Update properties of an existing Dashboard
adafruit.updateDashboard({
"username": "",
"id": "",
"dashboard": {}
}, context)
object
string
: a valid username stringstring
object
string
string
string
Replace an existing Dashboard
adafruit.replaceDashboard({
"username": "",
"id": "",
"dashboard": {}
}, context)
object
string
: a valid username stringstring
object
string
string
string
The Feeds endpoint returns information about the user's feeds. The response includes the latest value of each feed, and other metadata about each feed.
adafruit.allFeeds({
"username": ""
}, context)
object
string
: a valid username stringarray
Create a new Feed
adafruit.createFeed({
"username": "",
"feed": {}
}, context)
object
string
: a valid username stringstring
object
string
string
string
string
Delete an existing Feed
adafruit.destroyFeed({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keyOutput schema unknown
Returns feed based on the feed key
adafruit.getFeed({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keyUpdate properties of an existing Feed
adafruit.updateFeed({
"username": "",
"feed_key": "",
"feed": {}
}, context)
object
string
: a valid username stringstring
: a valid feed keyobject
string
string
string
string
Replace an existing Feed
adafruit.replaceFeed({
"username": "",
"feed_key": "",
"feed": {}
}, context)
object
string
: a valid username stringstring
: a valid feed keyobject
string
string
string
string
Get all data for the given feed
adafruit.allData({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
: Start time for filtering, returns records created after given time.string
: End time for filtering, returns records created before give time.integer
: Limit the number of records returned.string
: List of Data record fields to include in response as comma separated list. Acceptable values are: value
, lat
, lon
, ele
, id
, and created_at
.array
Create new data records on the given feed.
NOTE: when feed history is on, data value
size is limited to 1KB, when feed history is turned off data value size is limited to 100KB.
adafruit.createData({
"username": "",
"feed_key": "",
"datum": {}
}, context)
object
string
: a valid username stringstring
: a valid feed keyobject
string
string
number
string
string
string
Create multiple new Data records
adafruit.batchCreateData({
"username": "",
"feed_key": "",
"data": []
}, context)
object
string
: a valid username stringstring
: a valid feed keyarray
object
string
string
number
string
string
string
array
The Chart API is what we use on io.adafruit.com to populate charts over varying timespans with a consistent number of data points. The maximum number of points returned is 480. This API works by aggregating slices of time into a single value by averaging.
All time-based parameters are optional, if none are given it will default to 1 hour at the finest-grained resolution possible.
adafruit.chartData({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
: Start time for filtering, returns records created after given time.string
: End time for filtering, returns records created before give time.integer
: A resolution size in minutes. By giving a resolution value you will get back grouped data points aggregated over resolution-sized intervals. NOTE: time span is preferred over resolution, so if you request a span of time that includes more than max limit points you may get a larger resolution than you requested. Valid resolutions are 1, 5, 10, 30, 60, and 120.integer
: The number of hours the chart should cover.object
array
: The names of the columns returned as data.
string
array
: The actual chart data.
array
string
object
integer
string
string
object
Get the oldest data point in the feed. This request sets the queue pointer to the beginning of the feed.
adafruit.firstData({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
: List of Data record fields to include in response as comma separated list. Acceptable values are: value
, lat
, lon
, ele
, id
, and created_at
.Get the most recent data point in the feed. This request sets the queue pointer to the end of the feed.
adafruit.lastData({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
: List of Data record fields to include in response as comma separated list. Acceptable values are: value
, lat
, lon
, ele
, id
, and created_at
.Get the next newest data point in the feed. If queue processing hasn't been started, the first data point in the feed will be returned.
adafruit.nextData({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
: List of Data record fields to include in response as comma separated list. Acceptable values are: value
, lat
, lon
, ele
, id
, and created_at
.Get the previously processed data point in the feed. NOTE: this method doesn't move the processing queue pointer.
