@dcasia/mini-program-tailwind-webpack-plugin
Advanced tools
Comparing version 1.2.3 to 1.3.0-beta.0
'use strict'; | ||
var universalHandler = require('./universal-handler'); | ||
var webpackSources = require('webpack-sources'); | ||
@@ -36,9 +37,17 @@ /*! ***************************************************************************** | ||
})(FileType || (FileType = {})); | ||
var FrameworkUsedInTaro; | ||
(function (FrameworkUsedInTaro) { | ||
FrameworkUsedInTaro["React"] = "react"; | ||
FrameworkUsedInTaro["Vue2"] = "vue2"; | ||
FrameworkUsedInTaro["Vue3"] = "vue3"; | ||
})(FrameworkUsedInTaro || (FrameworkUsedInTaro = {})); | ||
var _a; | ||
var regExpStyle = /.+\.(?:wx|ac|jx|tt|q|c)ss$/; | ||
var regExpTemplate = /.+\.(wx|ax|jx|ks|tt|q)ml$/; | ||
function isStyleFile(filename) { | ||
return /.+\.(?:wx|ac|jx|tt|q|c)ss$/.test(filename); | ||
return regExpStyle.test(filename); | ||
} | ||
function isTemplateFile(filename) { | ||
return /.+\.(wx|ax|jx|ks|tt|q)ml$/.test(filename); | ||
return regExpTemplate.test(filename); | ||
} | ||
@@ -60,27 +69,52 @@ (_a = {}, | ||
var _this = this; | ||
var webpack = compiler.webpack; | ||
var sources = webpack.sources, Compilation = webpack.Compilation; | ||
var ConcatSource = sources.ConcatSource; | ||
compiler.hooks.thisCompilation.tap(MiniProgramTailwindWebpackPlugin.pluginName, function (compilation) { | ||
compilation.hooks.processAssets.tap({ | ||
name: MiniProgramTailwindWebpackPlugin.pluginName, | ||
stage: Compilation.PROCESS_ASSETS_STAGE_SUMMARIZE | ||
}, function (assets) { | ||
for (var pathname in assets) { | ||
var originalSource = assets[pathname]; | ||
var rawSource = originalSource.source().toString(); | ||
var handledSource = ''; | ||
if (isStyleFile(pathname)) { | ||
handledSource = universalHandler.handleSource(FileType.Style, rawSource, _this.options); | ||
var isWebpackV5 = compiler.webpack && compiler.webpack.version >= '5'; | ||
if (isWebpackV5) { | ||
var webpack = compiler.webpack; | ||
var sources = webpack.sources, Compilation_1 = webpack.Compilation; | ||
var ConcatSource_1 = sources.ConcatSource; | ||
compiler.hooks.thisCompilation.tap(MiniProgramTailwindWebpackPlugin.pluginName, function (compilation) { | ||
compilation.hooks.processAssets.tap({ | ||
name: MiniProgramTailwindWebpackPlugin.pluginName, | ||
stage: Compilation_1.PROCESS_ASSETS_STAGE_SUMMARIZE | ||
}, function (assets) { | ||
for (var pathname in assets) { | ||
var originalSource = assets[pathname]; | ||
