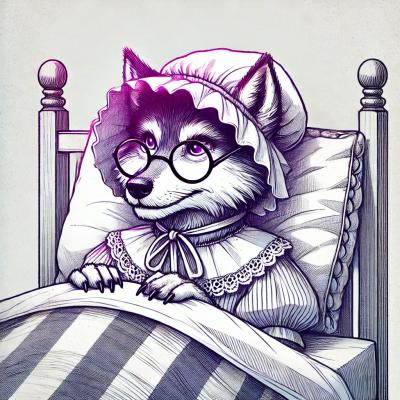
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@dotcms/client
Advanced tools
Official JavaScript library for interacting with DotCMS REST APIs.
@dotcms/client
is the official dotCMS JavaScript library designed to simplify interactions with the DotCMS REST APIs.
This client library provides a streamlined, promise-based interface to fetch pages and navigation API.
Install the package via npm:
npm install @dotcms/client
Or using Yarn:
yarn add @dotcms/client
@dotcms/client
supports both ES modules and CommonJS. You can import it using either syntax:
import { DotCmsClient } from '@dotcms/client';
const { DotCmsClient } = require('@dotcms/client');
First, initialize the client with your DotCMS instance details.
const client = DotCmsClient.init({
dotcmsUrl: 'https://your-dotcms-instance.com',
authToken: 'your-auth-token',
siteId: 'your-site-id'
});
Retrieve the elements of any page in your DotCMS system in JSON format.
const pageData = await client.page.get({
path: '/your-page-path',
language_id: 1,
personaId: 'optional-persona-id'
});
console.log(pageData);
Retrieve information about the DotCMS file and folder tree.
const navData = await client.nav.get({
path: '/',
depth: 2,
languageId: 1
});
console.log(navData);
Detailed documentation of the @dotcms/client
methods, parameters, and types can be found below:
DotCmsClient.init(config: ClientConfig): DotCmsClient
Initializes the DotCMS client with the specified configuration.
DotCmsClient.page.get(options: PageApiOptions): Promise<unknown>
Retrieves the specified page's elements from your DotCMS system in JSON format.
DotCmsClient.nav.get(options: NavApiOptions): Promise<unknown>
Retrieves information about the DotCMS file and folder tree.
GitHub pull requests are the preferred method to contribute code to dotCMS. Before any pull requests can be accepted, an automated tool will ask you to agree to the dotCMS Contributor's Agreement.
dotCMS comes in multiple editions and as such is dual licensed. The dotCMS Community Edition is licensed under the GPL 3.0 and is freely available for download, customization and deployment for use within organizations of all stripes. dotCMS Enterprise Editions (EE) adds a number of enterprise features and is available via a supported, indemnified commercial license from dotCMS. For the differences between the editions, see the feature page.
If you need help or have any questions, please open an issue in the GitHub repository.
Always refer to the official DotCMS documentation for comprehensive guides and API references.
Source | Location |
---|---|
Installation | Installation |
Documentation | Documentation |
Videos | Helpful Videos |
Forums/Listserv | via Google Groups |
@dotCMS | |
Main Site | dotCMS.com |
FAQs
Official JavaScript library for interacting with DotCMS REST APIs.
The npm package @dotcms/client receives a total of 18 weekly downloads. As such, @dotcms/client popularity was classified as not popular.
We found that @dotcms/client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.