adafruit.previousData({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
: List of Data record fields to include in response as comma separated list. Acceptable values are: value
, lat
, lon
, ele
, id
, and created_at
.Get the most recent data point in the feed in an MQTT compatible CSV format: value,lat,lon,ele
adafruit.retainData({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
Delete existing Data
adafruit.destroyData({
"username": "",
"feed_key": "",
"id": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
string
Returns data based on feed key
adafruit.getData({
"username": "",
"feed_key": "",
"id": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
string
: List of Data record fields to include in response as comma separated list. Acceptable values are: value
, lat
, lon
, ele
, id
, and created_at
.Update properties of existing Data
adafruit.updateData({
"username": "",
"feed_key": "",
"id": "",
"datum": {}
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
object
string
string
number
string
string
string
Replace existing Data
adafruit.replaceData({
"username": "",
"feed_key": "",
"id": "",
"datum": {}
}, context)
object
string
: a valid username stringstring
: a valid feed keystring
object
string
string
number
string
string
string
Returns more detailed feed record based on the feed key
adafruit.getFeedDetails({
"username": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
: a valid feed keyThe Groups endpoint returns information about the user's groups. The response includes the latest value of each feed in the group, and other metadata about the group.
adafruit.allGroups({
"username": ""
}, context)
object
string
: a valid username stringarray
Create a new Group
adafruit.createGroup({
"username": "",
"group": {}
}, context)
object
string
: a valid username stringobject
string
string
string
Delete an existing Group
adafruit.destroyGroup({
"username": "",
"group_key": ""
}, context)
object
string
: a valid username stringstring
string
Returns Group based on ID
adafruit.getGroup({
"username": "",
"group_key": ""
}, context)
object
string
: a valid username stringstring
Update properties of an existing Group
adafruit.updateGroup({
"username": "",
"group_key": "",
"group": {}
}, context)
object
string
: a valid username stringstring
object
string
string
string
Replace an existing Group
adafruit.replaceGroup({
"username": "",
"group_key": "",
"group": {}
}, context)
object
string
: a valid username stringstring
object
string
string
string
Add an existing Feed to a Group
adafruit.addFeedToGroup({
"group_key": "",
"username": ""
}, context)
object
string
string
: a valid username stringstring
Create new data for multiple feeds in a group
adafruit.createGroupData({
"username": "",
"group_key": "",
"group_feed_data": {
"feeds": []
}
}, context)
object
string
: a valid username stringstring
object
string
: Optional created_at timestamp which will be applied to all feed values created.array
: An array of feed data records with key
and value
properties.
object
string
string
object
: A location record with lat
, lon
, and [optional] ele
properties.
number
number
number
array
The Group Feeds endpoint returns information about the user's feeds. The response includes the latest value of each feed, and other metadata about each feed, but only for feeds within the given group.
adafruit.allGroupFeeds({
"group_key": "",
"username": ""
}, context)
object
string
string
: a valid username stringarray
Create a new Feed in a Group
adafruit.createGroupFeed({
"username": "",
"group_key": "",
"feed": {}
}, context)
object
string
: a valid username stringstring
object
string
string
string
string
All data for current feed in a specific group
adafruit.allGroupFeedData({
"username": "",
"group_key": "",
"feed_key": ""
}, context)
object
string
: a valid username stringstring
string
: a valid feed keystring
: Start time for filtering data. Returns data created after given time.string
: End time for filtering data. Returns data created before give time.integer
: Limit the number of records returned.array
Create new Data in a feed belonging to a particular group
adafruit.createGroupFeedData({
"username": "",
"group_key": "",
"feed_key": "",
"datum": {}
}, context)
object
string
: a valid username stringstring
string
: a valid feed keyobject
string
string
number
string
string
string
Create multiple new Data records in a feed belonging to a particular group
adafruit.batchCreateGroupFeedData({
"username": "",
"group_key": "",
"feed_key": "",
"data": []
}, context)
object
string
: a valid username stringstring
string
: a valid feed keyarray
object
string
string
number
string
string
string
array
Remove a Feed from a Group
adafruit.removeFeedFromGroup({
"group_key": "",
"username": ""
}, context)
object
string
string
: a valid username stringstring
Get the user's data rate limit and current activity level.