var rawSource = originalSource.source().toString(); | ||
var handledSource = ''; | ||
if (isStyleFile(pathname)) { | ||
handledSource = universalHandler.handleSource(FileType.Style, rawSource, _this.options); | ||
} | ||
else if (isTemplateFile(pathname)) { | ||
handledSource = universalHandler.handleSource(FileType.Template, rawSource, _this.options); | ||
} | ||
if (handledSource) { | ||
var source = new ConcatSource_1(handledSource); | ||
compilation.updateAsset(pathname, source); | ||
} | ||
} | ||
else if (isTemplateFile(pathname)) { | ||
handledSource = universalHandler.handleSource(FileType.Template, rawSource, _this.options); | ||
}); | ||
}); | ||
} | ||
else { | ||
compiler.hooks.thisCompilation.tap(MiniProgramTailwindWebpackPlugin.pluginName, function (compilation) { | ||
compilation.hooks.afterOptimizeAssets.tap(MiniProgramTailwindWebpackPlugin.pluginName, function (assets) { | ||
for (var pathname in assets) { | ||
var originalSource = assets[pathname]; | ||
var rawSource = originalSource.source().toString(); | ||
var handledSource = ''; | ||
if (isStyleFile(pathname)) { | ||
handledSource = universalHandler.handleSource(FileType.Style, rawSource, _this.options); | ||
} | ||
else if (isTemplateFile(pathname)) { | ||
handledSource = universalHandler.handleSource(FileType.Template, rawSource, _this.options); | ||
} | ||
if (handledSource) { | ||
var source = new webpackSources.ConcatSource(handledSource); | ||
// @ts-ignore | ||
compilation.updateAsset(pathname, source); | ||
} | ||
} | ||
if (handledSource) { | ||
var source = new ConcatSource(handledSource); | ||
compilation.updateAsset(pathname, source); | ||
} | ||
} | ||
}); | ||
}); | ||
}); | ||
} | ||
}; | ||
@@ -87,0 +121,0 @@ MiniProgramTailwindWebpackPlugin.pluginName = 'mini-program-tailwind-webpack-plugin'; |
@@ -7,2 +7,3 @@ 'use strict'; | ||
var wxml = require('@vivaxy/wxml'); | ||
var babel = require('@babel/core'); | ||
@@ -31,2 +32,3 @@ function _interopDefaultLegacy (e) { return e && typeof e === 'object' && 'default' in e ? e : { 'default': e }; } | ||
var wxml__namespace = /*#__PURE__*/_interopNamespace(wxml); | ||
var babel__namespace = /*#__PURE__*/_interopNamespace(babel); | ||
@@ -38,4 +40,10 @@ var FileType; | ||
})(FileType || (FileType = {})); | ||
var FrameworkUsedInTaro; | ||
(function (FrameworkUsedInTaro) { | ||
FrameworkUsedInTaro["React"] = "react"; | ||
FrameworkUsedInTaro["Vue2"] = "vue2"; | ||
FrameworkUsedInTaro["Vue3"] = "vue3"; | ||
