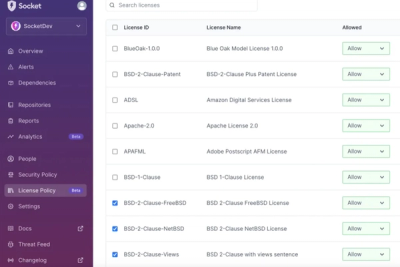
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
@edgeros/minio-client
Advanced tools
JSRE
and Node.js
The MinIO JavaScript Client SDK provides high level APIs to access any Amazon S3 compatible object storage server.
This guide will show you how to install the client SDK and execute an example JavaScript program. For a complete list of APIs and examples, please take a look at the JavaScript Client API Reference documentation.
npm install --save @edgeros/minio
The following parameters are needed to connect to a MinIO object storage server:
Parameter | Description |
---|---|
endPoint | Hostname of the object storage service. |
port | TCP/IP port number. Optional, defaults to 80 for HTTP and 443 for HTTPs. |
accessKey | Access key (user ID) of an account in the S3 service. |
secretKey | Secret key (password) of an account in the S3 service. |
useSSL | Optional, set to 'true' to enable secure (HTTPS) access. |
const Minio = require('@edgeros/minio')
const minioClient = new Minio.Client({
endPoint: 'play.min.io',
port: 9000,
useSSL: true,
accessKey: 'Q3AM3UQ867SPQQA43P2F',
secretKey: 'zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG',
})
This example connects to an object storage server, creates a bucket, and uploads a file to the bucket.
It uses the MinIO play
server, a public MinIO cluster located at https://play.min.io.
The play
server runs the latest stable version of MinIO and may be used for testing and development.
The access credentials shown in this example are open to the public.
All data uploaded to play
should be considered public and non-protected.
const Minio = require('@edgeros/minio')
// Instantiate the MinIO client with the object store service
// endpoint and an authorized user's credentials
// play.min.io is the MinIO public test cluster
const minioClient = new Minio.Client({
endPoint: 'play.min.io',
port: 9000,
useSSL: true,
accessKey: 'Q3AM3UQ867SPQQA43P2F',
secretKey: 'zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG',
})
// File to upload
const sourceFile = '/tmp/test-file.txt'
// Destination bucket
const bucket = 'js-test-bucket'
// Destination object name
const destinationObject = 'my-test-file.txt'
// Check if the bucket exists
// If it doesn't, create it
const exists = await minioClient.bucketExists(bucket)
if (exists) {
console.log('Bucket ' + bucket + ' exists.')
} else {
await minioClient.makeBucket(bucket, 'us-east-1')
console.log('Bucket ' + bucket + ' created in "us-east-1".')
}
// Set the object metadata
var metaData = {
'Content-Type': 'text/plain',
'X-Amz-Meta-Testing': 1234,
example: 5678,
}
// Upload the file with fPutObject
// If an object with the same name exists,
// it is updated with new data
await minioClient.fPutObject(bucket, destinationObject, sourceFile, metaData)
console.log('File ' + sourceFile + ' uploaded as object ' + destinationObject + ' in bucket ' + bucket)
The complete API Reference is available here:
makeBucket
listBuckets
bucketExists
removeBucket
listObjects
listObjectsV2
listObjectsV2WithMetadata
(Extension) [Only Node.js]listIncompleteUploads
getBucketVersioning
setBucketVersioning
setBucketLifecycle
getBucketLifecycle
removeBucketLifecycle
getObjectLockConfig
setObjectLockConfig
getObject
putObject
copyObject
statObject
removeObject
removeObjects
removeIncompleteUpload
selectObjectContent
getBucketNotification
setBucketNotification
removeAllBucketNotification
listenBucketNotification
(MinIO Extension)FAQs
MinIO client support JSRE and Node
The npm package @edgeros/minio-client receives a total of 0 weekly downloads. As such, @edgeros/minio-client popularity was classified as not popular.
We found that @edgeros/minio-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.