adafruit.getCurrentUserThrottle({
"username": ""
}, context)
object
string
: a valid username stringobject
integer
: Actions taken inside the time window.integer
: Max possible actions inside the time window (usually 1 minute).The Tokens endpoint returns information about the user's tokens.
adafruit.allTokens({
"username": ""
}, context)
object
string
: a valid username stringarray
Create a new Token
adafruit.createToken({
"username": "",
"token": {}
}, context)
object
string
: a valid username stringobject
string
Delete an existing Token
adafruit.destroyToken({
"username": "",
"id": ""
}, context)
object
string
: a valid username stringstring
string
Returns Token based on ID
adafruit.getToken({
"username": "",
"id": ""
}, context)
object
string
: a valid username stringstring
Update properties of an existing Token
adafruit.updateToken({
"username": "",
"id": "",
"token": {}
}, context)
object
string
: a valid username stringstring
object
string
Replace an existing Token
adafruit.replaceToken({
"username": "",
"id": "",
"token": {}
}, context)
object
string
: a valid username stringstring
object
string
The Triggers endpoint returns information about the user's triggers.
adafruit.allTriggers({
"username": ""
}, context)
object
string
: a valid username stringarray
Create a new Trigger
adafruit.createTrigger({
"username": "",
"trigger": {}
}, context)
object
string
: a valid username stringobject
string
Delete an existing Trigger
adafruit.destroyTrigger({
"username": "",
"id": ""
}, context)
object
string
: a valid username stringstring
string
Returns Trigger based on ID
adafruit.getTrigger({
"username": "",
"id": ""
}, context)
object
string
: a valid username stringstring
Update properties of an existing Trigger
adafruit.updateTrigger({
"username": "",
"id": "",
"trigger": {}
}, context)
object
string
: a valid username stringstring
object
string
Replace an existing Trigger
adafruit.replaceTrigger({
"username": "",
"id": "",
"trigger": {}
}, context)
object
string
: a valid username stringstring
object
string
The Permissions endpoint returns information about the user's permissions.
adafruit.allPermissions({
"username": "",
"type": "",
"type_id": ""
}, context)
object
string
: a valid username stringstring
string
array
Create a new Permission
adafruit.createPermission({
"username": "",
"type": "",
"type_id": "",
"permission": {}
}, context)
object
string
: a valid username stringstring
string
object
string
(values: r, w, rw)string
(values: secret, public, user, organization)string
Delete an existing Permission
adafruit.destroyPermission({
"username": "",
"type": "",
"type_id": "",
"id": ""
}, context)
object
string
: a valid username stringstring
string
string
string
Returns Permission based on ID
adafruit.getPermission({
"username": "",
"type": "",
"type_id": "",
"id": ""
}, context)
object
string
: a valid username stringstring
string
string
Update properties of an existing Permission
adafruit.updatePermission({
"username": "",
"type": "",
"type_id": "",
"id": "",
"permission": {}
}, context)
object
string
: a valid username stringstring
string
string
object
string
(values: r, w, rw)string
(values: secret, public, user, organization)string
Replace an existing Permission
adafruit.replacePermission({
"username": "",
"type": "",
"type_id": "",
"id": "",
"permission": {}
}, context)
object
string
: a valid username stringstring
string
string
object
string
(values: r, w, rw)string
(values: secret, public, user, organization)string
object
string
string
object
number
string
string
number
object
array
number
string
string
string
number
number
number
string
object
array
string
string
string
object
string
string
number
number
string
number
number
string
number
number
string
string
object
string
string
number
number
string
number
number
string
number
number
string
string
object
string
string
object
string
string
object
: Additional details about this feed.
object
integer
: Number of data points stored by this feed.object
object
array
: Access control list for this feed
object
boolean
object
array
boolean
number
string
string
string
string
string
boolean
: Is status notification active?integer
: Status notification timeout in minutes.string
string
string
string
(values: private, public)object
string
string
array
number
string
string
object
string
number
string
(values: feed, group, dashboard)number
string
(values: secret, public, user, organization)string
string
number
object
string
string
number
string
string
object
string
object
string
object
string
string
number
string
string
string
string
FAQs
DataFire integration for Adafruit IO REST API
We found that @datafire/adafruit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.