})(FrameworkUsedInTaro || (FrameworkUsedInTaro = {})); | ||
var _a; | ||
var _a$1; | ||
var charactersMap = { | ||
@@ -56,6 +64,6 @@ '[': '-', | ||
}; | ||
var backslashMap = (_a = {}, | ||
_a[FileType.Style] = '\\\\\\', | ||
_a[FileType.Template] = '\\', | ||
_a); | ||
var backslashMap = (_a$1 = {}, | ||
_a$1[FileType.Style] = '\\\\\\', | ||
_a$1[FileType.Template] = '\\', | ||
_a$1); | ||
function handleCharacters(content, type) { | ||
@@ -157,2 +165,8 @@ for (var from in charactersMap) { | ||
var _a; | ||
(_a = {}, | ||
_a[FrameworkUsedInTaro.React] = ['className'], | ||
_a[FrameworkUsedInTaro.Vue2] = ['class', 'staticClass'], | ||
_a[FrameworkUsedInTaro.Vue3] = ['class', 'staticClass'], | ||
_a); | ||
function replaceStringLiteralPlugin(_a) { | ||
@@ -163,5 +177,8 @@ var t = _a.types; | ||
StringLiteral: function (path) { | ||
var newContent = handleCharacters(path.node.value, FileType.Template); | ||
path.replaceWith(t.stringLiteral(newContent)); | ||
path.skip(); | ||
var rawContent = path.node.value; | ||
var newContent = handleCharacters(rawContent, FileType.Template); | ||
if (newContent !== rawContent) { | ||
path.replaceWith(t.stringLiteral(newContent)); | ||
path.skip(); | ||
} | ||
} | ||
@@ -172,3 +189,2 @@ } | ||
var babel = require('@babel/core'); | ||
var matchScriptsInsideClassNames = /{{.+?}}/g; | ||
@@ -190,6 +206,7 @@ var replaceMarker = '__MP_TW_PLUGIN_REPLACE__'; | ||
var script = scriptsMatchResults_1[_i]; | ||
var output = babel.transformSync(script[0], { | ||
var output = babel__namespace.transformSync(script[0], { | ||
generatorOpts: { | ||
minified: true | ||
}, | ||
configFile: false, | ||
plugins: [replaceStringLiteralPlugin] | ||
@@ -215,3 +232,8 @@ }); | ||
} | ||
var universalHandler = { | ||
handleStyle: handleStyle, | ||
handleTemplate: handleTemplate | ||
}; | ||
exports["default"] = universalHandler; | ||
exports.handleSource = handleSource; |
{ | ||
"name": "@dcasia/mini-program-tailwind-webpack-plugin", | ||
"version": "1.2.3", | ||
"version": "1.3.0-beta.0", | ||
"description": "", | ||
@@ -10,3 +10,3 @@ "main": "dist/index.js", | ||
"scripts": { | ||
"test": "echo \"Error: no test specified\" && exit 1", | ||
"test": "jest", | ||
"lint": "eslint . --ext .ts --fix", | ||
@@ -28,3 +28,6 @@ "build": "rollup --config rollup.config.ts", | ||
"@rollup/plugin-commonjs": "^21.0.2", | ||
"@rollup/plugin-multi-entry": "^4.1.0", | ||
"@rollup/plugin-typescript": "^8.3.1", | ||
"@tarojs/service": "^3.4.9", | ||
"@types/jest": "^27.4.1", | ||
"@typescript-eslint/eslint-plugin": "^5.13.0", | ||
@@ -36,3 +39,5 @@ "@typescript-eslint/parser": "^5.13.0", | ||
"eslint-plugin-import": "^2.25.4", | ||
"rollup": "^2.68.0" | ||
"jest": "^27.5.1", | ||
"rollup": "^2.68.0", | ||
"webpack": "^5.69.1" | ||
}, | ||
@@ -43,4 +48,5 @@ "dependencies": { | ||
"postcss": "^8.4.7", | ||
"webpack": "^5.69.1" | ||
"windicss-webpack-plugin": "^1.7.2", | ||
"webpack-sources": "^1.4.3" | ||
} | ||
} |
@@ -26,9 +26,9 @@ 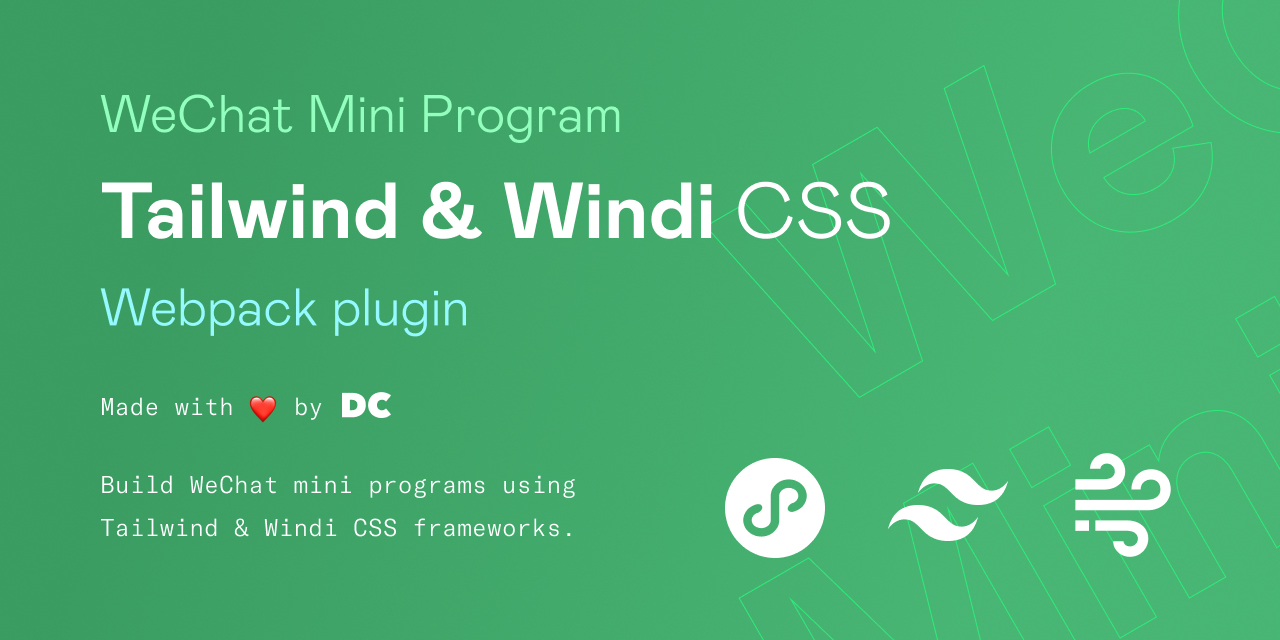 | ||
相关文章:[让你的小程序用上原汁原味的 Tailwind/Windi CSS](https://juejin.cn/post/7093809282272985119/) | ||
- - - | ||
## 快速开始 | ||
### 基于原生小程序 | ||
**以下示范操作步骤以集成 Windi CSS 为例* | ||
待更新... | ||
### 基于 MPX 框架 | ||
@@ -48,2 +48,3 @@ | ||
//... | ||
prefixer: false, | ||
extract: { | ||
@@ -54,2 +55,6 @@ // 将 .mpx 文件纳入范围 | ||
exclude: ['node_modules', '.git', 'dist'] | ||
}, | ||
corePlugins: { | ||
// 禁用掉在小程序环境中不可能用到的 plugins | ||
container: false | ||
} | ||
@@ -94,4 +99,37 @@ //... | ||
[示例项目](./examples/mpx) | ||
#### 案例 | ||
[MPX 集成案例](./examples/mpx) | ||
### 基于原生小程序 | ||
基于原生小程序的开发模式来集成这款插件,过程通常因每个团队的工作流不同而异。有的团队会有内部定制的一套 Webpack 或 Gulp 工作流,而有的团队甚至不会借助任何文件打包或处理的工作流去编写小程序。 | ||
但这里需要明确的一点是,若要想在以原生开发模式的基础之上去增加文件处理的功能,我们必须去额外的启动一套文件监听处理服务,这个服务通常由自定义配置好的 Webpack, Gulp 等第三方工具完成。 | ||
为了使这款插件具备超出 Webpack 适配范围之外尽可能大的兼容性,我们将核心功能分离并打包进了 `dist/universal-handler.js` 文件中,若你想在自己的工作流中使用该插件的核心功能,可以先在工作流逻辑中引入 `universal-handler`: | ||
```javascript | ||
const { handleSource } = require('@dcasia/mini-program-tailwind-webpack-plugin/dist/universal-handler') | ||
``` | ||
随后处理 template: | ||
```javascript | ||
const template = '<view class="w-10 h-[0.5px] text-[#ffffff]"></view>' | ||
const handledTemplate = handleSource('template', template, options) | ||
``` | ||
处理 style: | ||
```javascript | ||
const style = '.h-\\[0\\.5px\\] {height: 0.5px;}' | ||
const handledStyle = handleSource('style', style, options) | ||
``` | ||
此后你便可以将处理过的字符串返回至工作流原本的流程中来生成最终的文件。 | ||
> 对于集成过程中涉及到其他方面的细节可参考[小程序集成 Windi CSS 的自定义实现](https://juejin.cn/post/7093809282272985119#heading-5) | ||
#### 案例 | ||
[原生小程序集成案例(基于 Gulp)](./examples/native) | ||
- - - | ||
@@ -106,2 +144,15 @@ ## 可配置参数 | ||
- - - | ||
## 陷阱 | ||
- 在小程序中为了使组件样式可以被 Tailwind/Windi 的 CSS 产物作用到,我们需要在每一个组件的 JSON 配置文件中设置其[样式的作用域](https://developers.weixin.qq.com/miniprogram/dev/framework/custom-component/wxml-wxss.html#%E7%BB%84%E4%BB%B6%E6%A0%B7%E5%BC%8F%E9%9A%94%E7%A6%BB) `styleIsolation`,否则即使 Tailwind/Windi CSS 工作正常也无法用来开发组件 UI。([Issue#1](https://github.com/dcasia/wechat-mini-program-tailwind/issues/1)) | ||
```json | ||
{ | ||
"component": true, | ||
"styleIsolation": "apply-shared" | ||
} | ||
``` | ||
- 由于目前微信开发者工具的热重载功能无法监听到样式文件内由 `@import` 导入的 wxss 文件内容的变动,所以当启用热重载功能开发时,模拟器不会随着你对 Tailwind/Windi CSS 的更改而更新 UI。目前微信官方已知晓该 bug 的存在,在该 bug 修复之前,我们建议你在开发时关闭热重载,用传统的页面自动刷新来预览每一次的 UI 更新。([Issue#3](https://github.com/dcasia/wechat-mini-program-tailwind/issues/3)) | ||
- - - | ||
## FAQ | ||
@@ -108,0 +159,0 @@ |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No tests
QualityPackage does not have any tests. This is a strong signal of a poorly maintained or low quality package.
Found 1 instance in 1 package
44238
5
815
189
5
14
+ Addedwebpack-sources@^1.4.3
+ Added@antfu/utils@0.7.10(transitive)
+ Added@nodelib/fs.scandir@2.1.5(transitive)
+ Added@nodelib/fs.stat@2.0.5(transitive)
+ Added@nodelib/fs.walk@1.2.8(transitive)
+ Added@windicss/config@1.9.3(transitive)
+ Added@windicss/plugin-utils@1.9.3(transitive)
+ Addedbig.js@5.2.2(transitive)
+ Addedbraces@3.0.3(transitive)
+ Addedemojis-list@3.0.0(transitive)
+ Addedfast-glob@3.3.2(transitive)
+ Addedfastq@1.17.1(transitive)
+ Addedfill-range@7.1.1(transitive)
+ Addedget-port@6.1.2(transitive)
+ Addedglob-parent@5.1.2(transitive)
+ Addedis-extglob@2.1.1(transitive)
+ Addedis-glob@4.0.3(transitive)
+ Addedis-number@7.0.0(transitive)
+ Addedjiti@1.21.6(transitive)
+ Addedloader-utils@2.0.4(transitive)
+ Addedlodash@4.17.21(transitive)
+ Addedmagic-string@0.30.13(transitive)
+ Addedmerge2@1.4.1(transitive)
+ Addedmicromatch@4.0.8(transitive)
+ Addedpathe@1.1.2(transitive)
+ Addedpicomatch@2.3.1(transitive)
+ Addedqueue-microtask@1.2.3(transitive)
+ Addedreusify@1.0.4(transitive)
+ Addedrun-parallel@1.2.0(transitive)
+ Addedsource-list-map@2.0.1(transitive)
+ Addedto-regex-range@5.0.1(transitive)
+ Addedwebpack-sources@1.4.3(transitive)
+ Addedwebpack-virtual-modules@0.5.0(transitive)
+ Addedwindicss@3.5.6(transitive)
+ Addedwindicss-webpack-plugin@1.8.0(transitive)
- Removedwebpack@^5.69.1
- Removed@jridgewell/source-map@0.3.6(transitive)
- Removed@types/eslint@9.6.1(transitive)
- Removed@types/eslint-scope@3.7.7(transitive)
- Removed@types/estree@1.0.6(transitive)
- Removed@types/json-schema@7.0.15(transitive)
- Removed@types/node@22.9.1(transitive)
- Removed@webassemblyjs/ast@1.14.1(transitive)
- Removed@webassemblyjs/floating-point-hex-parser@1.13.2(transitive)
- Removed@webassemblyjs/helper-api-error@1.13.2(transitive)
- Removed@webassemblyjs/helper-buffer@1.14.1(transitive)
- Removed@webassemblyjs/helper-numbers@1.13.2(transitive)
- Removed@webassemblyjs/helper-wasm-bytecode@1.13.2(transitive)
- Removed@webassemblyjs/helper-wasm-section@1.14.1(transitive)
- Removed@webassemblyjs/ieee754@1.13.2(transitive)
- Removed@webassemblyjs/leb128@1.13.2(transitive)
- Removed@webassemblyjs/utf8@1.13.2(transitive)
- Removed@webassemblyjs/wasm-edit@1.14.1(transitive)
- Removed@webassemblyjs/wasm-gen@1.14.1(transitive)
- Removed@webassemblyjs/wasm-opt@1.14.1(transitive)
- Removed@webassemblyjs/wasm-parser@1.14.1(transitive)
- Removed@webassemblyjs/wast-printer@1.14.1(transitive)
- Removed@xtuc/ieee754@1.2.0(transitive)
- Removed@xtuc/long@4.2.2(transitive)
- Removedacorn@8.14.0(transitive)
- Removedajv@6.12.6(transitive)
- Removedajv-keywords@3.5.2(transitive)
- Removedbuffer-from@1.1.2(transitive)
- Removedchrome-trace-event@1.0.4(transitive)
- Removedcommander@2.20.3(transitive)
- Removedenhanced-resolve@5.17.1(transitive)
- Removedes-module-lexer@1.5.4(transitive)
- Removedeslint-scope@5.1.1(transitive)
- Removedesrecurse@4.3.0(transitive)
- Removedestraverse@4.3.05.3.0(transitive)
- Removedevents@3.3.0(transitive)
- Removedfast-deep-equal@3.1.3(transitive)
- Removedfast-json-stable-stringify@2.1.0(transitive)
- Removedglob-to-regexp@0.4.1(transitive)
- Removedgraceful-fs@4.2.11(transitive)
- Removedhas-flag@4.0.0(transitive)
- Removedjest-worker@27.5.1(transitive)
- Removedjson-parse-even-better-errors@2.3.1(transitive)
- Removedjson-schema-traverse@0.4.1(transitive)
- Removedloader-runner@4.3.0(transitive)
- Removedmerge-stream@2.0.0(transitive)
- Removedmime-db@1.52.0(transitive)
- Removedmime-types@2.1.35(transitive)
- Removedneo-async@2.6.2(transitive)
- Removedpunycode@2.3.1(transitive)
- Removedrandombytes@2.1.0(transitive)
- Removedsafe-buffer@5.2.1(transitive)
- Removedschema-utils@3.3.0(transitive)
- Removedserialize-javascript@6.0.2(transitive)
- Removedsource-map-support@0.5.21(transitive)
- Removedsupports-color@8.1.1(transitive)
- Removedtapable@2.2.1(transitive)
- Removedterser@5.36.0(transitive)
- Removedterser-webpack-plugin@5.3.10(transitive)
- Removedundici-types@6.19.8(transitive)
- Removeduri-js@4.4.1(transitive)
- Removedwatchpack@2.4.2(transitive)
- Removedwebpack@5.96.1(transitive)
- Removedwebpack-sources@3.2.3(transitive)